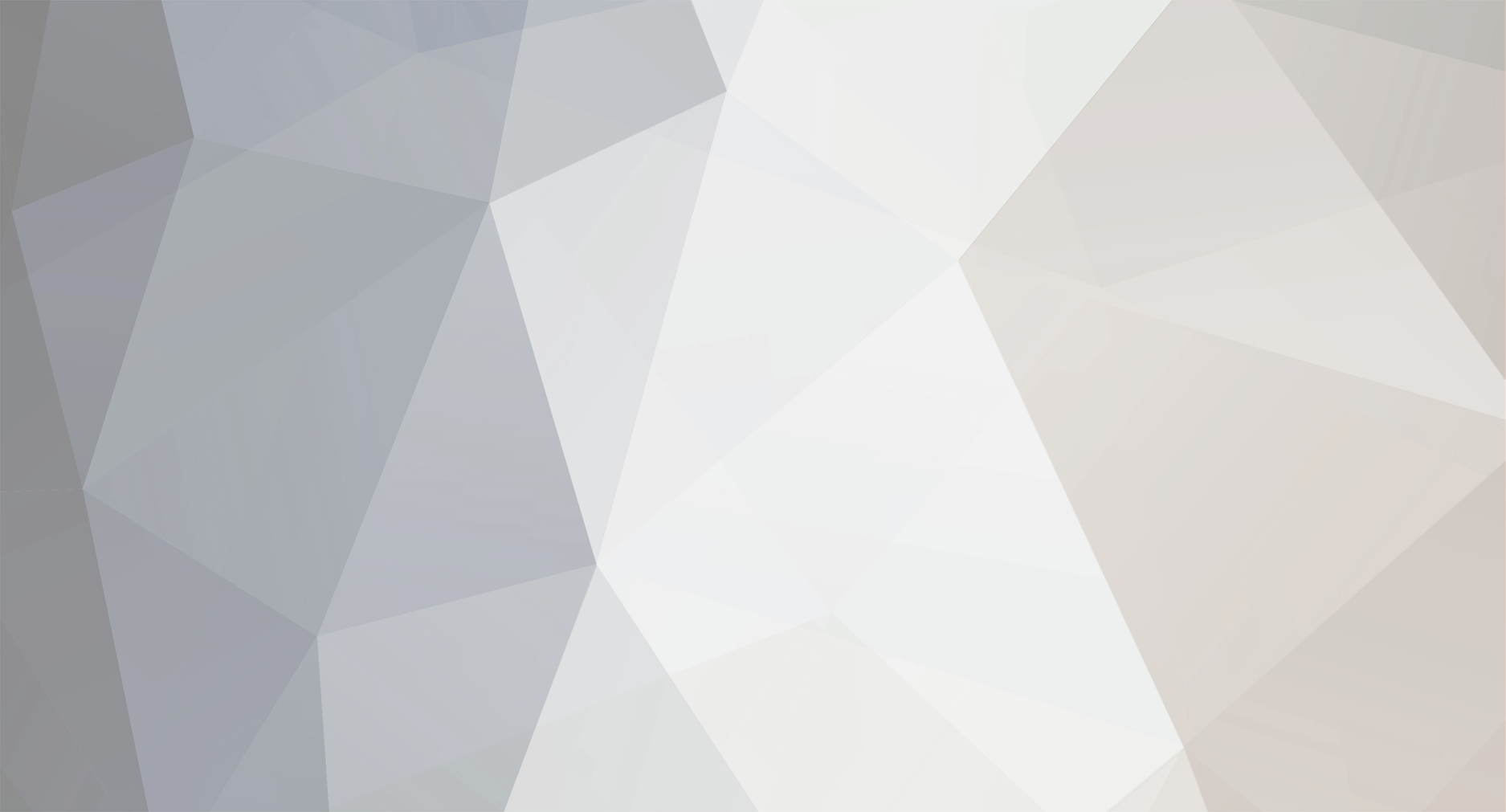
superkillen
-
Posts
23 -
Joined
-
Last visited
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Posts posted by superkillen
-
-
13 minutes ago, The Smokie. said:
I’ll have a look at it once I finish what I’m doing.
cheers man, I am pretty sure that it's not my computer since my other profile on my main (dps) is working just fine without Fps drop...
