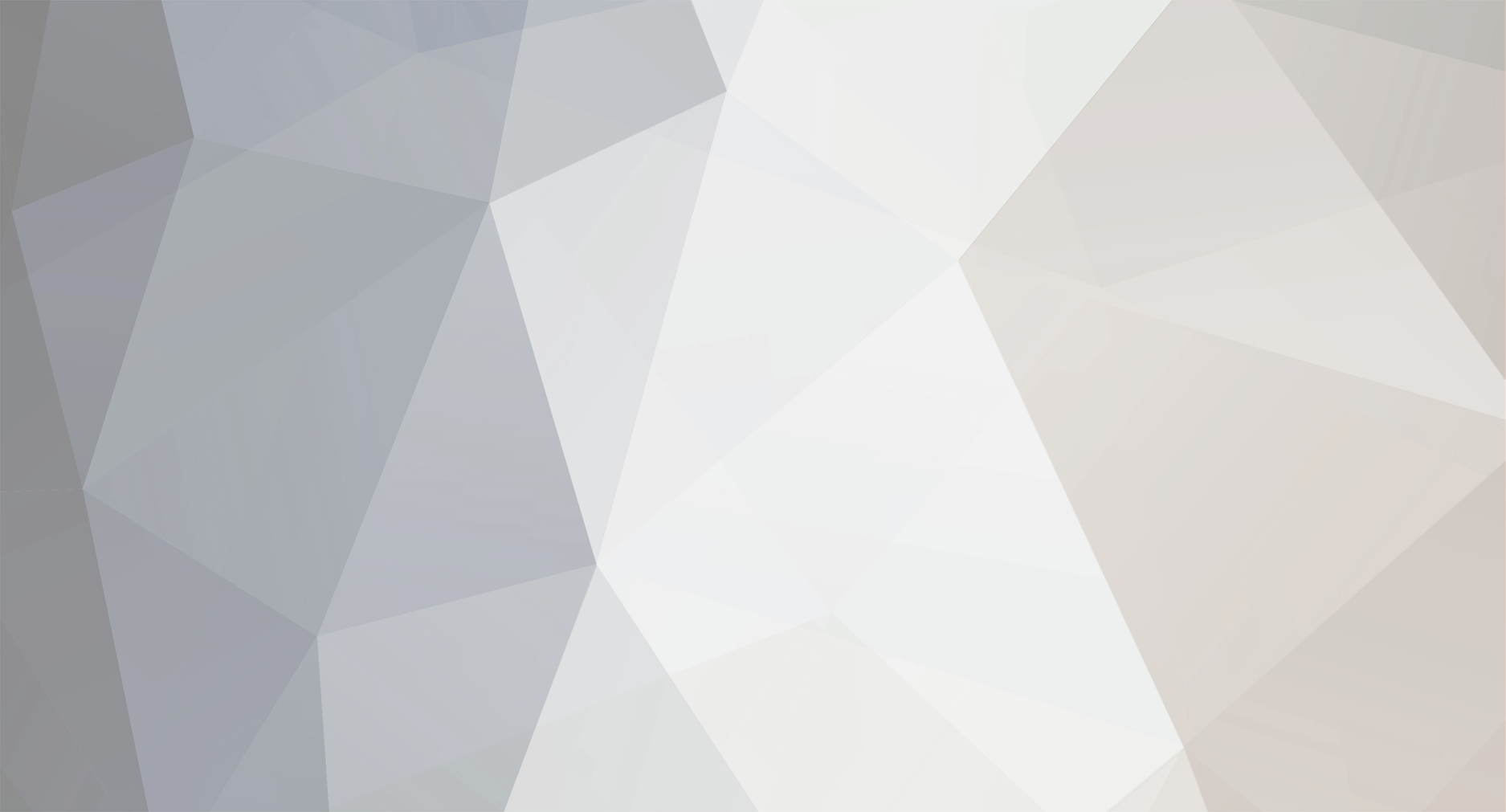
Sleepwalker
-
Posts
19 -
Joined
-
Last visited
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Posts posted by Sleepwalker
-
-
I'll remove all the lua parts and see how it performs then, thanks for the input guys
-
I feel like I'm writing my rotations the completely wrong way, they feel super clunky and I think it's mainly because I'm just writing lines that are unnecessary.
I'd like to use Explosive shot and Multi-Shot as priority, arcane shot as 1'st filler and Steady shot as a last resort if everything else is on cooldown but what I'm finding is that even if the spells are off CD it'll still use steady shot even though I've outlined it to only use the spell if the others are unusable.
There has to be a much simpler way of me doing this like a check if on CD or whatever.
