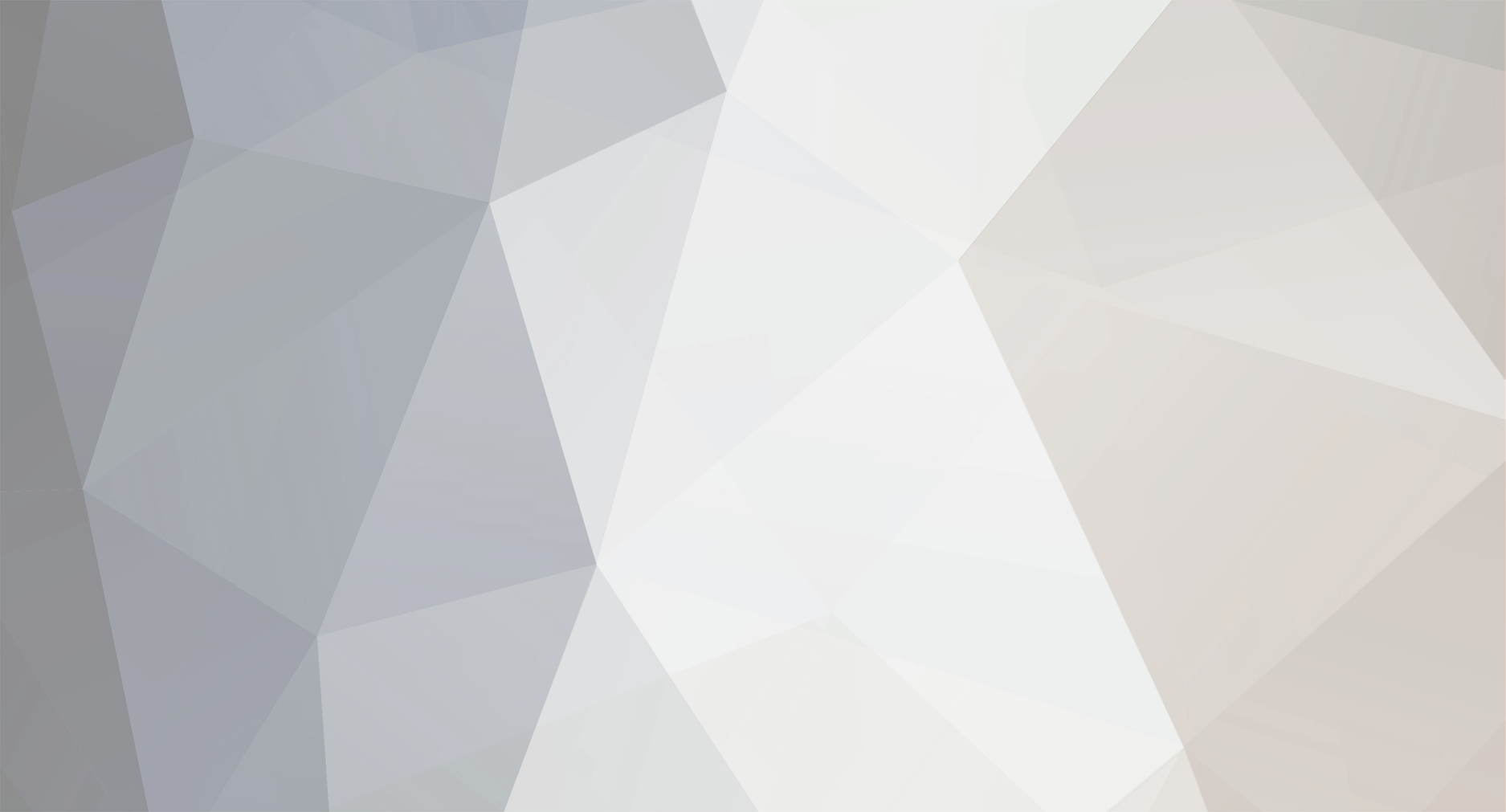
krycess
-
Posts
25 -
Joined
-
Last visited
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Posts posted by krycess
-
-
It still isn't clicking for me. Is this the right "state" that you are talking about?
internal class Fight : TreeTask { public Fight() { GrindingState = new Grinding(); } public override int Priority => 1000; public override bool Activate() { return Party.GetParty().Any(x => x.InCombat) && !ObjectManager.Me.IsSwimming; } public override void Execute() { GrindingState.Run(); } Grinding GrindingState { get; } }
-
Hi! I'm writing a new product but I don't quite know how to call a fight class from it.
