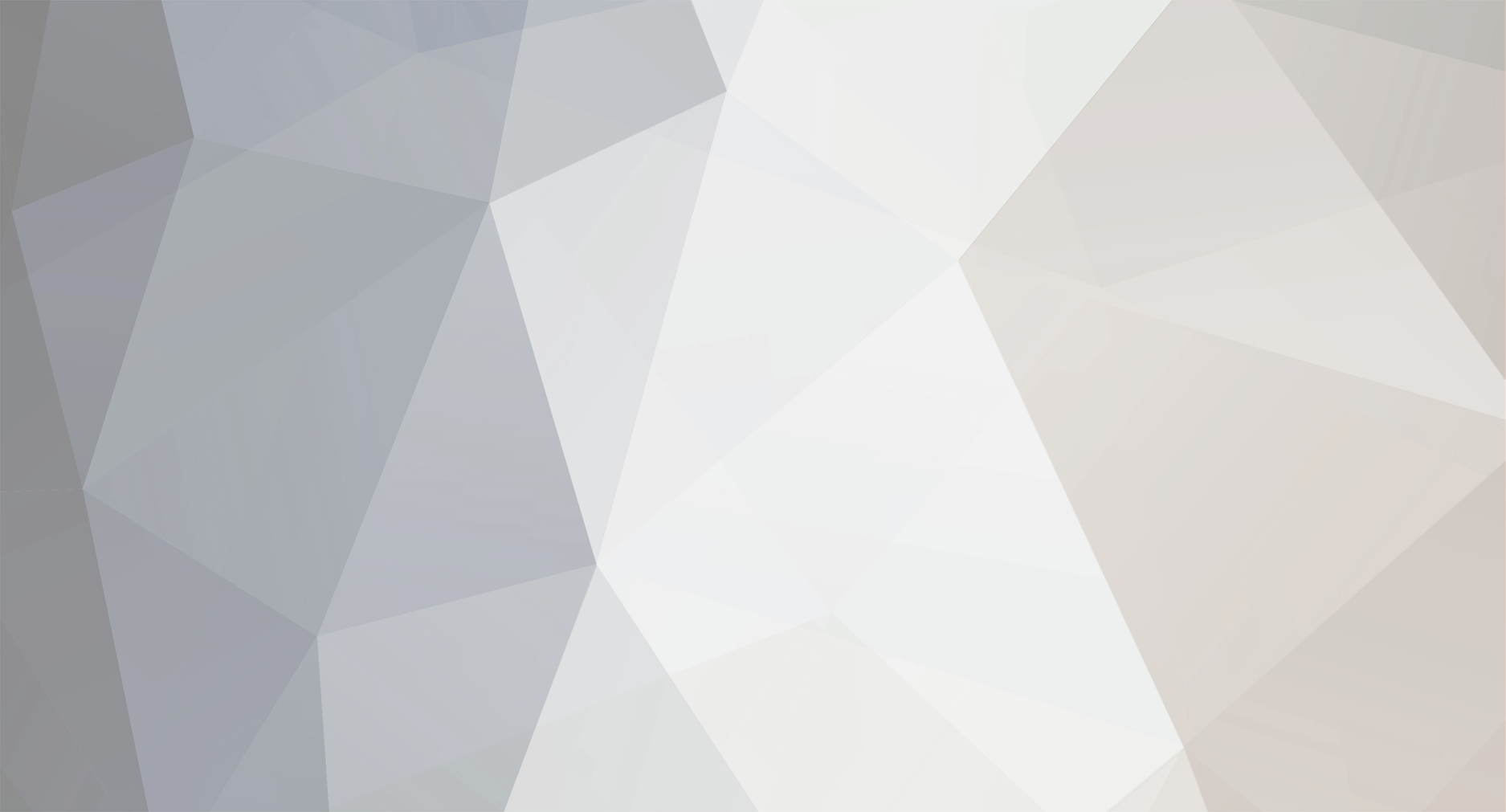
Pasterke
-
Posts
165 -
Joined
-
Last visited
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Posts posted by Pasterke
-
-
If there is any need for a pve fight class (healing, tank, dps) let me know.
