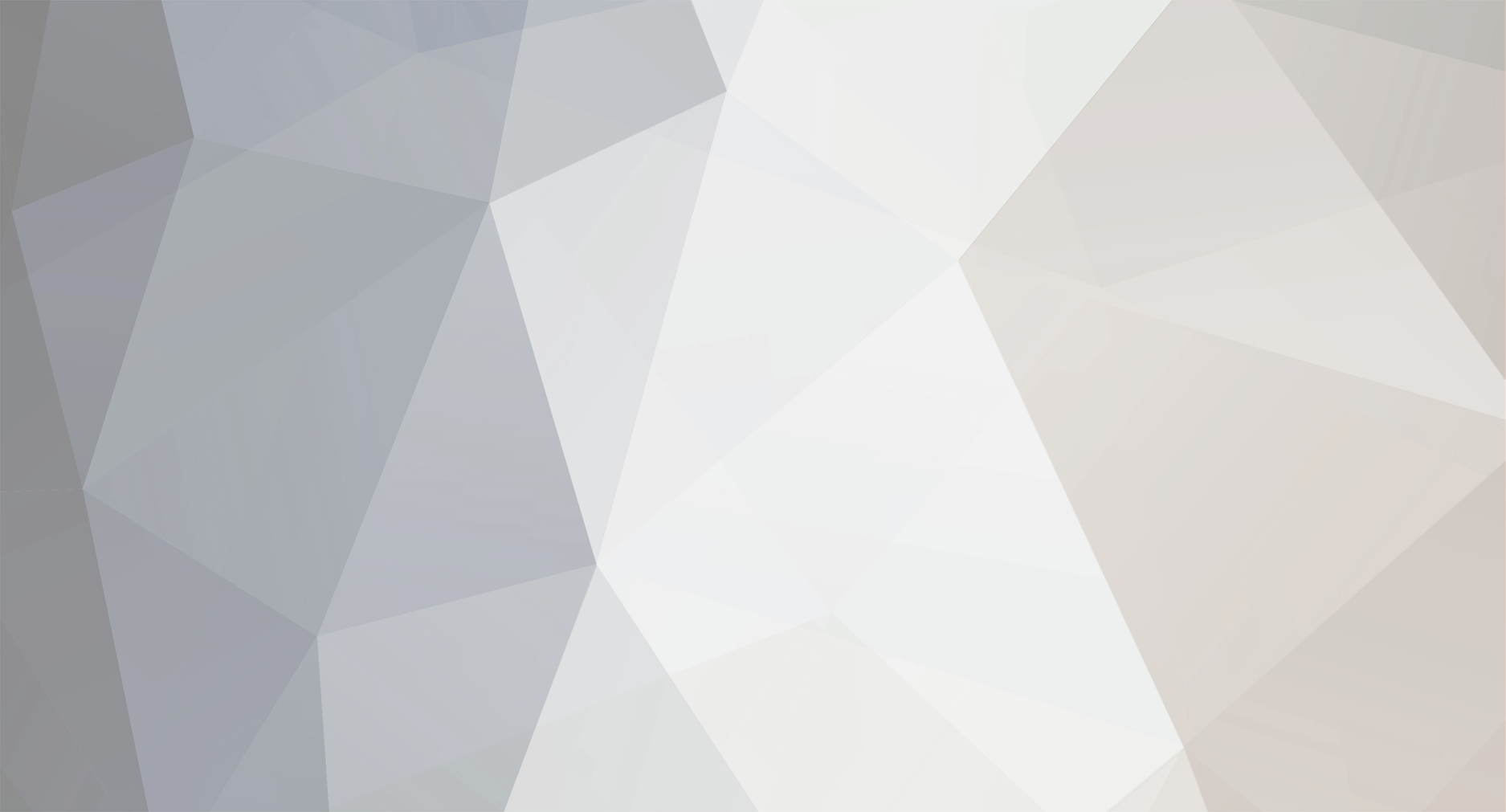
Pasterke
-
Posts
165 -
Joined
-
Last visited
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Posts posted by Pasterke
-
-
proving grounds or LFR. Don't test in dungeons !
-
Oui, il faut C# pour une fightclass heal.
Mais vous avez ici un example. Ca marche tres bien pour druid restoration. Une fois que vous avez la mouture, c'est pas tres difficile pour l'adopter a une autre class heal.
-
Ainsi joint la fightclass que j'utilise pour le moment en solution vs2015.
