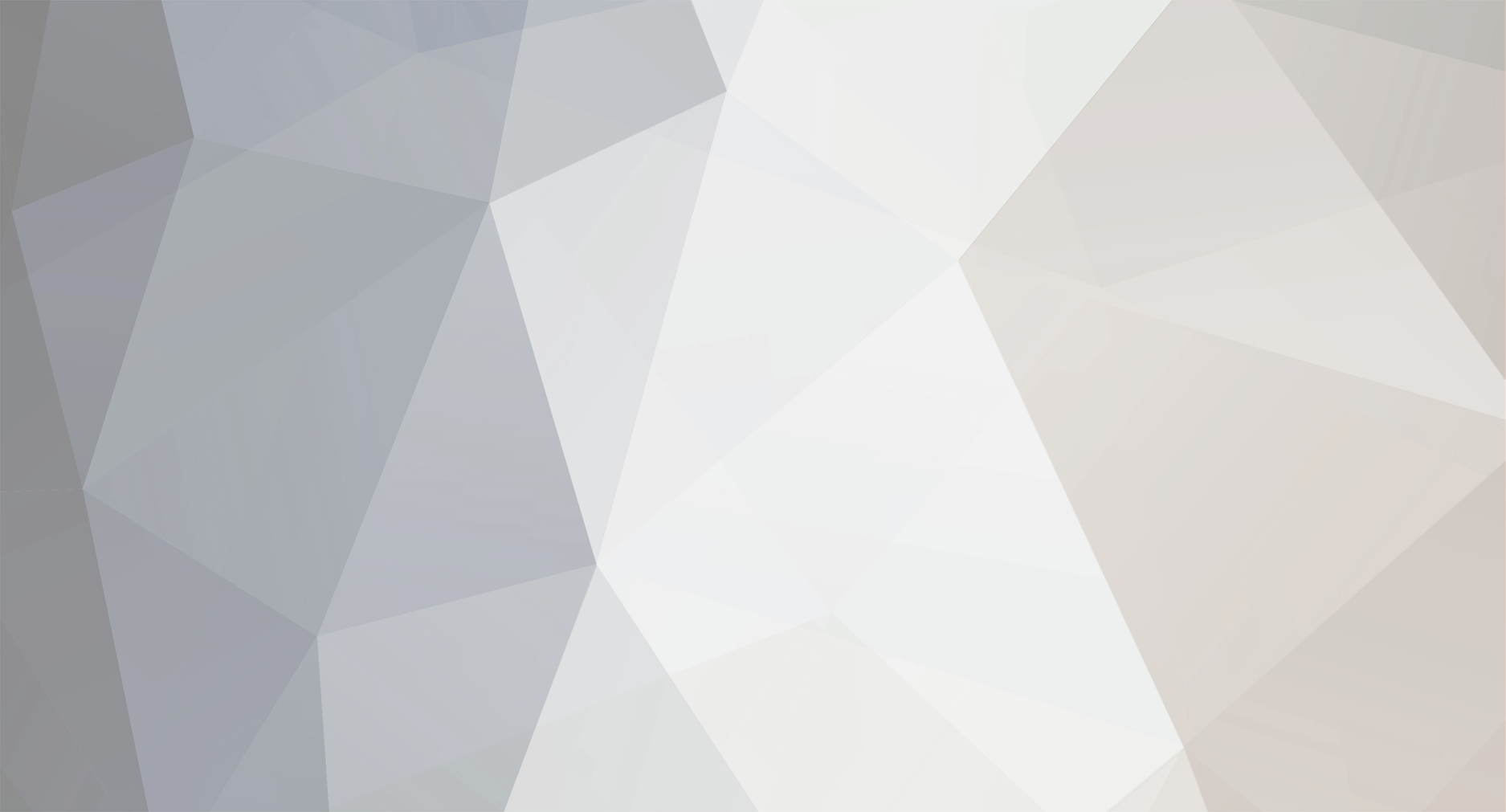
Matenia
-
Posts
2226 -
Joined
-
Last visited
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Bug Report Comments posted by Matenia
-
-
-
I did the same test yesterday (exact same in development tools). Maybe it was a bad seed or something. My computer had already been running all day.