I will level a toon from scratch and write it while i'm progressing.
Don't ask for PvP, I just hate PvP :)
-
-
It's OK, I restarted everything and will continue to make the fightclass :)
-
I give up. Too many System.NullReferenceException errors because of the bad use of lists.
As long as we can't refer to FirstOrDefault() == null it's impossible to get rid of those System.NullReferenceException.
Normally it's so easy, you build a list, you sort the list and you take the FirstOrDefault(). But with wrobot structure to approach units, that's impossible.
-
Why he's not casting wild mushroom ?
using System; using System.IO; using System.Collections.Generic; using System.ComponentModel; using System.Configuration; using System.Linq; using System.Text; using System.Threading; using System.Threading.Tasks; using System.Windows.Forms; using robotManager; using robotManager.FiniteStateMachine; using robotManager.Helpful; using wManager.Wow.Class; using wManager.Wow.Enums; using wManager.Wow.Helpers; using wManager.Wow.ObjectManager; public class Main : ICustomClass { private DruidResto _druid; public float Range { get { return 39.0f; } } public void Initialize() { _druid = new DruidResto(); _druid.Pulse(); } public void Dispose() { _druid.Stop(); } public void ShowConfiguration() { MessageBox.Show("No settings for this FightClass."); } class DruidResto { // Property: private bool _isLaunched; public bool IsLaunched { get { return _isLaunched; } set { _isLaunched = value; } } // Spells: private Spell _regrowth; private Spell _wildgrowth; private Spell _healingtouch; private Spell _rejuvenation; private Spell _tranquility; private Spell _swiftmend; private Spell _wildmushroom; private Spell _genesis; private Spell _typhoon; private Spell _incapacitatingroar; private Spell _rebirth; private Spell _naturesvigil; private Spell _naturesSwiftness; private Spell _lifebloom; private Spell _clearcasting; private Spell _ironbark; private Spell _barkskin; private Spell _forceofnature; public DruidResto() { _regrowth = new Spell("Regrowth"); _wildgrowth = new Spell("Wild Growth"); _healingtouch = new Spell("Healing Touch"); _rejuvenation = new Spell("Rejuvenation"); _tranquility = new Spell("Tranquility"); _swiftmend = new Spell("Swiftmend"); _wildmushroom = new Spell("Wild Mushroom"); _genesis = new Spell("Genesis"); _typhoon = new Spell("Typhoon"); _incapacitatingroar = new Spell("Incapacitating Roar"); _rebirth = new Spell("Rebirth"); _naturesvigil = new Spell("Nature's Vigil"); _naturesSwiftness = new Spell("Nature's Swiftness"); _lifebloom = new Spell("Lifebloom"); _clearcasting = new Spell("Clearcasting"); _ironbark = new Spell("Iron Bark"); _barkskin = new Spell("Barkskin"); _forceofnature = new Spell("Force of Nature"); } public void Pulse() { _isLaunched = true; var thread = new Thread(RoutineThread) { Name = "Restoration Druid FightClass" }; thread.Start(); } public void Stop() { _isLaunched = false; Logging.WriteFight("Stop 'Restoration Druid'"); } void RoutineThread() { Logging.WriteFight("'Restoration Druid' Started"); while (_isLaunched) { Routine(); Thread.Sleep(10); // Temps d'attante pour éviter d'utiliser trop le processeurs } Logging.WriteFight("'Restoration Druid' Stopped"); } public const uint WILD_GROWTHI = 48438, REGROWTHI = 8936, HEALING_TOUCHI = 5185, REJUVENATIONI = 774, SWIFTMENDI = 18562, LIFEBLOOMI = 33763, WILD_MUSHROOMI = 145205, FORCE_OF_NATUREI = 102693, TRANQUILITYI = 740, GENESISI = 145518, EINDE = 0; void Routine() { if (!Conditions.InGameAndConnectedAndAlive) return; if (Lifebloom()) return; } IEnumerable<WoWUnit> GetPartyTargets() { var partyMembers = Party.GetPartyHomeAndInstance(); var ret = new List<WoWUnit>(); foreach (var m in partyMembers) { if (m.IsValid && m.IsAlive && m.InCombat && m.Target.IsNotZero()) { var targetUnit = new WoWUnit(ObjectManager.GetObjectByGuid(m.Target).GetBaseAddress); if (targetUnit.IsValid && targetUnit.IsAlive) { ret.Add(targetUnit); } } } return ret.Distinct(); } private