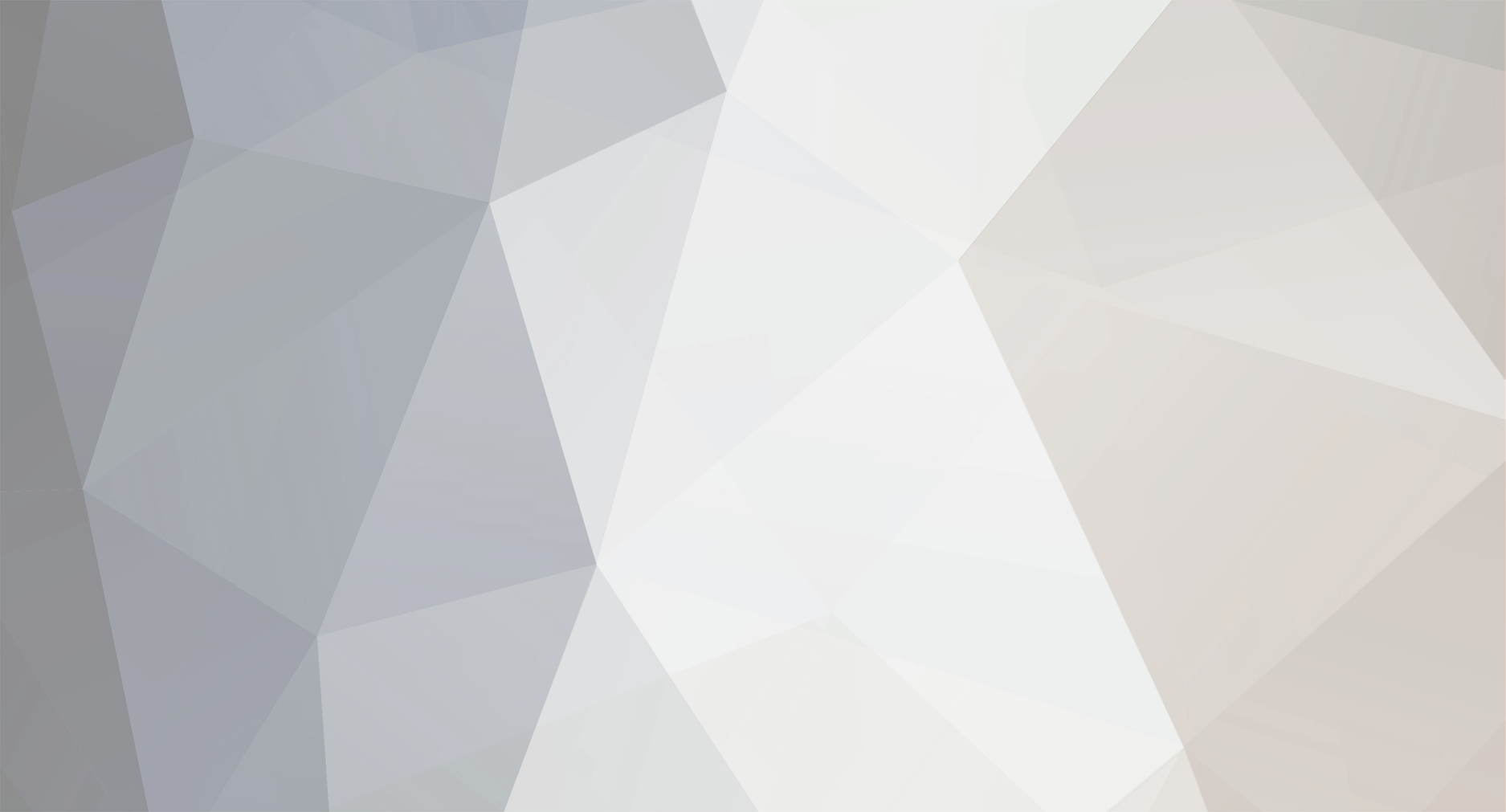
Matenia
-
Posts
2226 -
Joined
-
Last visited
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Posts posted by Matenia
-
-
-
You still haven't understood me. You did exactly what I wanted you to do. 2 separate quests you can pulse (with the same quest id, but different target entries for different mobs).