Edit: Just did it again and it still always reports 1 - this is WRobot 32758.
I can get it to work if I execute it before even starting the bot (Quester). If I use it while the bot is running without ever using it before, it will ALWAYS result in 1.
For now, I just made my own implementation in my quester profile to work around. -
I'm sure this worked on TBC back in the day. Currently definitely broken on Vanilla.
-
No, this:
Keep in mind, I am NOT advertising this as a solution to your problems. I'm saying you should do this yourself to fit your own needs. A product that works cross expansion and is made for the public will never do exactly what you personally want it to do. -
You're still very obviously a bot. For my product, I reworked all the routes, added offmeshes and made the bot follow objects as well as possible.
Even then, you still end up getting reported. Maybe not on massive Russian WotLK servers where nobody cares anyway.
I have probably 3-4k hours of BG botting under my hood just with WRobot between Vanilla, TBC and Wrath. Trust me, when I say that just fixing the pathing itself won't be enough to not get caught. -
It's because nobody really uses the BG bot.
I tediously handcrafted routes and added offmeshes where possible in my own product.
I suggest you do the same for the best and most "natural" results. -
TraceLine.TraceLineGo returns false when there is no collision and true if there is a condition.
You need to negate the condition.
You need to use hitflags WMO for spells. -
I just want to say, that I do not use table.unpack in the fightclass and I am getting no freezes, hence why I haven't been able to reproduce this.
I'm also 99% certain that the lua error is not related to the freezes, because apparently a plugin doing ReloadUI() is not fixing it - so it isn't a GC issue -
He said he already uses a plugin like that...
That's not the solution. It's when you either click "start" too quickly twice in a row or reload the context/produt incorrectly through code. -
If use spirit healer is activated, it will ALWAYS use it.
You need to use advanced settings for how many times you die frequently to use the spirit healer dynamically. This is why it used to be a feature in HMP. Droidz implemented most of that now. -
There is no other way. If you are on their threat, you are in combat. Otherwise literally there is NO WAY. It's not in the game.
-
That's correct behavior. It only checks if you're being targeted by the unit and have combat state AND if you're not ignoring combat in settings.
If you want to know whether a unit has threat towards you, use the threat API (only works in Wotlk+) -
This is great. Now I don't have to use a Lua hook anymore.
Otherwise you can use a Lua hook to create a race condition between your selection code and ANY code turning in the quest:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Linq; using System.Reflection; using System.Security.Cryptography; using System.Text.RegularExpressions; using System.Threading; using System.Threading.Tasks; using DatabaseManager.Enums; using robotManager.Events; using robotManager.FiniteStateMachine; using robotManager.Helpful; using robotManager.Products; using wManager; using wManager.Wow.Bot.Tasks; using wManager.Wow.Class; using wManager.Wow.Enums; using wManager.Wow.Helpers; using wManager.Wow.ObjectManager; public class QuestRewardSelector { public static string TooltipName = "QuestItemCompareTooltip"; public static void Start() { TooltipName = LuaVariableSettings.CurrentSetting.QuestToolTipName; RegisterHook(); CreateTooltip(); EventsLua.AttachEventLua((LuaEventsId) Enum.Parse(typeof(LuaEventsId), "QUEST_COMPLETE"), QuestCompleteHandler); } public static void Stop() { } public static void CreateTooltip() { Lua.LuaDoString($@" if not {TooltipName} then {TooltipName} = CreateFrame('GameTooltip', '{TooltipName}', nil, 