List<WoWPlayer> GetPartyMembers(int maxHealthPercent = 100, float maxDistance = float.MaxValue, bool orderByHealth = true) { return GetPartyMembers(ObjectManager.Me.Position, maxHealthPercent, maxDistance, orderByHealth); } private List<WoWPlayer> GetPartyMembers(Vector3 positionCenter, int maxHealthPercent = 100, float maxDistance = float.MaxValue, bool orderByHealth = true) { var partyMembers = Party.GetPartyHomeAndInstance(); var ret = new List<WoWPlayer>(); foreach (var m in partyMembers) { if (m.IsValid && m.IsAlive && m.HealthPercent < maxHealthPercent && m.Position.DistanceTo(positionCenter) <= maxDistance) { ret.Add(m); } } if (orderByHealth) ret = new List<WoWPlayer>(ret.OrderBy(p => p.HealthPercent)); return ret; } string GetTankPlayerName() { var lua = new[] { "partyTank = \"\";", "for groupindex = 1,MAX_PARTY_MEMBERS do", " if (UnitInParty(\"party\" .. groupindex)) then", " local role = UnitGroupRolesAssigned(\"party\" .. groupindex);", " if role == \"TANK\" then", " local name, realm = UnitName(\"party\" .. groupindex);", " partyTank = name;", " return;", " end", " end", "end", }; return Lua.LuaDoString(lua, "partyTank"); } WoWPlayer GetTankPlayer() { var p = new WoWPlayer(0); var playerName = GetTankPlayerName(); if (!string.IsNullOrWhiteSpace(playerName)) { playerName = playerName.ToLower().Trim(); var party = GetPartyMembers().OrderBy(o => o.GetDistance); foreach (var woWPlayer in party) { if (woWPlayer.Name.ToLower() == playerName) { p = new WoWPlayer(woWPlayer.GetBaseAddress); break; } } } if (string.IsNullOrWhiteSpace(playerName)) { p = new WoWPlayer(ObjectManager.Me.GetBaseAddress); } return p; } bool BaseHealSpell(Spell spell, int maxHealthPercent, float maxDistance) { if (!spell.KnownSpell) return false; if (!spell.IsSpellUsable) return false; var partyMembers = GetPartyMembers(); foreach (var partyMember in partyMembers) { if (!TraceLine.TraceLineGo(partyMember.Position)) // TraceLine permet de vérifier un obstacle entre deux position (true si obstacle) { Interact.InteractGameObject(partyMember.GetBaseAddress, true); // sélectionne la cible in game //MovementManager.Face(partyMember); // Faire face à la cible spell.Launch(); return true; } } return false; } bool Lifebloom() { if (!_lifebloom.KnownSpell) return false; if (!_lifebloom.IsSpellUsable) return false; var partyMembers = GetTankPlayer(); if (!TraceLine.TraceLineGo(partyMembers.Position) && partyMembers.IsAlive && partyMembers.HealthPercent > 0 && !partyMembers.HaveBuff("Lifebloom")) { Interact.InteractGameObject(partyMembers.GetBaseAddress, true); // sélectionne la cible in game //MovementManager.Face(partyMembers); // Faire face à la cible _lifebloom.Launch(); // Lancer le sort return true; } return false; } private Vector3 mushroomPosition; private WoWPlayer mushroomTarget; private DateTime mushroomTime; private uint wildMushroomID = 145205; private bool needMushroom { get; set; } bool WildMushroom() { if (!_wildmushroom.KnownSpell) return false; if (!_wildmushroom.IsSpellUsable) return false; var partyMember = GetTankPlayer(); if (!TraceLine.TraceLineGo(partyMember.Position)) // lign of sight { Interact.InteractGameObject(partyMember.GetBaseAddress, true); //select target Vector3 location = partyMember.Position; SpellManager.CastSpellByIDAndPosition(WILD_MUSHROOMI, location); mushroomTarget = new WoWPlayer(partyMember.GetBaseAddress); mushroomPosition = location; mushroomTime.AddSeconds(29); return true; } return false; } } }
-
Ok, thanks, will look at this example to create another one :)
-
I realy think someting is wrong. If you choose the option to cast a buff onSelf :
