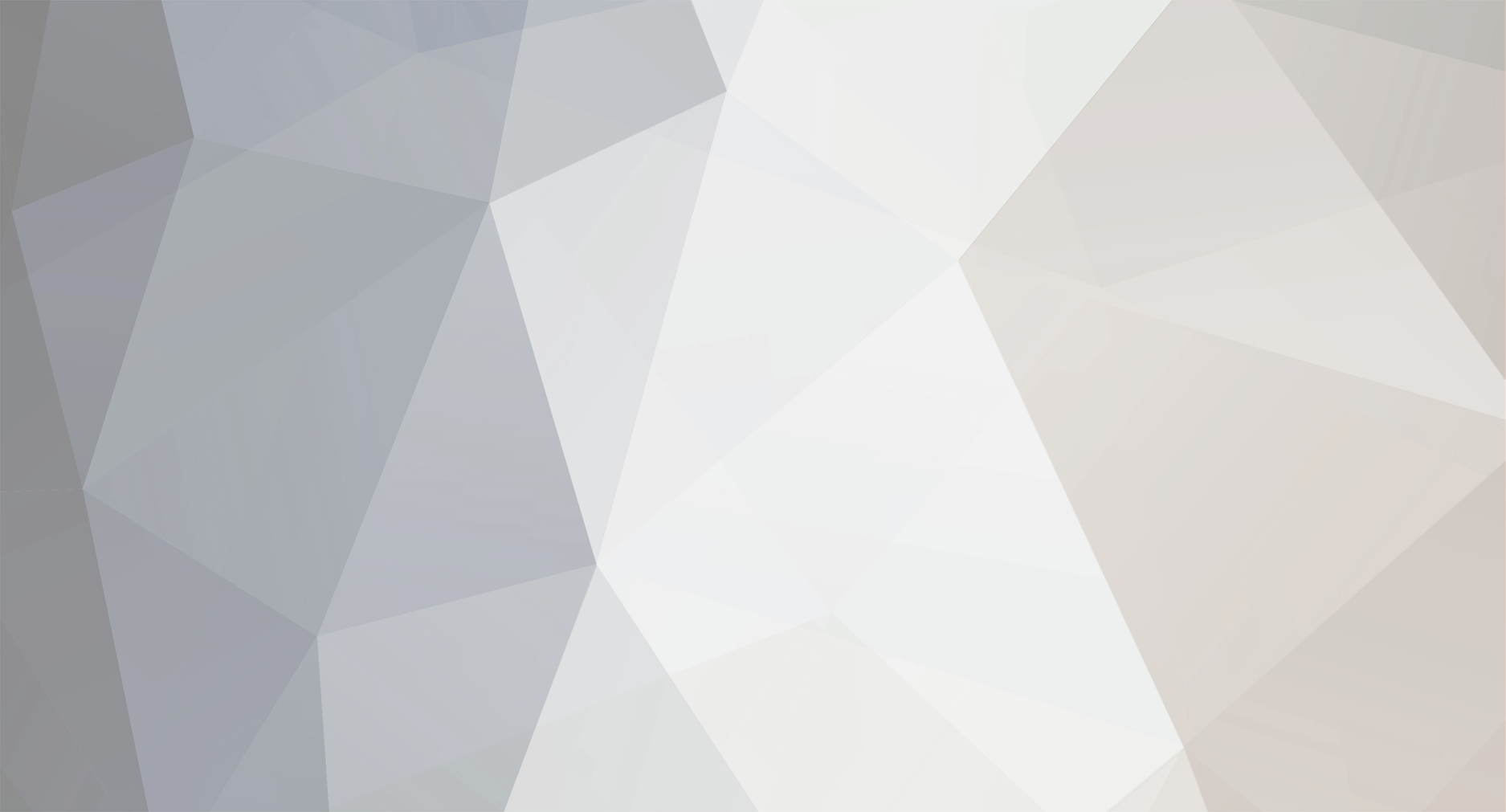
Sye
-
Posts
40 -
Joined
-
Last visited
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Posts posted by Sye
-
-
Crafting Function with a Wait while Player is Casting.
public static void Crafting(string profession, string itemName, int quantity = 0) { MovementManager.StopMoveTo(false, 1000); var ItemToCraft = Lua.LuaDoString<int>(string.Format(@" if not TradeSkillFrame or TradeSkillFrame:IsVisible() == nil then CastSpellByName('{0}') end for i=1, GetNumTradeSkills() do Quantity = {2}; local skillName, _, numAvailable = GetTradeSkillInfo(i); if (skillName == '{1}') then SelectTradeSkill(i); if Quantity == 0 then Quantity = numAvailable; end DoTradeSkill(i, Quantity); break; end end return Quantity;", profession, itemName, quantity) ); if (ItemToCraft > 0) { Logging.Write("Player is Crafting " + itemName); int Time = (3000 + Usefuls.LatencyReal) * ItemToCraft; MovementManager.StopMoveTo(false, Time); DateTime time = DateTime.Now.AddMilliseconds(Time); while (DateTime.Now < time && !ObjectManager.Me.InCombatFlagOnly && !ObjectManager.Me.InCombat) { Thread.Sleep(300); } Logging.Write("Crafting Has Came to a End."); Lua.LuaDoString("HideUIPanel(TradeSkillFrame)"); } }
-
Use Item By Name
public static void UseItemByName(string ItemName) { var useItem = string.Format(@" for bag = 0,4 do for slot = 1,GetContainerNumSlots(bag) do local item = GetContainerItemLink(bag,slot) if item and item:find('{0}') then local startTime = GetItemCooldown(GetContainerItemID(bag,slot)) if startTime <= 0 then UseItemByName('{0}') break; end end end end", ItemName); Lua.LuaDoString(useItem); }
-
Csharp LoadProfile
public class Load { public static void LoadProfile(string ProfileName) { var p = Quest.QuesterCurrentContext.Profile as QuesterProfile; if (p != null) { p.QuestsSorted.Add(new wManager.Wow.Class.QuestsSorted { Action = wManager.Wow.Class.QuestAction.LoadProfile, NameClass = ProfileName }); } } }
-
@Droidzis there a way minimize wrobot from a plugin?