The only difference is you're using C# to directly checking for objective count on the quest objectives, I suggested you use XML to do so.
I guess I should've posted a longer example to make it more clear. -
What you're doing is essentially the same thing I suggested, except I wanted you to fill in the complete condition in XML, whereas you use C#.
You'll still need 2 pulses for 2 different quests, both of which have a different objective that needs completing. Each quest then has a different target entry set.
-
Look at the XML file it generates. Looks like this and works fine:
<ObjectiveCount1>0</ObjectiveCount1> <ObjectiveCount2>1</ObjectiveCount2> <ObjectiveCount3>0</ObjectiveCount3> <ObjectiveCount4>0</ObjectiveCount4> <ObjectiveCount5>0</ObjectiveCount5> <AutoDetectObjectiveCount1>false</AutoDetectObjectiveCount1> <AutoDetectObjectiveCount2>false</AutoDetectObjectiveCount2> <AutoDetectObjectiveCount3>false</AutoDetectObjectiveCount3> <AutoDetectObjectiveCount4>false</AutoDetectObjectiveCount4> <AutoDetectObjectiveCount5>false</AutoDetectObjectiveCount5>
-
It may say that, but it definitely 100% works. If you set the objective count and set it to autodetect false, it will work. I've been using it on TBC for quite a while.
-
For something like this just get the addon TurnIn and spam click the NPC for a few seconds
-
Create 2 quests with the same id, but different names (so you can pulse both steps), then set the objective count 1 to whatever number the first kill requires in your questlog (8, for example), and on the second quest, you set objective count 2 to 6. Set all other objective counts to 0.
Now you can pulse both steps that each contain different information on where and what to kill.
-
Make your own profiles
-
You're using MoP. The client is a buggy mess for private servers. So just use this plugin to auto-fix it:
using System; using System.Threading; using System.Threading.Tasks; using System.Diagnostics; using System.ComponentModel; using System.Collections.Generic; using System.Collections; using robotManager.Helpful; using robotManager.Products; using wManager.Plugin; using wManager.Wow.Enums; using wManager.Wow.Helpers; using wManager.Wow.ObjectManager; public class Main : IPlugin { private Stopwatch ActionTimer; private bool isRunning; private BackgroundWorker pulseThread; public void Initialize() { isRunning = true; EventsLuaWithArgs.OnEventsLuaWithArgs += Events; EventsLua.AttachEventLua(LuaEventsId.PLAYER_ENTER_COMBAT, m => Event_PLAYER_ENTER_COMBAT()); EventsLua.AttachEventLua(LuaEventsId.PLAYER_LEAVE_COMBAT, m => Event_PLAYER_LEAVE_COMBAT()); EventsLua.AttachEventLua(LuaEventsId.PLAYER_REGEN_ENABLED, m => Event_PLAYER_LEAVE_COMBAT()); Logging.Write("[LuaCombatFix] Init"); ActionTimer = new Stopwatch(); pulseThread = new BackgroundWorker(); pulseThread.DoWork += Pulse; pulseThread.RunWorkerAsync(); } public void Events(LuaEventsId id, List<string> args) { /*for (int i = 0; i < 11; i++) { Logging.Write(id.ToString()); Logging.Write("\nArg" + i + " contains: " + args[i]); }*/ if (id == LuaEventsId.UNIT_SPELLCAST_SENT && args[0] == "player") { Logging.WriteDebug("[LuaCombatFix] Player cast sent: " + args[1]); if (ObjectManager.Me.InCombat) { //Logging.WriteDebug("[LuaCombatFix] Resetting timer with time left: " + ActionTimer.ElapsedMilliseconds); ActionTimer.Reset(); if(!ActionTimer.IsRunning) { ActionTimer.Start(); } } else { ActionTimer.Reset(); ActionTimer.Stop(); } } } public void Pulse(object sender, DoWorkEventArgs args) { try { while (isRunning) { if (!Products.InPause && Products.IsStarted) { Logging.WriteDebug("[LuaCombatFix] Checking Timer with time left: " + ActionTimer.ElapsedMilliseconds); if (ActionTimer.ElapsedMilliseconds >= 8000) { Logging.Write("[LuaCombatFix] Fixing