[F] 17:22:24 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:25 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:25 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:25 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:25 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:25 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:25 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:26 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:26 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:26 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:26 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:26 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:26 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:27 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:27 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:27 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:27 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:27 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:27 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:28 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:28 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:28 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:28 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:28 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:28 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:29 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:29 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:29 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:29 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:29 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:29 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:29 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:30 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:30 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:30 - Cast (onself) Mark of the Wild (Mark of the Wild) [F] 17:22:30 - Cast (onself) Mark of the Wild (Mark of the Wild) ..........
I think that's also what keeps me frustrating with my lists.
-
Ty, but :
public static List<WoWPlayer> GetFriendlyPlayersAndMe() { List<WoWPlayer> listPlayersResult = new List<WoWPlayer>(); List<WoWPlayer> allPlayers = ObjectManager.GetObjectWoWPlayer(); foreach (var player in allPlayers) { if (player.IsValid && player.IsAlive && player.PlayerFaction == ObjectManager.Me.PlayerFaction) listPlayersResult.Add(player); } listPlayersResult.Add(ObjectManager.Me); // Add your character Logging.Write("First Element: " + listPlayersResult.FirstOrDefault()); // print the first element return listPlayersResult; }
Result => 17:04:49 - First Element: wManager.Wow.ObjectManager.WoWPlayer.
Problem still exists :(
To make a Healing Routine, I need to know if the player is tank or not, to cast Lifebloom on him. Then I put the player in anoter list Tanks.
If no tanks (solo playing) then the tanks is Me, so I cast Lifebloom on myself. Same thing for Wild Mushrooms.
-
List<Object> party = new List<Object>(); var p = new WoWPlayer(0); var obj = ObjectManager.Me; if (obj.IsValid && obj.Type == WoWObjectType.Player) { p = new WoWPlayer(obj.GetBaseAddress); party.Add(p); } return party;
If i try with List<WoWUnit> or List<WoWplayer> always the same result : wManager.Wow.ObjectManager.WoWPlayer
-
-
How can I cast a spell on a specific target ?
example : Lifebloom cast on tank. Or another spell on a specific target.
it's no problem to find all those targets, but there isn't a method in de spellcasting objects to cast it on a specific target.
something like Spellmanager.Cast(string spell, wowplayer unit), without that possibilty it's almost impossible to make a healing class.
-
If you choose Powershot talent that's the result of the log :
[D] 13:25:41 - Spell(string spellName): spellName=Powershot, Id found: 177571, Name found: Powershot, NameInGame found: Powershot, know = False
Same story if you select glaive toss.
-
I tweaked this routine in that way that it works as supposed for an guardian druid.
If you like it, you can replace the one that comes with wrobot with this one.
-
I have here an bosslist with all WoW bosses in raids, instances and scenarios.
Is it possible to implent this, that way we can use our cooldowns on bosses and don't waste it on trash ?
