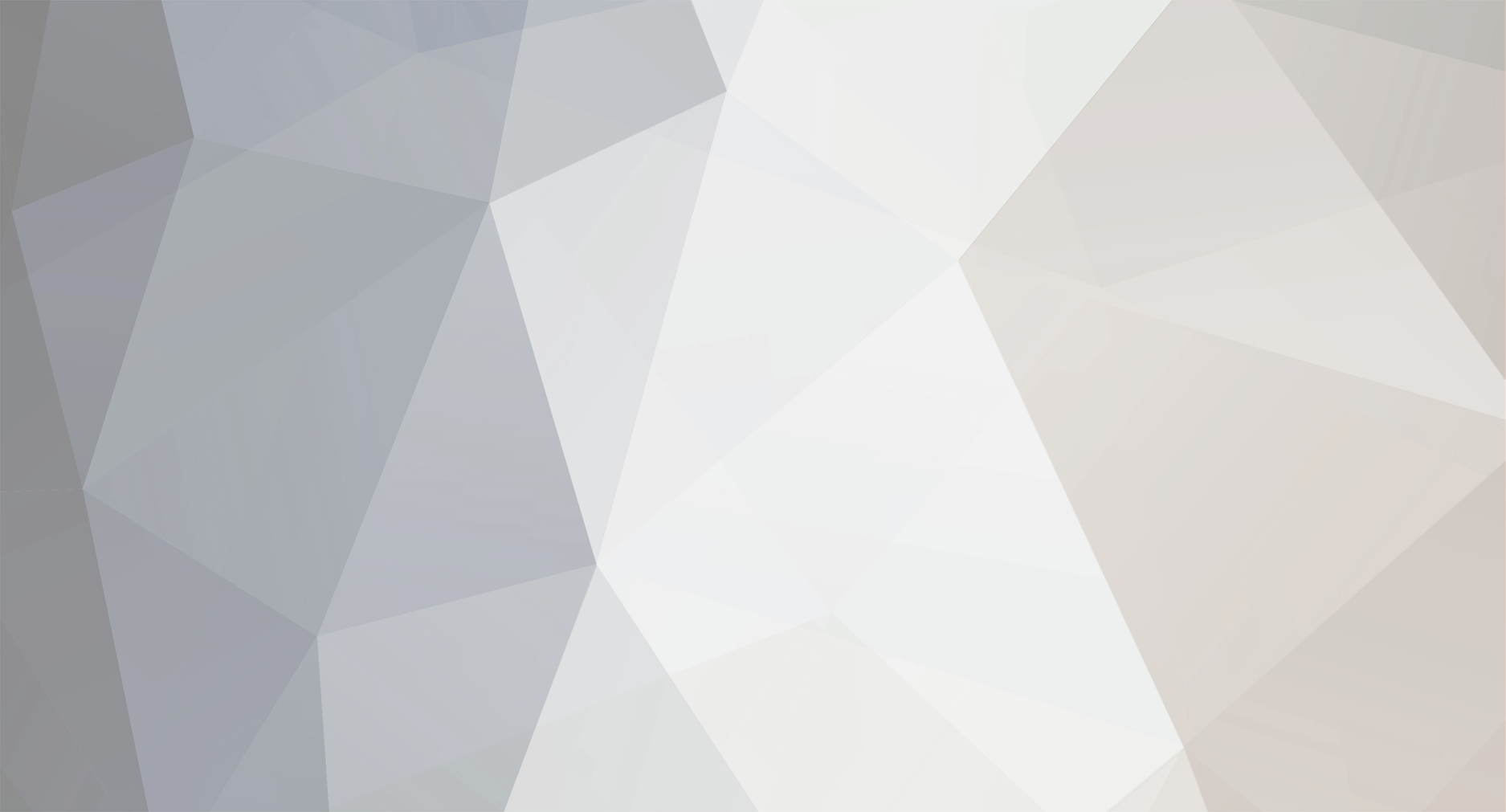
Matenia
Elite user-
Posts
2232 -
Joined
-
Last visited
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Everything posted by Matenia
-
Take a look at the free quester for alliance poisons, it has the solution by calling autoloot in memory.