Sorry, c'est celle la que j'utilise :
-
using System.Configuration; using System.IO; using System.Linq; using System.Threading; using System.Xml; using robotManager; using robotManager.FiniteStateMachine; using robotManager.Helpful; using wManager.Wow.Class; using wManager.Wow.Helpers; using wManager.Wow.ObjectManager; using wManager.Wow.Bot.States; using Timer = robotManager.Helpful.Timer; using wManager.Wow.Enums; using MemoryRobot; public class Main : ICustomClass { public float Range { get { return 40; } } private RestoDruid _RestoDruid; public void Initialize() { _RestoDruid = new RestoDruid(); _RestoDruid.Pulse(); } public void Dispose() { if (_RestoDruid != null) { _RestoDruid.Stop(); } } public void ShowConfiguration() { MessageBox.Show("No Configuration for this Routine"); } } public class RestoDruid { private bool _isLaunched; public bool IsLaunched { get { return _isLaunched; } set { _isLaunched = value; } } public void Pulse() { _isLaunched = true; var thread = new Thread(RoutineThread) { Name = "RestoDruid_FightClass" }; thread.Start(); } public void Stop() { _isLaunched = false; Logging.WriteFight("Stop 'RestoDruid'"); } void RoutineThread() { Logging.WriteFight("'RestoDruid' Started"); while (_isLaunched) { Routine(); Thread.Sleep(10); // Temps d'attante pour éviter d'utiliser trop le processeurs } Logging.WriteFight("'RestoDruid' Stopped"); } public uint Healthstone = 5512; public uint AncientRejuvenationPotion = 127836; public uint AncientManaPotion = 188303; void Routine() { if (ObjectManager.Me.IsMounted) return; if (ObjectManager.Me.IsCast) return; if (ObjectManager.Me.HealthPercent <= 45 && needToUseItem(Healthstone)) return; if (ObjectManager.Me.ManaPercentage <= 30 && needToUseItem(AncientManaPotion)) return; if (needWildGrowth()) return; if (needHealingTouch()) return; return; } private Spell _healingTouch; public RestoDruid() { _healingTouch = new Spell("Healing Touch"); Logging.WriteFight("'RestoDruid' by Pasterke loaded"); } public bool needHealingTouch() { if (!CanUseSpell(_healingTouch)) return false; if (getPartyMembers().Count() > 0) { try { var t = getPartyMembers().Where(p => p.IsAlive && p.GetDistance <= 40 && !TraceLine.TraceLineGo(p.Position) && p.HealthPercent <= RestoSettings.CurrentSetting.HealingTouch) .OrderBy(p => p.HealthPercent).FirstOrDefault(); if (t != null && !ObjectManager.Me.GetMove) { SpellManager.CastSpellByNameOn(_healingTouch.Name, t.Name); Logging.WriteFight(_healingTouch.Name + " on " + t.Name); return true; } } catch (Exception e) { Logging.WriteDebug(e.Message); } } return false; } public bool needWildGrowth() { if (!CanUseSpell(_wildGrowth)) return false; if (getPartyMembers().Count() > 0) { try { WoWUnit unit = new WoWUnit(0); var t = getPartyMembers().Where(p => p.IsAlive && p.GetDistance <= 40 && !TraceLine.TraceLineGo(p.Position) && p.HealthPercent <= RestoSettings.CurrentSetting.WildGrowth).OrderBy(p => p.HealthPercent).ToList(); if (t.Count() > 0) { int count = 0; int tel = 0; Vector3 mainPos = t.FirstOrDefault().Position; unit = new WoWUnit(t.FirstOrDefault().GetBaseAddress); //Logging.WriteFight(unit.Name); count = t.Count(p => p.IsAlive && p.Position.DistanceTo(mainPos) <= 30 && p.HealthPercent <= RestoSettings.CurrentSetting.WildGrowth); //Logging.WriteFight("count players(t): " + count + " aantal leden: " + getPartyMembers().Count()); if (getPartyMembers().Count() > 5) { tel = 5; } else { tel = 3; } //Logging.WriteFight("tel variable: " + tel); if (count >= tel && !ObjectManager.Me.GetMove) { SpellManager.CastSpellByNameOn(_wildGrowth.Name, unit.Name); Logging.WriteFight("Casting: " + _wildGrowth.Name + " on: " + unit.Name); return true; } } } catch (Exception