Combat"); ActionTimer.Reset(); Lua.LuaDoString("ReloadUI()"); } } Thread.Sleep(1000); } } catch (Exception ex) { Logging.Write(ex.Message); } } public void Event_PLAYER_ENTER_COMBAT() { Logging.WriteDebug("[LuaCombatFix] Player entering combat with time left: " +ActionTimer.ElapsedMilliseconds); ActionTimer.Reset(); ActionTimer.Start(); } public void Event_PLAYER_LEAVE_COMBAT() { Logging.WriteDebug("[LuaCombatFix] Player leaving combat with time left: " + ActionTimer.ElapsedMilliseconds); ActionTimer.Reset(); ActionTimer.Stop(); } public void Dispose() { try { isRunning = false; Logging.Write("[LuaCombatFix] Stopped"); } catch (Exception ex) { Logging.Write(ex.Message); } } public void Settings() { Logging.Write("[LuaCombatFix] No settings available"); } }
-
I already have all that, since I write my own fightclasses (including targeting). So I guess I could easily load my fightclasses as a plugin instead, to reduce load and then just delete wRotation.dll? Usually my bots are never sitting above 5% CPU load anyway, but sometimes after running it all day, it really starts building up, especially if I do a lot of shit in BGs.
-
2 hours ago, forerun said:
Genarlly a biggest hog thing in wrobot is wrotatnion ^^. If you write rotation as a plugin (c# only ) and completely remove wrotation.dll file the wrobot load will be 1% max :) 3 x panda wow + 3 x wrobots with rotation autofollow scanning around all mobs etc on old athlon x2 250 and all is working quite well here. Wrotation is a hog ;)
To clarify, you just use your own targeting logic and then basically just call your own C# fightclass yourself?
-
You can use C#
Lua.RunMacroText("/cast Chain Heal(Rank1)");
Or Lua directly
CastSpellByName("Chain Heal(Rank1)")
-
The trial is only for private servers and should work. However, I figured that something may have corrupted the your install (maybe faulty RAM), which could be your problem.
That's why I suggested a complete pre-installed client download (try the one Excalibur supplies).
-
Try to download a different pre-installed client. The Feenix one is modified, for example.
-
Start both WoW and the bot with admin privileges
-
Those things would be great to have.
-
Make your own profile, don't use the default
-
That is likely because you're calling it several times a second, instead of only once. If you use it over and over, of course it'll stutter. So use it only once. Play around with the 800ms timer instead.
-
23 hours ago, lonellywolf said:
I am having this exact problem and can't figure out how to make the character to move backward smoothly.
I literally posted here, what OP asked for. He said he'd like to do MoveBackwardStart() C_Timer.After(200, function() MoveBackwardStop() end).
I posted everything required to call that EXACT code. The only exception being that I would stop backwardsmoving after 800 milliseconds, not 200. -
4 hours ago, Droidz said:
If you can wait next update, I added OnEventsLuaWithArgs in vanilla and TBC, and I fixed problem with missing events.
you're the best!
-
Try deleting your saved tiles/paths. I'm not sure it'll help, but potentially could.
-
For BGs, you need to disable "queue only if party leader".
-
It's possible, but the battlegrounder follows enemies and tries to kill them. So you need a fightclass that priotizes healing units around you while still able to damage enemies.
I have 2 such fightclasses on here and am selling a paid version of the resto shaman as well.
-
1) sounds like your fightclass is bugged/bad
2) just repair the bot, it seems it's utterly broken
How can I train skills every 4 lel ?
in WRobot for Wow Vanilla - Help and support
Posted
Answer is on the forums. You can also modify the levels in one of the TrainLevel plugins here.
If you add your trainer in SW and set your bot to use flightmasters, it will choose them to make the route quicker.