-
Create follow path in quester profile with CShape
Matenia replied to 79135's topic in General discussion
//bad pseudo code: bool reachedP1 = false; if(!reachedP1) { wManager.Wow.Bot.Tasks.GoToTask.ToPosition(p1); } //p1 is reached here if(ObjectManager.Me.Position.DistanceTo(p1) < 5) { reachedP1 = true; } wManager.Wow.Bot.Tasks.GoToTask.ToPosition(p2); wManager.Wow.Bot.Tasks.GoToTask.ToPosition(p...); wManager.Wow.Bot.Tasks.GoToTask.ToPosition(pN); But keep in mind, you have to do this in full C# quest. NOT just overrideCSharpPulse! Or else it will go to P1 over and over, because everytime it sets reachedP1 to false in the start. If you want to use this in OverrideCSharpPulse, you have to use Var API, like here: Instead of just using boolean you will do if(Var.GetVar<bool>("reachedP1") == null) { Var.SetVar("reachedP1", false); } if(!Var.GetVar<bool>("reachedP1")) { GoToTask.ToPos(p1); //if close code Var.SetVar("reachedP1", true); } It's all a bit pseudo code but you get the idea -
This is correct. I read Lua events and build my own timer with DateTime instances updated on events
-
Either set loot threshold to epic with party loot enabled or find an addon that auto greeds. No plugin for that.
-
Okay, I guess you've misunderstood. PartyHelper.dll is the plugin which you run in Party mode or wRotation on the "follower" (the main also has to run something). That's not what I was pointing towards. There is a RestoShamVanilla.dll in there, which is a resto shaman fightclass for vanilla. If you want to modify it, I threw in the C# source as RestoShamVanilla.zip (entire Visual Studio project) there. However, if you don't know what you're doing, this isn't gonna of much use for you expect that it's a working fightclass and you don't have to make your own.
-
C# restosham fightclass with source code attached
-
PVP plugins - track enemy players for example
Matenia replied to vanbotter's topic in General discussion
foreach (WoWUnit Mob in ObjectManager.GetWoWUnitAttackables().Where(x => x.Type == WoWObjectType.Player && x.GetDistance2D < AggroMonitorSettings.CurrentSetting.SearchRange && x.TargetObject.Name != AggroMonitorSettings.CurrentSetting.Tank1 && x.TargetObject.Name != AggroMonitorSettings.CurrentSetting.Tank2 && x.TargetObject.Name != AggroMonitorSettings.CurrentSetting.Tank3 && x.TargetObject.Name != ObjectManager.Me.Name && ObjectManager.Target.TargetObject != null)) This filters it to just players, if you modify AggroMonitor -
private static Predicate<Npc> _dbFilter = n => n.CurrentProfileNpc && (n.Type == Npc.NpcType.Vendor || n.Type == Npc.NpcType.Repair); public static void Start() { FiniteStateMachineEvents.OnBeforeCheckIfNeedToRunState += VendorHandler; } private static void VendorHandler(Engine engine, State state, CancelEventArgs cancelable) { if(state != null && state.GetType() == typeof(ToTown) && new ToTown().NeedToRun) { NpcDB.AcceptOnlyProfileNpc = true; NpcDB.ListNpc.RemoveAll(_dbFilter); Npc vendor = FindVendor(); //this is your own function that returns an object of type NPC if (vendor != null) { NpcDB.AddNpc(vendor, false, true); } } }
-