'GameTooltipTemplate'); {TooltipName}:SetOwner(UIParent, 'ANCHOR_NONE'); end "); } public static void RegisterHook() { Lua.LuaDoString($@" if (not {LuaVariableSettings.CurrentSetting.QuestHookName}) then _OriginalQuestCompleteOnClick = QuestFrameCompleteQuestButton:GetScript(""OnClick""); function hook_QuestComplete(self, button, arg2, arg3) self = this; if (button == ""FORCE"") then --DEFAULT_CHAT_FRAME:AddMessage('Forcing quest acceptance!'); _OriginalQuestCompleteOnClick(self, button); else C_Timer.After(2500, function() --DEFAULT_CHAT_FRAME:AddMessage('Delay quest turn in by 2500ms'); _OriginalQuestCompleteOnClick(self, button); end); end end QuestFrameCompleteQuestButton:SetScript(""OnClick"", hook_QuestComplete); _GetQuestReward = GetQuestReward; function GetQuestReward(index, force) if(force) then return _GetQuestReward(index); else C_Timer.After(2500, function() --DEFAULT_CHAT_FRAME:AddMessage('Delay quest turn in by 2500ms'); return _GetQuestReward(index); end); end end --DEFAULT_CHAT_FRAME:AddMessage('Quest hook initiated'); {LuaVariableSettings.CurrentSetting.QuestHookName} = true; end "); } private static void QuestCompleteHandler(object context) { Lua.LuaDoString("QuestFrameCompleteQuestButton:Disable();"); PluginLog.Log("About to complete quest - INTERCEPT!"); SelectQuestItem(); Lua.LuaDoString("QuestFrameCompleteQuestButton:Enable();"); //fallback Lua.LuaDoString($"_GetQuestReward(1);"); } private static void SelectQuestItem() { try { int questRewards = Lua.LuaDoString<int>("return GetNumQuestChoices()"); if (questRewards == 0) { return; } Dictionary<ParsedItem, int> itemRewards = new Dictionary<ParsedItem, int>(); for (int i = 1; i <= questRewards; i++) { Lua.LuaDoString($@" {TooltipName}:Hide(); {TooltipName}:SetOwner(UIParent, 'ANCHOR_NONE'); {TooltipName}:ClearLines(); {TooltipName}:SetQuestItem(""choice"", {i}); "); ParsedItem item = ItemParser.ParseCurrentTooltip(TooltipName, true); if (item.isUsable) { itemRewards.Add(item, i); } } ItemComparator comparator = new ItemComparator(StatWeights.ForClass(ObjectManager.Me.WowClass)); ParsedItem bestItem = comparator.FindBestItem(itemRewards.Keys.ToList()); int index = itemRewards[bestItem]; PluginLog.Log("Found best item " + bestItem.name + " as reward number: " + index); Lua.LuaDoString($@" --QuestFrameCompleteQuestButton:Enable(); _GetQuestReward({index}); "); Thread.Sleep(1000); } catch (Exception e) { Logging.WriteError("" + e); } } private static async void SelectQuestItemAsync() { await Task.Run(() => { SelectQuestItem(); }); } }
-
Thank you, I did something similar but this should help in case it reloads the engine (Quester reload etc).
-
OnAddState does not work it seems. It's never called inside the plugin.
OnStartEngine, all states are missing. -
When I use OnStartEngine, none of the states have been added yet and I need to get their priority (and in some cases, I need to add NextStates).
I will try OnAddState I think -
Even with offmesh distance 1000+ it does not work.
I PM'd you the code, you can take a look. It's 6 different offmeshes for all directions. -
Can you remove the path I have shown anyway?
It is wrong. The bot falls down a wall. There is no valid path down there. -
In my case, I have 6 offmeshes.
Darnassus Portal Down
Darnassus Portal Down and Auberdine
Ruth'eran to Auberdine
So depending on where the bot is, it should always find the correct connection, I think.
I will try incrementing search distance. -
You need to add UseEvenIfCanFindPath or w/e it's called to the connection.
-
Seems to work, haven't had a problem today. Consider it fixed
-
That should not cause the fightclass to load twice creating a deadlock on levelup, right?
Atm if you level up, what happens in the log I posted above keeps happening. I think even without framelock -
Could it be because I call
SpellManager.UpdateSpellBook();
after successful training (in my own training state)?