-
(Csharp) - return all the Glyph a player has.
private static List<string> Glyphs() { var GlyphNames = @" local Index = {} for i = 1, GetNumGlyphSockets() do local id = select(3, GetGlyphSocketInfo(i)); if id ~= nil then local GlyphName = GetSpellInfo(id); tinsert(Index, GlyphName); end end return unpack(Index); "; return Lua.LuaDoString<List<string>>(GlyphNames); }
or if people do not wanna use .Contain in a list format. here is a boolen.
private static bool Glyphs(string GlyphNum) { var GlyphNames = $@" for i = 1, GetNumGlyphSockets() do local id = select(3, GetGlyphSocketInfo(i)); if id ~= nil then local GlyphName = GetSpellInfo(id); if GlyphName and string.find(GlyphName, ""{GlyphNum}"") return true; break; end end end return false; "; return Lua.LuaDoString<bool>(GlyphNames); }
-
function UseItemInSlow(slotId) local SlotCoolDown = select(2, GetInventoryItemCooldown("player", slotId)); local ItemEquipedName = select(1, GetItemInfo(GetInventoryItemID("player", slotId))); if SlotCoolDown == 0 then UseItemByName(ItemEquipedName) end end
-
-
Hello,
are you playing on war and?
-
If you can provide me with the rotation you awake, I can write you one.
-
Faster way of getting people to sigh your guild charter.
var PlayersAroundMe = ObjectManager.GetObjectWoWPlayer().Where(c => c.Position.DistanceTo(ObjectManager.Me.Position) < 30 && c.Faction == ObjectManager.Me.Faction && c.IsAlive); ItemsManager.UseItem("Guild Charter"); foreach (var Toon in PlayersAroundMe) { ObjectManager.Me.Target = Toon.Guid; bool PlayerHasNoGuild = Lua.LuaDoString<bool>(@" local GuildName = GetGuildInfo('target'); if GuildName == nil then return true; else return false; end"); Thread.Sleep(100); if (PlayerHasNoGuild) { Chat.SendChatMessageWhisper("Can you please sigh my guild Chart, pretty please :)", Toon.Name); Thread.Sleep(100); Lua.LuaDoString("OfferPetition();"); Thread.Sleep(100); Logging.Write("Petition Offered to :" + Toon.Name); } }
-
Private servers only.
-
wManager.wManagerSetting.CurrentSetting.CloseIfPlayerTeleported = bool;
bool = true/false?
-
This type of feature would need to be a product to control quester.
-
using System.ComponentModel; using wManager.Events; using wManager.Plugin; using wManager.Wow.Helpers; public class Main : IPlugin { public void Initialize() { OthersEvents.OnDismount += delegate (CancelEventArgs cancelable) { Move.JumpOrAscend(); }; } public void Dispose() { } public void Settings() { } }
-
(Csharp) Return Deadly Postion Stack count.
public static int ReturnDeadlyPosionStacks { get { return Lua.LuaDoString<int>(@" local DeadlyPoison = {'Deadly Poison', 'Deadly Poison II', 'Deadly Poison III', 'Deadly Poison IV', 'Deadly Poison V', 'Deadly Poison VI', 'Deadly Poison VII','Deadly Poison VIII', 'Deadly Poison IX'} local DeadlyPoisonStackCount = 0; for Poison = 1, #DeadlyPoison do for i = 1, 20 do local name, _, _, count = UnitDebuff('target', i); if name and string.find(name, DeadlyPoison[Poison]) then DeadlyPoisonStackCount = count; end end end return DeadlyPoisonStackCount;"); } }
-
Return Deadly Poison Stack Count
Lua script :
local PoisonName = {"Deadly Poison", "Deadly Poison II", "Deadly Poison III", "Deadly Poison IV", "Deadly Poison V", "Deadly Poison VI", "Deadly Poison VII", "Deadly Poison VIII", "Deadly Poison IX"} local PoisonStackCount = 0; for Poison = 1, #PoisonName do for i= 1, 20 do local name, _, _, count = UnitDebuff("target", i); if (PoisonName[Poison] == name) then PoisonStackCount = count; end end end return PoisonStackCount;
You can edit the poisonName table and add any poison that has a stacking effect.
-
I’d love to help ?
-
using System; using System.Threading; using robotManager.Helpful; using wManager.Plugin; using wManager.Wow.Enums; using wManager.Wow.Helpers; public class Main :IPlugin { bool _Loading; private ContinentId ContinentZone = (ContinentId)Usefuls.ContinentId; public void Initialize() { _Loading = true; if(_Loading) { try { while(Conditions.InGameAndConnectedAndAliveAndProductStartedNotInPause) { if(ContinentZone == ContinentId.IsleofConquest) { Battleground.ExitBattleGround(); } Thread.Sleep(Usefuls.Latency + 250); } } catch (Exception e) { Logging.Write("error : " + e); } } } public void Dispose() { _Loading = false; } public void Settings() { Logging.Write("There are no settings."); } }
-
wManager.wManagerSetting.CurrentSetting.FlightMasterDiscoverRange = 0;
-
rotation
in General discussion
Posted
Because sirus protection is a big problem.