e) { Logging.WriteDebug(e.Message); } } return false; } bool CanUseSpell(Spell spell) { try { if (!spell.KnownSpell) return false; if (SpellManager.GetSpellCooldownTimeLeft(spell.Name) > 0) { return false; } return true; } catch (Exception e) { Logging.WriteDebug(e.Message); } return false; } public bool debuffExists(string debuff) { return (ObjectManager.Me.Guid == ObjectManager.Target.BuffCastedBy(debuff)); } public bool MeIsInParty { get { return getPartyMembers().Count() > 0 ? true : false; } } public bool myBuffExists(string buff, WoWUnit unit) { return unit.BuffCastedByAll(buff).Contains(ObjectManager.Me.Guid); } public bool hasMyHot(WoWUnit unit) { return unit.BuffCastedByAll("Regrowth").Contains(ObjectManager.Me.Guid) || unit.BuffCastedByAll("Rejuvenation").Contains(ObjectManager.Me.Guid) || unit.BuffCastedByAll("Wild Growth").Contains(ObjectManager.Me.Guid); } public IEnumerable<WoWUnit> getPartyMembers() { var units = new List<WoWUnit>(); var partyMembers = Party.GetPartyGUIDHomeAndInstance(); if (partyMembers.Any()) { foreach (Int128 u in partyMembers) { var un = new WoWUnit(ObjectManager.GetObjectByGuid(u).GetBaseAddress); units.Add(un); } units.Add(ObjectManager.Me); } return units; } public string GetTankPlayerName() { if (Usefuls.MapZoneName == "Proving Grounds") return "Oto the Protector"; var lua = new[] { "partyTank = \"\";", "for groupindex = 1,MAX_PARTY_MEMBERS do", " if (UnitInParty(\"party\" .. groupindex)) then", " local role = UnitGroupRolesAssigned(\"party\" .. groupindex);", " if role == \"TANK\" then", " local name, realm = UnitName(\"party\" .. groupindex);", " partyTank = name;", " return;", " end", " end", "end", }; return Lua.LuaDoString(lua, "partyTank"); } public bool needToUseItem(uint itemEntry) { if (!ItemsManager.HasItemById(itemEntry)) return false; if (Bag.GetContainerItemCooldown((int)itemEntry) > 0) return false; try { Lua.LuaDoString("local name = GetItemInfo(" + itemEntry + "); RunMacroText('/use ' .. name);"); } catch (Exception e) { Logging.WriteDebug("Use Item => " + e.Message); } return true; } public WoWUnit GetPartyTank() { if (getPartyMembers().Count() > 0) { try { var p = new WoWUnit(0); var playerName = GetTankPlayerName(); if (!string.IsNullOrWhiteSpace(playerName)) { playerName = playerName.ToLower().Trim(); var party = getPartyMembers(); foreach (var woWPlayer in party) { if (woWPlayer.Name.ToLower() == playerName) { return p = new WoWUnit(woWPlayer.GetBaseAddress); } } } } catch (Exception e) { Logging.WriteDebug(e.Message); } } return null; } }
Example comment coder une routine avec C#.
-
Make sure you have no ancient mana before starting gathering. Buy buff for 50 ancient mana and repeat until you have no more ancient mana, or the quantity you want.
-
Still try to getting it in place for legion.
-
Uploaded an new version :)
-
Ok, saw it, before last update it worked. Will recompile it.
-
If you use my combatlooter plugin, don't use it when you want to skin. It's not supported. The main goal was to loot mobs, not skin.
-
use auto vendor addon
-
3 hours ago, Droidz said:
Hello, if you can wait next update and tell me if problem is resolved.
It's ok now after update :)
-
47 minutes ago, eeny said:
If this is the first of many ID changes... im going to be mad.. or very busy
That's the point, the ID's are not changed in game, just wrobot return the wrong id's.
-
[D] 09:43:25 - [Spell] Healing Touch (Id found: 5185, Name found: Healing Touch, NameInGame found: Healing Touch, Know = True, IsSpellUsable = True)
[D] 09:43:25 - [Spell] Rejuvenation (Id found: 224526, Name found: Rejuvenation, NameInGame found: Rejuvenation, Know = False, IsSpellUsable = True) ???????????????????????????????????????????????????