I'd really appreciate some help with this since it effects a couple of the rotations I've written but this one it effects the most as explosive shot and multi-shot are my main sources of damage.
using System; using System.Collections.Generic; using System.Threading; using robotManager.Helpful; using robotManager.Products; using wManager.Wow.Class; using wManager.Wow.Enums; using wManager.Wow.Helpers; using wManager.Wow.ObjectManager; public class Main : ICustomClass { public string name = "dRotation (Multishot Hunter)"; public float Range { get { return 5.0f; } } private bool _isRunning; /* * Initialize() * When product started, initialize and launch Fightclass */ public void Initialize() { _isRunning = true; Logging.Write(name + " Is initialized."); CreateStatusFrame(); Rotation(); } /* * Dispose() * When product stopped */ public void Dispose() { _isRunning = false; Logging.Write(name + " Stop in progress."); Lua.LuaDoString(@"dRotationFrame.text:SetText(""dRotation Stopped!"")"); } /* * ShowConfiguration() * When use click on Fightclass settings */ public void ShowConfiguration() { Logging.Write(name + " No settings for this Fightclass."); } /* * Spells for Rotation */ public Spell ExplosiveShot = new Spell("Explosive Shot"); public Spell MultiShot = new Spell("Multi-Shot"); public Spell ArcaneShot = new Spell("Arcane Shot"); public Spell SliceAndDice = new Spell("Slice and Dice"); public Spell BlackArrow = new Spell("Black Arrow"); public Spell SteadyShot = new Spell("Steady Shot"); /* Rotation() */ public void Rotation() { Logging.Write(name + ": Started."); while (_isRunning) { try { if (Products.InPause) { Lua.LuaDoString(@"dRotationFrame.text:SetText(""dRotation Paused!"")"); } if (!Products.InPause) { if (!ObjectManager.Me.IsDeadMe) { if (!ObjectManager.Me.InCombatFlagOnly) { Lua.LuaDoString(@"dRotationFrame.text:SetText(""dRotation Active!"")"); } else if (ObjectManager.Me.InCombatFlagOnly && ObjectManager.Me.Target > 0) { CombatRotation(); } } } } catch (Exception e) { Logging.WriteError(name + " ERROR: " + e); } Thread.Sleep(10); // Pause 10 ms to reduce the CPU usage. } Logging.Write(name + ": Stopped."); } /* * CreateStatusFrame() * InGame Status frame to see which spells casting next */ public void CreateStatusFrame() { Lua.LuaDoString(@" if not dRotationFrame then dRotationFrame = CreateFrame(""Frame"") dRotationFrame:ClearAllPoints() dRotationFrame:SetBackdrop(StaticPopup1:GetBackdrop()) dRotationFrame:SetHeight(70) dRotationFrame:SetWidth(210) dRotationFrame.text = dRotationFrame:CreateFontString(nil, ""BACKGROUND"", ""GameFontNormal"") dRotationFrame.text:SetAllPoints() dRotationFrame.text:SetText(""dRotation by Dreamful, Ready!"") dRotationFrame.text:SetTextColor(1, 1, 1, 6) dRotationFrame:SetPoint(""CENTER"", 0, -240) dRotationFrame:SetBackdropBorderColor(0, 0, 0, 0) dRotationFrame:SetMovable(true) dRotationFrame:EnableMouse(true) dRotationFrame:SetScript(""OnMouseDown"",function() dRotationFrame:StartMoving() end) dRotationFrame:SetScript(""OnMouseUp"",function() dRotationFrame:StopMovingOrSizing() end) dRotationFrame.Close = CreateFrame(""BUTTON"", nil, dRotationFrame, ""UIPanelCloseButton"") dRotationFrame.Close:SetWidth(15) dRotationFrame.Close:SetHeight(15) dRotationFrame.Close:SetPoint(""TOPRIGHT"", dRotationFrame, -8, -8) dRotationFrame.Close:SetScript(""OnClick"", function() dRotationFrame:Hide() DEFAULT_CHAT_FRAME:AddMessage(""dRotationStatusFrame |cffC41F3Bclosed |cffFFFFFFWrite /dRotation to enable again."") end) SLASH_WHATEVERYOURFRAMESARECALLED1=""/dRotation"" SlashCmdList.WHATEVERYOURFRAMESARECALLED = function() if dRotationFrame:IsShown() then dRotationFrame:Hide() else dRotationFrame:Show() end end end"); } /* * CombatRotation() */ public void CombatRotation() { // Slice and Dice if (SliceAndDice.KnownSpell && SliceAndDice.IsSpellUsable && SliceAndDice.IsDistanceGood && !ObjectManager.Me.HaveBuff(6774) && ObjectManager.Me.ComboPoint >= 5) { SliceAndDice.Launch(); Lua.LuaDoString(@"dRotationFrame.text:SetText(""Casting Slice and