-
16:54:53 - Level UP! Reload Fight Class. 16:54:53 - [WarlockTBC]: Force closing authentication thread 16:54:53 - Unloaded Warlock Fightclass [F] 16:54:54 - [FightClass] Loading Fight class: F:\Games\WoW 2.4.3\wRobot2.4.3\\FightClass\WarlockTBC.dll 16:54:54 - [Looting] Loot Defias Night Blade [D] 16:54:54 - [Spell] Create Healthstone (Id found: 6201, Name found: Create Healthstone, NameInGame found: Create Healthstone, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Create Healthstone (Id found: 6201, Name found: Create Healthstone, NameInGame found: Create Healthstone, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Create Healthstone (Id found: 6201, Name found: Create Healthstone, NameInGame found: Create Healthstone, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Create Healthstone (Id found: 6201, Name found: Create Healthstone, NameInGame found: Create Healthstone, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Create Healthstone (Id found: 6201, Name found: Create Healthstone, NameInGame found: Create Healthstone, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Create Healthstone (Id found: 6201, Name found: Create Healthstone, NameInGame found: Create Healthstone, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Create Soulstone (Id found: 693, Name found: Create Soulstone, NameInGame found: Create Soulstone, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Create Soulstone (Id found: 693, Name found: Create Soulstone, NameInGame found: Create Soulstone, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Create Soulstone (Id found: 693, Name found: Create Soulstone, NameInGame found: Create Soulstone, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Create Soulstone (Id found: 693, Name found: Create Soulstone, NameInGame found: Create Soulstone, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Create Soulstone (Id found: 693, Name found: Create Soulstone, NameInGame found: Create Soulstone, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Create Soulstone (Id found: 693, Name found: Create Soulstone, NameInGame found: Create Soulstone, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Create Healthstone (Id found: 6201, Name found: Create Healthstone, NameInGame found: Create Healthstone, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Create Soulstone (Id found: 693, Name found: Create Soulstone, NameInGame found: Create Soulstone, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Life Tap (Id found: 1454, Name found: Life Tap, NameInGame found: Life Tap, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Demon Skin (Id found: 687, Name found: Demon Skin, NameInGame found: Demon Skin, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Demon Armor (Id found: 706, Name found: Demon Armor, NameInGame found: Demon Armor, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Fel Armor (Id found: 44977, Name found: Fel Armor, NameInGame found: Fel Armor, Know = False, IsSpellUsable = False) [D] 16:54:54 - [Spell] Unending Breath (Id found: 5697, Name found: Unending Breath, NameInGame found: Unending Breath, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Consume Shadows (Id found: 36472, Name found: Consume Shadows, NameInGame found: Consume Shadows, Know = False, IsSpellUsable = True) [D] 16:54:54 - [Spell] Summon Imp (Id found: 688, Name found: Summon Imp, NameInGame found: Summon Imp, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Summon Voidwalker (Id found: 697, Name found: Summon Voidwalker, NameInGame found: Summon Voidwalker, Know = True, IsSpellUsable = True) [D] 16:54:54 - [Spell] Summon Felguard (Id found: 30146, Name found: Summon Felguard, NameInGame found: Summon Felguard, Know = False, IsSpellUsable = False) [D] 16:54:54 - [Spell] Health Funnel (Id found: 755, Name found: Health Funnel, NameInGame found: Health Funnel, Know = True, IsSpellUsable = True) [D] 16:54:55 - [Spell] Drain Life (Id found: 689, Name found: Drain Life, NameInGame found: Drain Life, Know = True, IsSpellUsable = True) [D] 16:54:55 - [Spell] Soul Link (Id found: 25228, Name found: Soul Link, NameInGame found: Soul Link, Know = False, IsSpellUsable = False) [D] 16:54:55 - [Spell] Fel Domination (Id found: 18708, Name found: Fel Domination, NameInGame found: Fel Domination, Know = False, IsSpellUsable = False) [D] 16:54:55 - [Spell] Immolate (Id found: 348, Name found: Immolate, NameInGame found: Immolate, Know = True, IsSpellUsable = True) [D] 16:54:55 - [Spell] Corruption (Id found: 172, Name found: Corruption, NameInGame found: Corruption, Know = True, IsSpellUsable = True) [D] 16:54:55 - [Spell] Shadow Bolt (Id found: 686, Name found: Shadow Bolt, NameInGame found: Shadow Bolt, Know = True, IsSpellUsable = True) [D] 16:54:55 - [Spell] Shoot (Id found: 5019, Name found: Shoot, NameInGame