-
Hi!
I've tried to do a healing FC for my character or ascension private server.Everything works great except for when my FC targets someone to heal them I go from around 144 fps down to almost freeze for a second..
This happens everytime my FC tries to target another player.when it casts heals on myself it's flawless.
what can be the cause of this issue?
-
when I am riding vehicles (like the animals in the PvP world quest) my rotation doesn't start, I wonder if there is a easy fix in the options for something like this? played around with cast when mounted but it didn't work.
-
been trying to configure my Vengence demon hunter to do Spirit bomb when I got Soul Fragments, but when I have this
<FightClassSpell>
<FightClassConditions>
<FightClassCondition>
<ContionType>BuffStack</ContionType>
<Param xsi:type="FightClassConditionBuffStack">
<Number>1</Number>
<Type>BiggerOrEqual</Type>
<Name>Soul Fragments</Name>
</Param>
</FightClassCondition>
</FightClassConditions>
<SpellName>Spirit Bomb</SpellName>
<Priority>4</Priority>
</FightClassSpell>he doesn't do Spirit Bomb at all, but when I do it with condition Buff and type Soul Fragments True he does it, but he pretty much spams it even if he doesn't have any soul fragments.
fixed it, C sharp code: wManager.Wow.ObjectManager.ObjectManager.Me.BuffStack(203981) >= 4
works on Soul Fragments but BuffStack condition don't! -
*poke* I've got this problem aswell....
same stuff happens to my prot pala
-
yeah this is a little over the top for my knowledge, I will just add all the debuffs :)
thanks for your help! -
1 minute ago, Schaka said:
You just add the debuffname, like such:
local buffNames = { ["Toxic Saliva"] = true, ["Shadow Word: Pain"] = true, };
will be a long list... would it be able to do it with debuffType?
-
2 hours ago, Schaka said:
UnitBuff looks like this in TBC
local name, rank, iconTexture, count, duration, timeLeft = UnitBuff(unit, buffIndex[, castable]);
UnitDebuff looked like this:
local name, rank, iconTexture, count, debuffType, duration, timeLeft = UnitDebuff(unitID, debuffIndex [, removable]);
local buffNames = { ["Toxic Saliva"] = true, }; for i=1,40 do local name, rank, iconTexture, count, debuffType, duration, timeLeft = UnitDebuff("player", i) if (buffNames[name]) then result=1 return; end end
you sir is a god among men.
worked perfect, so if I want more debuffs cleansed, do I have to do a new cleanse for every debuff? or could I add so it cleanses all poison/disease/magic?
-
Hi!
I've created a fightclass for my paladin while leveling, I want him to cleanse nasty debuffs... (the one I am trying to cleanse now is Toxic Saliva, spell ID 7125he doesn't do nothing about it when I have
Return value research: 1
Return value var: result
but if I delete both those lines he just spams cleanse (it works but that's just cuz I've got it in the last spots in my priority list)
my question is, what have I done wrong? :(
-
hello!
my bot keeps reloading in combat every 30-60 min or so, pretty annoying when she does it in a middle of a fight :(
is there a fix to make this manually instead? I guess it does it to clear the memory.... -
yepp, this log is from fighting 3 harpys stacked on top off each other
-
still uses blood strike instead of pestilence, could it be some other kind of option?
-
ah missclick :( still I did change it now and it still does blood strike....
-
works great now, except for one thing... now he doesn't do pestilence when there are 3+ targets around... instead he does blood strike and pestilence is higher in priority list
-
oh my god.... thank you a lot for quick response Runaro!
cheers! -
hello I wonder why my rotation does icy touch before howling blast when there are more then 2 targets around my target :(
I've got howling blast as nr.1 in my priority list -
got MSI Z97 as motherboard and it said intel Z97 as chipset, downloaded it now and hopes it works! :)
-
hello!
I am using your 3.3.5 bot and my wow freezes sometimes and sometimes it just randomly reloads... why is this?
fps drop when bot switches target.
in Fight Classes assistance
Posted
yeah I know that's what The Ascension private server are all about, it's classless high risk servers ?
I will check this out!