Gonna dump some code here I've used to experiment, you can adjust it for your own needs: public class SmartPulls { private static WoWLocalPlayer Me = ObjectManager.Me; private static int _checkDistance = 22; private static int _frontDistance = 15; public static void Start() { if (PluginSettings.CurrentSetting.SmartPulls) { FightEvents.OnFightStart += FightValidityCheck; Radar3D.OnDrawEvent += Radar3DOnOnDrawEvent; } } private static void Radar3DOnOnDrawEvent() { if (Radar3D.IsLaunched) { Vector3 rightPlayerVector = Helper.CalculatePosition(Me.Position, -Helper.Atan2Rotation(Me.Position, Me.Position, Me.Rotation), -_checkDistance); Vector3 leftPlayerVector = Helper.CalculatePosition(Me.Position, -Helper.Atan2Rotation(Me.Position, Me.Position, Me.Rotation), _checkDistance); Vector3 rightTargetVector = Helper.CalculatePosition(rightPlayerVector, -Helper.Atan2Rotation(Me.Position, rightPlayerVector, 0), _checkDistance + _frontDistance); Vector3 leftTargetVector = Helper.CalculatePosition(leftPlayerVector, -Helper.Atan2Rotation(Me.Position, leftPlayerVector, 0), -(_checkDistance + _frontDistance)); Radar3D.DrawCircle(rightPlayerVector, 2.5f, Color.Blue); Radar3D.DrawCircle(leftPlayerVector, 2.5f, Color.Green); Radar3D.DrawCircle(rightTargetVector, 2.5f, Color.Yellow); Radar3D.DrawCircle(leftTargetVector, 2.5f, Color.DarkRed); Radar3D.DrawLine(rightPlayerVector, leftPlayerVector, Color.Gold); Radar3D.DrawLine(leftPlayerVector, leftTargetVector, Color.Gold); Radar3D.DrawLine(leftTargetVector, rightTargetVector, Color.Gold); Radar3D.DrawLine(rightTargetVector, rightPlayerVector, Color.Gold); } } public static void Stop() { FightEvents.OnFightStart -= FightValidityCheck; Radar3D.OnDrawEvent -= Radar3DOnOnDrawEvent; } public static void Pulse() { if (PluginSettings.CurrentSetting.SmartPulls && !Escape.IsEscaping && Me.Target > 0 && !Fight.InFight && !Me.InCombatFlagOnly && Logging.Status != "Regeneration" && !Me.HaveBuff("Resurrection Sickness")) { Vector3 rightPlayerVector = Helper.CalculatePosition(Me.Position, -Helper.Atan2Rotation(Me.Position, Me.Position, Me.Rotation), -_checkDistance); Vector3 leftPlayerVector = Helper.CalculatePosition(Me.Position, -Helper.Atan2Rotation(Me.Position, Me.Position, Me.Rotation), _checkDistance); Vector3 rightTargetVector = Helper.CalculatePosition(rightPlayerVector, -Helper.Atan2Rotation(Me.Position, rightPlayerVector, 0), _checkDistance + _frontDistance); Vector3 leftTargetVector = Helper.CalculatePosition(leftPlayerVector, -Helper.Atan2Rotation(Me.Position, leftPlayerVector, 0), -(_checkDistance + _frontDistance)); List<Vector3> boundBox = new List<Vector3> { rightPlayerVector, leftPlayerVector, rightTargetVector, leftTargetVector }; WoWUnit unit = ObjectManager.GetObjectWoWUnit() .Where(o => o.IsAlive && o.Reaction == Reaction.Hostile && !o.IsPet && !o.IsPlayer() && o.Guid != Me.Target && (o.Level >= Me.Level ? o.Level - Me.Level <= 3 : Me.Level - o.Level <= 6) && VectorHelper.PointInPolygon2D(boundBox, o.Position) && !TraceLine.TraceLineGo(Me.Position, o.Position, CGWorldFrameHitFlags.HitTestSpellLoS) && !wManagerSetting.IsBlackListed(o.Guid)) .OrderBy(o => o.GetDistance) .FirstOrDefault(); if (unit != null) { PluginLog.Log($"{unit.Name} is in our way, attacking!"); MovementManager.StopMoveTo(false, 500); ObjectManager.Me.Target = unit.Guid; Fight.StartFight(unit.Guid); ObjectManager.Me.Target = unit.Guid; } } } //don't pull another target if we are already in combat with anyone (e.g. body pulled mobs) private static void FightValidityCheck(WoWUnit unit, CancelEventArgs cancelable) { if (Escape.IsEscaping || ObjectManager.GetObjectWoWUnit().Count(u => u.IsTargetingMeOrMyPet && u.Reaction == Reaction.Hostile) > 0) { return; } if (CheckForGroup(unit, cancelable)) { MovementManager.StopMoveTo(false, 500); cancelable.Cancel = true; return; } if (CheckForClearPath(unit, cancelable)) { MovementManager.StopMoveTo(false, 500); cancelable.Cancel = true; return; } } private static bool CheckForGroup(WoWUnit unit, CancelEventArgs cancelable) { // group too large, nothing we can do, stop fight and go away int addAmount = ObjectManager.GetObjectWoWUnit().Count(o => o.IsAlive && o.Reaction == Reaction.Hostile && o.Guid != unit.Guid && o.Position.DistanceTo(unit.Position) <= 12 && !o.IsTargetingMeOrMyPetOrPartyMember); if (addAmount > 1) { wManagerSetting.AddBlackList(unit.Guid, 60000); Fight.StopFight(); Lua.LuaDoString("ClearTarget()"); wManagerSetting.AddBlackListZone(unit.Position, 30 + addAmount * 5); PathFinder.ReportBigDangerArea(unit.Position, 30 + addAmount * 5); PluginLog.Log("Stopping pull on large group!"); return true; } return false; } private static bool CheckForClearPath(WoWUnit unit, CancelEventArgs cancelable) { if (Me.InCombatFlagOnly) { return false; } Vector3 rightPlayerVector = Helper.CalculatePosition(Me.Position, -Helper.Atan2Rotation(unit.Position, Me.Position, 0), _checkDistance); Vector3 leftPlayerVector = Helper.CalculatePosition(Me.Position, -Helper.Atan2Rotation(unit.Position, Me.Position, 0), -_checkDistance); Vector3 rightTargetVector = Helper.CalculatePosition(unit.Position, -Helper.Atan2Rotation(Me.Position, unit.Position, 0), -_checkDistance); Vector3 leftTargetVector = Helper.CalculatePosition(unit.Position, -Helper.Atan2Rotation(Me.Position, unit.Position, 0), _checkDistance); List<Vector3> boundingBox = new List<Vector3>{rightPlayerVector, leftPlayerVector, rightTargetVector, leftTargetVector}; if (ObjectManager.GetObjectWoWUnit().FirstOrDefault(o => o.IsAlive && o.Reaction == Reaction.Hostile && o.Guid != unit.Guid && VectorHelper.PointInPolygon2D(boundingBox, o.Position)) != null) { WoWUnit newEnemy = ObjectManager.GetObjectWoWUnit() .Where(o => o.IsAlive && o.Reaction == Reaction.Hostile && o.SummonedBy <= 0 && !o.IsPlayer() && o.Guid != unit.Guid && !wManagerSetting.IsBlackListed(o.Guid) && !wManagerSetting.IsBlackListedNpcEntry(o.Entry)) .OrderBy(o => o.GetDistance) .FirstOrDefault(); if (newEnemy != null) { wManagerSetting.AddBlackList(unit.Guid, 60000); Fight.StopFight(); Fight.StartFight(newEnemy.Guid); PluginLog.Log($"Enemy in the way, can't pull here - pulling {newEnemy.Name} instead"); } return true; } return false; } }
-
-
It adds bandages automatically (turn off first aid, if you don't want that feature). As for bags, yes I add those too, but I remove them when you get bigger bags. I don't save the modified settings though, so you're likely running something that saves settings explicitly (like a quester profile, another plugin, etc). So they shouldn't show up twice in the list or even be visible at all. I'll release an update tomorrow where it won't add bags to the list at all. It's just gonna be unfortunate if you loot a new bag, don't have enough space to equip it and then sell it.
-
-
-
Someone recorded one bot and forwarded it to the GMs, they had video proof and banned whenever.
-
Can also be modified to use Regeneration in Battlegrounder. For things like vanishing in combat and staying in stealth to regen you need C# to subscribe to fight events and block the bot's main thread to stop it from engaging in combat (really advanced - better start slow).