[D] 09:43:25 - [Spell] Regrowth (Id found: 224527, Name found: Regrowth, NameInGame found: Regrowth, Know = False, IsSpellUsable = True) ?????????????????????????????????????????????????????ID's are wrong should be : 774 and 8936
[D] 09:43:25 - [Spell] Efflorescence (Id found: 145205, Name found: Efflorescence, NameInGame found: Efflorescence, Know = True, IsSpellUsable = True)
[D] 09:43:25 - [Spell] Wild Growth (Id found: 48438, Name found: Wild Growth, NameInGame found: Wild Growth, Know = True, IsSpellUsable = True)
[D] 09:43:25 - [Spell] Swiftmend (Id found: 18562, Name found: Swiftmend, NameInGame found: Swiftmend, Know = True, IsSpellUsable = True)
[D] 09:43:25 - [Spell] Flourish (Id found: 197721, Name found: Flourish, NameInGame found: Flourish, Know = True, IsSpellUsable = True)
[D] 09:43:25 - [Spell] Ironbark (Id found: 102342, Name found: Ironbark, NameInGame found: Ironbark, Know = True, IsSpellUsable = True)
[D] 09:43:25 - [Spell] Lifebloom (Id found: 33763, Name found: Lifebloom, NameInGame found: Lifebloom, Know = True, IsSpellUsable = True)
[D] 09:43:25 - [Spell] Barkskin (Id found: 22812, Name found: Barkskin, NameInGame found: Barkskin, Know = True, IsSpellUsable = True)
[D] 09:43:25 - [Spell] Innervate (Id found: 29166, Name found: Innervate, NameInGame found: Innervate, Know = True, IsSpellUsable = True)
[D] 09:43:25 - [Spell] Rebirth (Id found: 20484, Name found: Rebirth, NameInGame found: Rebirth, Know = True, IsSpellUsable = True)
[D] 09:43:25 - [Spell] Moonfire (Id found: 8921, Name found: Moonfire, NameInGame found: Moonfire, Know = True, IsSpellUsable = True)
[D] 09:43:25 - [Spell] Sunfire (Id found: 93402, Name found: Sunfire, NameInGame found: Sunfire, Know = True, IsSpellUsable = True)
[D] 09:43:25 - [Spell] Solar Wrath (Id found: 5176, Name found: Solar Wrath, NameInGame found: Solar Wrath, Know = True, IsSpellUsable = True)Realy annoying
-
If you use the flight class creator, you can add the condition IsSpellOverlayed. Set Healing Touch and true.
-
private Spell healingTouch = new Spell("Healing Touch");
If(healingTouch.IsSpellOverlayed) {....} //because healing touch spell on your castbar light up when you have Predatory Swiftness proc.
-
You don't have paid routines here. You make your own with the fightclass creator. Easy to do if you know how to play your class. It's already 1 year I use this bot, and for now no bans anymore. I was also an HB user, but became sick of the banwaves and to start over again and again.
-
the old fashion way, with a goto.
-
You can't create an healing routine with the FightClass Creator. You need to code it in C#.
-
Most of my hair is already grey, and iI think the rest is also become grey while coding an druid restoration fightclass.
I always got null references errors when I started the fightclass. The evil one was Efflorescence after debugging alot.
You have to check if you have a pet with the name Efflorescence and not for a wowobject, because the mushroom you plant with Efflorescence is seen by wrobot as an pet :)
Another bug : wrobot don't see if you are in combat or not. Fight.InFight or ObjectManager.Me.Incombat always return false.
-
private Spell catForm = new Spell("Cat Form");
If (!ObjectManager.Me.HaveBuff("Cat Form") && Fight.InFight) { catForm.launch(); }
-
Sometimes it's difficult to check debuffs/buffs before casting a spell.
Example : Druid Guardian, if you have Faerie Swarm talent, you need to cast Faerie Fire; because Faerie Swarm is unknown spell. If you need to check if debuff faerie swarm exists, then you have a problem, for those who don't have Faerie Swarm.
To check the debuff I use following C# code :
public bool debuffExixts(string debuff) { /*[F] 11:09:45 - check Aura: Growl: ID=6795, Stack=0, TimeLeft=1082 ms, Owner=00000000079D3B1E0834FC0000000000*/ List<Aura> t = ObjectManager.Target.GetAllBuff(); foreach (var aura in t) { string[] checkAura = aura.ToString().Split(':'); //Logging.WriteFight("check Aura: " + checkAura[0].Trim()); if (ObjectManager.Me.Guid == ObjectManager.Target.BuffCastedBy(checkAura[0].Trim()) && checkAura[0].Contains(debuff)) return true; } return false; }
Faerie Fire and Faerie Swarm have 1 common text, Faerie. Then you can use debuffExists("Faerie") to check.
- Droidz and BetterSister
-
2
-
This is only possible with c# code. Set your current position and add 5 yards, then cast freezing trap at that postion. Easy stuff.
-
This is a base Framework in C# to develop a fightclass.
Everything is named Test, just rename Test to Disc, Warrior etc ....
I put some comments where needed.
Imoportant : CastSpellOn can't be used on unfriendly targets because the protected status of the WoW API !
Hope this is usefull :)
-
Here some addons that I use. Feel free to use the ones you like.
DBM-v4-r188-BC-Vanilla-Mods.zip
ShadowedUnitFrames-v3.2.12.zip
DK Debuff Bug since update
in WRobot for Wow Wrath of the Lich King - Help and support
Posted
Try that :
public static bool myDebuffExists(String debuff) { var auras = ObjectManager.Target.GetAllBuff(); foreach(var a in auras) { string aura = a.ToString(); if (aura.Contains(debuff) && (aura.Contains(ObjectManager.Me.Guid.ToString()) || aura.Contains(ObjectManager.Me.Guid128.ToString()))) return true; } return false; }