found: Shoot, Know = True, IsSpellUsable = True) [D] 16:54:55 - [Spell] Fear (Id found: 5782, Name found: Fear, NameInGame found: Fear, Know = True, IsSpellUsable = True) [D] 16:54:55 - [Spell] Drain Soul (Id found: 1120, Name found: Drain Soul, NameInGame found: Drain Soul, Know = True, IsSpellUsable = True) [D] 16:54:55 - [Spell] Unstable Affliction (Id found: 43523, Name found: Unstable Affliction, NameInGame found: Unstable Affliction, Know = False, IsSpellUsable = False) [D] 16:54:55 - [Spell] Fear (Id found: 5782, Name found: Fear, NameInGame found: Fear, Know = True, IsSpellUsable = True) [D] 16:54:55 - [Spell] Death Coil (Id found: 46283, Name found: Death Coil, NameInGame found: Death Coil, Know = False, IsSpellUsable = False) [D] 16:54:55 - [Spell] Sacrifice (Id found: 34661, Name found: Sacrifice, NameInGame found: Sacrifice, Know = False, IsSpellUsable = True) [D] 16:54:55 - [Spell] Suffering (Id found: 33703, Name found: Suffering, NameInGame found: Suffering, Know = False, IsSpellUsable = False) [D] 16:54:55 - [Spell] Berserking (Id found: 26635, Name found: Berserking, NameInGame found: Berserking, Know = False, IsSpellUsable = False) [D] 16:54:55 - [Spell] Shadowburn (Id found: 30546, Name found: Shadowburn, NameInGame found: Shadowburn, Know = False, IsSpellUsable = False) [D] 16:54:55 - [Spell] Amplify Curse (Id found: 18288, Name found: Amplify Curse, NameInGame found: Amplify Curse, Know = True, IsSpellUsable = True) [D] 16:54:55 - [Spell] Howl of Terror (Id found: 39048, Name found: Howl of Terror, NameInGame found: Howl of Terror, Know = False, IsSpellUsable = False) [D] 16:54:55 - [Spell] Siphon Life (Id found: 41597, Name found: Siphon Life, NameInGame found: Siphon Life, Know = False, IsSpellUsable = False) [D] 16:54:55 - [Spell] Curse of Agony (Id found: 980, Name found: Curse of Agony, NameInGame found: Curse of Agony, Know = True, IsSpellUsable = True) [D] 16:54:55 - [Spell] Siphon Life (Id found: 41597, Name found: Siphon Life, NameInGame found: Siphon Life, Know = False, IsSpellUsable = False) [D] 16:54:55 - [Spell] Curse of Agony (Id found: 980, Name found: Curse of Agony, NameInGame found: Curse of Agony, Know = True, IsSpellUsable = True) 16:54:55 - Unloaded Warlock Fightclass [D] 16:54:55 - Deserialize<T>(TextReader reader): System.Threading.ThreadAbortException: Thread was being aborted. at System.RuntimeTypeHandle.IsSecurityTransparent(RuntimeTypeHandle typeHandle) at System.RuntimeType.get_IsSecurityTransparent() at System.Reflection.CustomAttribute.AllowCriticalCustomAttributes(RuntimeType type) at System.Reflection.CustomAttribute.GetCustomAttributes(RuntimeType type, RuntimeType caType, Boolean inherit) at System.RuntimeType.GetCustomAttributes(Type attributeType, Boolean inherit) at System.Xml.Serialization.TempAssembly.LoadGeneratedAssembly(Type type, String defaultNamespace, XmlSerializerImplementation& contract) at System.Xml.Serialization.XmlSerializer..ctor(Type type, String defaultNamespace) at robotManager.Helpful.XmlSerializer.Deserialize[T](TextReader reader) [D] 16:54:55 - F:\Games\WoW 2.4.3\wRobot2.4.3\\Settings\CustomClass-Warlock-Name.Sunstrider.xml - Deserialize<T>(String path): System.Threading.ThreadAbortException: Thread was being aborted. at robotManager.Helpful.XmlSerializer.Deserialize[T](TextReader reader) at robotManager.Helpful.XmlSerializer.Deserialize[T](String path) [D] 16:54:56 - [MovementManager] Avoid wall: StrafeLeft [F] 16:54:56 - [FightClass] Loading Fight class: F:\Games\WoW 2.4.3\wRobot2.4.3\\FightClass\WarlockTBC.dll [D] 16:54:56 - [Spell] Create Healthstone (Id found: 6201, Name found: Create Healthstone, NameInGame found: Create Healthstone, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Create Healthstone (Id found: 6201, Name found: Create Healthstone, NameInGame found: Create Healthstone, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Create Healthstone (Id found: 6201, Name found: Create Healthstone, NameInGame found: Create Healthstone, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Create Healthstone (Id found: 6201, Name found: Create Healthstone, NameInGame found: Create Healthstone, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Create Healthstone (Id found: 6201, Name found: Create Healthstone, NameInGame found: Create Healthstone, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Create Healthstone (Id found: 6201, Name found: Create Healthstone, NameInGame found: Create Healthstone, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Create Soulstone (Id found: 693, Name found: Create Soulstone, NameInGame found: Create Soulstone, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Create Soulstone (Id found: 693, Name found: Create Soulstone, NameInGame found: Create Soulstone, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Create