-
You can use: Lua.LuaDoString("SitOrStand()"); //newer wow versions Lua.LuaDoString("SitStandOrDescendStart()"); //alternative approach Lua.LuaDoString("DoEmote('SIT')"); //if you wanna stand up specifically Lua.LuaDoString("DoEmote('STAND')");
-
while(!Conditions.IsAttackedAndCannotIgnore && ObjectManager.Me.HealthPercent < wManager.wManagerSetting.CurrentSetting.FoodMaxPercent) { Thread.Sleep(500); }
-
Warden.txt is (afaik) created by oGasai (don't worry, you can say the name). It's also the only software I can think of that gets injected into the client. However, afaik it shouldn't list scanned addresses. Maybe I'm wrong, I haven't used it in ages. It should default all warden scans and protect from them, so I doubt it's protected. If that's not what you were using, disregard this.
-
Avvi's C# Tips & Tricks with Helpful Code Snippets
Matenia replied to Avvi's topic in Developers assistance
Everything after run is regular Lua, so if you want to run your macro like that, just do (Vanilla has no RunMacroText, so it would never work anyway - but more importantly, you should use an IDE to edit your code so you can see things like trying to put 4 quotation marks won't work, because your string ends at ( and then inbetween the next ")" it tries to execute Macro2 as code. See Strings.) Lua.LuaDoString("RunMacro('Macro2')"); -
https://superuser.com/questions/1004697/bind-application-to-a-specific-network-interface I'm not sure if you can use the Relogger.exe to call a .cmd instead of another .exe, but this should essentially do that. You just call ForceBindIP with the correct parameters to automatically inject into WoW.
-
I would assume (I haven't tried, because I've yet to be banned except once on a single character): - use ForceBindIP to bind one instance of ProxyCap and one WoW.exe to the same Virtual Network Adapter each - set each ProxyCap to run a different proxy - set each ProxyCap to re-route a difference WoW.exe by naming them numerically Which part is causing issues?
-
You can probably modify this to your liking. If you want something completely automated, you'll have to purchase
-
The problem isn't pathing, it's that wrobot wants to make a path exactly to them and there is none because they stand behind a counter in the inn. So you need to edit their coordinates slightly to move them "outside" of that. Either in your database or grinder/quester. This won't work with hmp because hmp has its own database (which you can edit with dbeaver because it's SQLite)
-
Based on the virtual machine thing - if they have a way to send a broadcast message to your entire LAN through wow and see which other clients receive it, it should be possible to run this all without a VM just by setting up virtual network adapters. I'm not getting banned, so if someone else can test that theory, you might have a solution that's a bit complicated but no resource hog
-
Version 1.0.0
447 downloads
No refunds - you are purchasing a digital copy of a product. For questions and bugreports, please reach out to me on Discord. Disclaimer: This fightclass only works with the English client. It is possible, that I will add support for more later. The attached file is a max level Sub Rogue fightclass for farming. Rogues have a lot of abilities you can integrate into a bot in a smart way. Doing so for every ability while not looking obviously like a bot isn't an easy task. This fightclass will hopefully fulfill all your needs. I highly recommend getting Poisons and creating Instant Poison. The fightclass will use this automatically. Dynamic Rotation Based Upon Level Uses Backstab/Ambush/Garrote at lower levels and Cheap Shot at higher levels Can be set to always range pull Situational Spell Usage Will range pull if many enemies are around the target (Throw, Shoot, etc) Pick Pockets Humanoids (yes, it loots too) Evasion/Blade Flurry on multiple enemies Vanish and regen in stealth if you are low HP without Evasion available Automatic Skill Detection Automatically detects if you learn new spells while leveling, no need to restart the bot No need for anything on your bars either, it will swap spells out itself PURCHASE NOW - 6.50€ I, the owner and creator of this file, am in no way associated with the wRobot company. By purchasing this file, you agree to the contract of the purchasing website and that alone. Check out my other Fightclasses