Dice"")"); return; } // BlackArrow if (BlackArrow.KnownSpell && BlackArrow.IsSpellUsable && BlackArrow.IsDistanceGood) { BlackArrow.Launch(); Lua.LuaDoString(@"dRotationFrame.text:SetText(""Casting Slice and Dice"")"); return; } // ExplosiveShot if (ExplosiveShot.KnownSpell && ExplosiveShot.IsSpellUsable && ExplosiveShot.IsDistanceGood && ObjectManager.Me.ComboPoint <= 4) { ExplosiveShot.Launch(); Lua.LuaDoString(@"dRotationFrame.text:SetText(""Casting ExplosiveShot"")"); return; } // MultiShot if (MultiShot.KnownSpell && MultiShot.IsSpellUsable && !ExplosiveShot.IsSpellUsable && MultiShot.IsDistanceGood && ObjectManager.Me.ComboPoint <= 4) { MultiShot.Launch(); Lua.LuaDoString(@"dRotationFrame.text:SetText(""Casting MultiShot"")"); return; } // ArcaneShot if (ArcaneShot.KnownSpell && ArcaneShot.IsSpellUsable && !MultiShot.IsSpellUsable && !ExplosiveShot.IsSpellUsable && ArcaneShot.IsDistanceGood && ObjectManager.Me.ComboPoint <= 4) { ArcaneShot.Launch(); Lua.LuaDoString(@"dRotationFrame.text:SetText(""Casting ArcaneShot"")"); return; } // SteadyShot if (SteadyShot.KnownSpell && SteadyShot.IsSpellUsable && !ArcaneShot.IsSpellUsable && !MultiShot.IsSpellUsable && !ExplosiveShot.IsSpellUsable && SteadyShot.IsDistanceGood && ObjectManager.Me.ComboPoint <= 4) { SteadyShot.Launch(); Lua.LuaDoString(@"dRotationFrame.text:SetText(""Casting SteadyShot"")"); return; } // CompoundShot if (ObjectManager.Me.ComboPoint >= 5 && ObjectManager.Me.HaveBuff(6774)) { wManager.Wow.Helpers.SpellManager.CastSpellByIdLUA(826679); Lua.LuaDoString(@"dRotationFrame.text:SetText(""Casting CompoundShot"")"); return; } } }
-
5 hours ago, Droidz said:
Hello, when you use flying mount?
yes that's correct, doesn't matter which zone I'm flying round the bot stutters every time it sees a node while flying
Edit: I decided to try a few things and started using "WRobot (No Lock Frame)" exe and the issue is now resolved
-
Simple as the title really, the bot stutters and lags hard when it spots a node. It's fine when it uses the path I've created but once I see a node it stutters a lot.
-
On 2/15/2021 at 10:33 AM, iMod said:
UnitExtension:
/// <summary> /// Gets the units around our unit in the given range. /// </summary> /// <param name="range">The range we are looking in.</param> /// <param name="objectType">The object type we are looking for.</param> /// <returns>Returns a list of units if we found one, otherwise a empty list.</returns> public static IEnumerable<WoWUnit> GetAttackableUnits(this WoWUnit instance, int range, WoWObjectType objectType = WoWObjectType.Unit) { // Get units IEnumerable<WoWUnit> results = ObjectManager.GetObjectWoWUnit().Where(u => u.Type == objectType && u.IsAlive && u.MaxHealth > 500 && (u.Position.DistanceTo2D(instance.Position) <= range) && u.IsAttackable && !TraceLine.TraceLineGo(u.Position)); // Return return results; }
Sample:
bool useSwipe = (ObjectManager.Me.GetAttackableUnits(5).Count() >= 3);
Thanks for this, seems way more neat than what I have going on at the min. Another issue I've ran into though is when there's more than 1 feral in the group it doesn't keep up rake anymore since it can see his rake debuff on the boss. Is there any way to make it use my personal applied debuffs rather than everyones?
Here's my rake snippet:
// Rake if (Rake.KnownSpell && Rake.IsSpellUsable && Rake.IsDistanceGood && !ObjectManager.Target.HaveBuff(9904) && ObjectManager.Target.BuffTimeLeft(new List<uint> { 9904 }) < 1000) { Rake.Launch(); Lua.LuaDoString(@"dRotationFrame.text:SetText(""Casting Rake"")"); return; }
-
20 minutes ago, Marsbar said:
Oh lol dude, add a
using System.Linq;to the usings at the top of the file
I can't believe it was something so simple hahah! I can tweak around with more things now at least thanks so much! I just gotta figure out how to stop the other spells casting now instead of swipe but I'm sure I can figure that out.
Edit:
I just used an else if statement in the swipe spell and it works perfectly, so now it'll cast swipe if 3 targets and shred if it's less than that. Thanks so much!