-
Thx, that's what I wanted to know :)
-
Thx, but that's not what i'm asking. I speak here about C# and not how to build an xml fighting class.
-
We have an cast sequence rotation. Let's say :
cast spell 1
cast spell 2
cast spell 3
cast spell 4
What happens after he casts spell 1 ? He continues till the bottom of the cast sequence, or he goes back to the top of the list ?
Practical example :
If we have the buff Lock and Load then we want to cast Explosive Shot. If he returns to the top of the list, then he will cast 2 explosive shots in a row.
If he continues to the bottom of the list, then he will cast other spells between.
-
Where is that zone ?
-
He don't cast Rapid Fire and Focus Fire.
-
There's is no base spellname for Nether Tempest. You only get that skill if you choose that specific talent.
If you have Faerie Swarm and you cast Faerie Fire, then he casts Faerie Swarm. The only thing then, when he look for the debuff, it's Faerie Swarm and not Faerie Fire.
I made a solution for that one ;
new SpellState("Faerie Fire", 23, context => !MeFeralSettings.CurrentSetting.HaveFaerieSwarm && !(ObjectManager.Me.Guid == ObjectManager.Target.BuffCastedBy("Faerie Fire")), false, true, false, false, true, true, 0, false, false, false, false), new SpellState("Faerie Fire", 22, context => MeFeralSettings.CurrentSetting.HaveFaerieSwarm && !(ObjectManager.Me.Guid == ObjectManager.Target.BuffCastedBy("Faerie Swarm")), false, true, false, false, true, true, 0, false, false, false, false),
[Setting] [DefaultValue(false)] [Category("Druid Feral")] [DisplayName("Faerie Swarm Talent")] [Description("Put on true if you have that Talent")] public bool HaveFaerieSwarm { get; set; }
-
If you have spells added to your spellbook via talents, the bot don't use it and if you debug he says spellname = failed.
For mages : don't recognize Nether Tempest
For Druids : don't recognize Faerie Swarm.
-
Target Buff is only checking if the debuff is on the target, not who put the debuff on the target.
In raid, with 3 feral druids, he never put rip, rake if another druid provide the debuff.
So, you need a check from who is the debuff.
public bool debuffExists(int Debuff, WoWUnit onTarget) { if (onTarget != null) { WoWAura aura = onTarget.GetAllAuras().FirstOrDefault(a => a.SpellId == Debuff && a.CreatorGuid == Me.Guid); if (aura != null) { return true; } } return false; }
-
Thx alot :)
-
Any way to interrupt spells ?
something like :
if (SpellManager.KnownSpell(MightyBash) && (Me.CurrentTarget.IsCasting && Me.CanInterruptCurrentSpellCast)) { do something; }
A few questions about the CustomClass
in WRotation assistance
Posted
what are you trying to do ? If your interact returns null, wrobot will crash.
public WoWPlayer getNearestPlayer
{
get
{
var t = ObjectManager.GetObjectWoWPlayer().Where(p => p != null //from here on you can add checks
&& p.IsAlive
&& p.IsHorde //or p.IsAlliance
&& !TraceLine.TraceLineGo(p.Position) //test if no objects between you and target (target is in line of sight)
&& p.GetDistance <= 40).OrderBy(p => p.HealthPercent).ThenBy(p => p.GetDistance).ToList(); //1st sort on healtpercent and if you want then by distance
if (t.Count() > 0) // check if t > 0, what means he find something you ask
{
WoWPlayer v = new WoWPlayer(t.FirstOrDefault().GetBaseAddress);
return v;
}
return null; //if t == 0 then return null
}
}
then yo can use getNearestPlayer to do something in your code
if (getNearestPlayer != null)
{
try
{
Interact.InteractGameObject(getNearestPlayer.GetBaseAddress);
spell.Launch();
}
catch (Exception e) { Logging.WriteError("ErrorDescription: " + e.ToString()); }
}