for another bot, I simply called
CustomClasses.Instance.Current.Fight(ObjectManager.Instance.Units.Where(x => x.IsInCombat));
but for wRobot, I don't quite know what to call...
do I simply do this?
internal class Fight : TreeTask { public override int Priority => 1000; public override bool Activate() { return Party.GetParty().Any(x => x.InCombat) && !ObjectManager.Me.IsSwimming; } public override void Execute() { CustomClass.LoadCustomClass(); } }
-
I use these to detect shaman buffs and they work well
internal static class EnhancementHelper { public static bool HasTwoHandEquipped => Lua.LuaDoString<int>("result = IsEquippedItemType('Two-Hand')", "result") == 1; public static bool HasShieldEquipped => Lua.LuaDoString<int>("result = IsEquippedItemType('Shields')", "result") == 1; public static bool HasMainHandEnhancement => Lua.LuaDoString<int>("result = GetWeaponEnchantInfo()", "result") == 1; public static bool HasOffHandEnhancement => Lua.LuaDoString<int>("_, _, _, result = GetWeaponEnchantInfo()", "result") == 1; }
my implementation looks like this
internal class Imbue : TTask { readonly ISpellService spellService; public Imbue( ISpellService spellService) { this.spellService = spellService; } public override int Priority => 999; public override bool Activate() { return (spellService.RockbiterWeapon.KnownSpell || spellService.WindfuryWeapon.KnownSpell || spellService.FlametongueWeapon.KnownSpell) && (spellService.RockbiterWeapon.IsSpellUsable || spellService.WindfuryWeapon.IsSpellUsable || spellService.FlametongueWeapon.IsSpellUsable) && (!EnhancementHelper.HasMainHandEnhancement || (!EnhancementHelper.HasShieldEquipped && !EnhancementHelper.HasTwoHandEquipped && !EnhancementHelper.HasOffHandEnhancement)); } public override void Execute() { if (spellService.WindfuryWeapon.KnownSpell && !EnhancementHelper.HasMainHandEnhancement) spellService.WindfuryWeapon.Launch(); else if (spellService.FlametongueWeapon.KnownSpell && EnhancementHelper.HasMainHandEnhancement && !EnhancementHelper.HasOffHandEnhancement) spellService.FlametongueWeapon.Launch(); else spellService.RockbiterWeapon.Launch(); } }
-
these are mostly for my own reference but also for anyone who is looking to do the same things that I was trying to do.
these properties and methods assist in swapping warrior stances and checking for weapon enhancements such as poisons/oils/shaman enhancements etcetcetc
public static Task<bool> HasBattleStance => Task.FromResult(Lua.LuaDoString<int>("_,_,isActive,_ = GetShapeshiftFormInfo(1);", "isActive") == 1); public static Task CastBattleStanceAsync() { Lua.LuaDoString("CastSpellByName('Battle Stance')"); return Task.CompletedTask; } public static Task<bool> HasDefensiveStance => Task.FromResult(Lua.LuaDoString<int>("_,_,isActive,_ = GetShapeshiftFormInfo(2);", "isActive") == 1); public static Task CastDefensiveStanceAsync() { Lua.LuaDoString("CastSpellByName('Defensive Stance')"); return Task.CompletedTask; } public static Task<bool> HasBerserkerStance => Task.FromResult(Lua.LuaDoString<int>("_,_,isActive,_ = GetShapeshiftFormInfo(3);", "isActive") == 1); public static Task CastBerserkerStanceAsync() { Lua.LuaDoString("CastSpellByName('Berserker Stance')"); return Task.CompletedTask; } public static Task<bool> HasMainHandEnhancement => Task.FromResult(Lua.LuaDoString<int>("result = GetWeaponEnchantInfo()", "result") == 1); public static Task<bool> HasOffHandEnhancement => Task.FromResult(Lua.LuaDoString<int>("_, _, _, result = GetWeaponEnchantInfo()", "result") == 1);
-
sure thing. I'll send you a message
-
Greetings!
I have never checked out WRobot before and I am eager to jump into development.
Is there a Discord server or anyone that I can ask questions as I go along?
Calling FightClass from Product
in General assistance
Posted · Edited by krycess
yeah I wasn't planning on using the FSM so I would need to add combat behavior from scratch.
I have written all of this before for another platform so I would just have to port it over.
It would require a totally different type of "fightclass" however... so maybe it should be all inclusive before I release