Soulstone (Id found: 693, Name found: Create Soulstone, NameInGame found: Create Soulstone, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Create Soulstone (Id found: 693, Name found: Create Soulstone, NameInGame found: Create Soulstone, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Create Soulstone (Id found: 693, Name found: Create Soulstone, NameInGame found: Create Soulstone, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Create Soulstone (Id found: 693, Name found: Create Soulstone, NameInGame found: Create Soulstone, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Create Healthstone (Id found: 6201, Name found: Create Healthstone, NameInGame found: Create Healthstone, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Create Soulstone (Id found: 693, Name found: Create Soulstone, NameInGame found: Create Soulstone, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Life Tap (Id found: 1454, Name found: Life Tap, NameInGame found: Life Tap, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Demon Skin (Id found: 687, Name found: Demon Skin, NameInGame found: Demon Skin, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Demon Armor (Id found: 706, Name found: Demon Armor, NameInGame found: Demon Armor, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Fel Armor (Id found: 44977, Name found: Fel Armor, NameInGame found: Fel Armor, Know = False, IsSpellUsable = False) [D] 16:54:56 - [Spell] Unending Breath (Id found: 5697, Name found: Unending Breath, NameInGame found: Unending Breath, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Consume Shadows (Id found: 36472, Name found: Consume Shadows, NameInGame found: Consume Shadows, Know = False, IsSpellUsable = True) [D] 16:54:56 - [Spell] Summon Imp (Id found: 688, Name found: Summon Imp, NameInGame found: Summon Imp, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Summon Voidwalker (Id found: 697, Name found: Summon Voidwalker, NameInGame found: Summon Voidwalker, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Summon Felguard (Id found: 30146, Name found: Summon Felguard, NameInGame found: Summon Felguard, Know = False, IsSpellUsable = False) [D] 16:54:56 - [Spell] Health Funnel (Id found: 755, Name found: Health Funnel, NameInGame found: Health Funnel, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Drain Life (Id found: 689, Name found: Drain Life, NameInGame found: Drain Life, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Soul Link (Id found: 25228, Name found: Soul Link, NameInGame found: Soul Link, Know = False, IsSpellUsable = False) [D] 16:54:56 - [Spell] Fel Domination (Id found: 18708, Name found: Fel Domination, NameInGame found: Fel Domination, Know = False, IsSpellUsable = False) [D] 16:54:56 - [Spell] Immolate (Id found: 348, Name found: Immolate, NameInGame found: Immolate, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Corruption (Id found: 172, Name found: Corruption, NameInGame found: Corruption, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Shadow Bolt (Id found: 686, Name found: Shadow Bolt, NameInGame found: Shadow Bolt, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Shoot (Id found: 5019, Name found: Shoot, NameInGame found: Shoot, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Fear (Id found: 5782, Name found: Fear, NameInGame found: Fear, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Drain Soul (Id found: 1120, Name found: Drain Soul, NameInGame found: Drain Soul, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Unstable Affliction (Id found: 43523, Name found: Unstable Affliction, NameInGame found: Unstable Affliction, Know = False, IsSpellUsable = False) [D] 16:54:56 - [Spell] Fear (Id found: 5782, Name found: Fear, NameInGame found: Fear, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Death Coil (Id found: 46283, Name found: Death Coil, NameInGame found: Death Coil, Know = False, IsSpellUsable = False) [D] 16:54:56 - [Spell] Sacrifice (Id found: 34661, Name found: Sacrifice, NameInGame found: Sacrifice, Know = False, IsSpellUsable = True) [D] 16:54:56 - [Spell] Suffering (Id found: 33703, Name found: Suffering, NameInGame found: Suffering, Know = False, IsSpellUsable = False) [D] 16:54:56 - [Spell] Berserking (Id found: 26635, Name found: Berserking, NameInGame found: Berserking, Know = False, IsSpellUsable = False) [D] 16:54:56 - [Spell] Shadowburn (Id found: 30546, Name found: Shadowburn, NameInGame found: Shadowburn, Know = False, IsSpellUsable = False) [D] 16:54:56 - [Spell] Amplify Curse (Id found: 18288, Name found: Amplify Curse, NameInGame found: Amplify Curse, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Howl of Terror (Id found: 39048, Name found: Howl of Terror, NameInGame found: Howl of Terror, Know = False, IsSpellUsable = False) [D] 16:54:56 - [Spell] Siphon Life (Id found: 41597, Name found: Siphon Life, NameInGame found: Siphon Life, Know = False, IsSpellUsable = False) [D] 16:54:56 - [Spell] Curse of Agony (Id found: 980, Name found: Curse of Agony, NameInGame found: Curse of Agony, Know = True, IsSpellUsable = True) [D] 16:54:56 - [Spell] Siphon Life (Id found: 41597, Name found: Siphon Life, NameInGame found: Siphon Life, Know = False, IsSpellUsable = False) [D] 16:54:56 - [Spell] Curse of Agony (Id found: 980, Name found: Curse of Agony, NameInGame found: Curse of Agony, Know = True, IsSpellUsable = True) [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Attack Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Diplomacy Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Dodge Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: First Aid Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Mace Specialization Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Perception Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Shoot Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Skinning Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Sword Specialization Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: The Human Spirit Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Amplify Curse Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Corruption Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Corruption Rank 2 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Corruption Rank 3 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Curse of Agony Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Curse of Agony Rank 2 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Curse of Agony Rank 3 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Drain Life Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Drain Life Rank 2 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Drain Life Rank 3 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Drain Soul Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Drain Soul Rank 2 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Fear Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Life Tap Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Life Tap Rank 2 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Life Tap Rank 3 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Create Healthstone Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Create Healthstone Rank 2 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Create Soulstone Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Create Soulstone Rank 2 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Demon Armor Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Demon Armor Rank 2 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Demon Skin Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Demon Skin Rank 2 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Health Funnel Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Health Funnel Rank 2 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Health Funnel Rank 3 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Summon Imp Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Summon Voidwalker Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Unending Breath Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Immolate Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Immolate Rank 2 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Immolate Rank 3 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Immolate Rank 4 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Shadow Bolt Rank 1 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Shadow Bolt Rank 2 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Shadow Bolt Rank 3 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Shadow Bolt Rank 4 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Shadow Bolt Rank 5 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Consume Shadows Rank 2 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Sacrifice Rank 2 [F] 16:54:56 - [RTF] Fightclass framework found in spellbook: Torment Rank 3 16:54:56 - Loaded Warlock Fightclass [D] 16:54:58 - [MovementManager] Current pos: -10974,92 ; 274,0682 ; 28,50009 ; "None" - Target pos: -10969,6 ; 283,1646 ; 28,87038 ; "None" Continent: Azeroth Tile: 31.48612_52.57797 [D] 16:55:01 - [MovementManager] Current pos: -10974,92 ; 274,0682 ; 28,50009 ; "None" - Target pos: -10970,34 ; 275,3438 ; 28,84533 ; "None" Continent: Azeroth Tile: 31.48612_52.57797 [D] 16:55:03 - [Looting] Cannot loot the target (Defias Night Blade), ignore it.
Aura.GUID is zero in vanilla 1.12
in Bug Tracker
Posted
To reiterate what Droidz said - as far as I'm aware, the info is literally not available in memory to the client.
The Lua API is equally limited in 1.12.