9 minutes ago, Zan said:Go download VS Community Edition and add WRobot references while writing in C#
Yeah makes a lot of sense, I've just been a bit of a fool and stuck to using NP++ for a while now, I'll swap over to that thank you again also.
-
20 minutes ago, Marsbar said:
This should be enough as your if statement:
if (Swipe.KnownSpell && Swipe.IsSpellUsable && Swipe.IsDistanceGood && ObjectManager.Me.ComboPoint <= 3 && ObjectManager.GetWoWUnitAttackables().Count(u => u.Position.DistanceTo(ObjectManager.Target.Position) <= 8 && u.Guid != ObjectManager.Pet.Guid) >= 3)I get the same error every time, I really appreciate all the help I'm receiving. The error looks the same as everyone elses suggestion though, .Count cannot be used like a method.
-
3 hours ago, Apexx said:
Here's what I use to check attackers in range:
public static int GetAttackerCountInRange(float yards) { int EnemyCountInRange = ObjectManager.GetUnitAttackPlayer().Count(t => t.IsAlive && t.IsValid && t.IsAttackable && t.InCombat && t.IsTargetingMeOrMyPetOrPartyMember && t.GetDistance <= yards); if (EnemyCountInRange > 0) { Logging.WriteDebug($"Enemy attacking count = {EnemyCountInRange}"); return EnemyCountInRange; } return 0; }
Can you show how you've implemented this? I'm pretty nooby when it comes to c#. I've tried putting the code in its own spot then calling GetAttackerCountInRange in the swipe spell however I run into an error saying this:
in reference to your third line.
-
12 hours ago, Zan said:
ObjectManager.GetWoWUnitHostile......
Implementing this code into the fight class base I'm using doesn't appear to work, wrobot just throws up errors. I've attached the fight class below and an image with said error, thanks again for your help.
-
8 minutes ago, Zan said:
It looks more related to non C# profiles but I'm unsure exactly how I'd implement that code into my fight class
-
I'd like to use Swipe in cat form when there's a certain amount of mobs in range but I can't seem to find any api information on how to accomplish that.
I've attached the base I've been using below if you have any suggestions.
Thanks!
-
1 hour ago, Talamin said:
You can check this out, really good Base for a Fightclass!
I was more looking for something implemented into the wrobot api itself so I wouldn't have to rewrite my whole fight class sorry, but that looks real nice!
Maybe there's something I can use with ObjectManager like unit count that's in range of me or something?
-
Is there any snippets for having more than 1 target? I'd like to use Swipe in AoE 2+ targets and Shred in single target
-
That fixed it for the target dummy thanks! not too sure about players though
-
I edited a fightclass someone made for mutilate so it would work on Ascension wow server, it works in dungeons and raids perfectly however it won't attack players or the target dummies. I'll upload it here so you can have a look and I'll attach my settings below so you can suggest anything.
-
On 11/8/2020 at 4:14 PM, Droidz said:
Hello, in product settings try to put lower value at the option "Bobber search time"
sorry for late response it's still occurring. Here's all my settings if you have any other suggestions
-
Hey so I've ran into a small issue with fishing in which it just fails to click the bobber sometimes, I've noticed it's generally when there's a fish caught as soon as I cast the rod like within the first second, I don't know if this issue is just specific to the server I play on but it's pretty annoying when most of the casts I do I have a fish hooked just as the rod is cast and the bot lets it get away.
I've tried adjusting latency to 500 - 1000 and that doesn't seem to have helped at all.
-
Sorry to revive an old thread but I'm having issues with the script above recognizing the queue popping up.
using wManager.Wow.Enums; using wManager.Wow.Helpers; using System.Collections.Generic; public class Main : wManager.Plugin.IPlugin { public void Initialize() { EventsLuaWithArgs.OnEventsLuaWithArgs += MyLuaHandler; } public void Dispose() { EventsLuaWithArgs.OnEventsLuaWithArgs -= MyLuaHandler; } private void MyLuaHandler(LuaEventsId id, List<string> args) { if (id == LuaEventsId.LFG_PROPOSAL_SHOW) { Lua.LuaDoString("LFDDungeonReadyDialogEnterDungeonButton:Click()"); } } public void Settings() { // no settings } }
Is it possible there's a different event other than LFG_PROPOSAL_SHOW ? if so how would I go about finding it? on 3.3.5
Ascension WoW
in WRobot for Wow Wrath of the Lich King - Help and support
Posted
I know this thread is old but even when having the game in windowed mode, running via Wow.exe and all the right settings the above memory error occurs no matter what. Those errors happen after the game crashes completely and the window disappears.
Honestly people getting banned were probably running mining/leveling whatever scripts. I purely used Wrobot to write my own custom rotation scripts but now I can't get it to run at all on ascension.