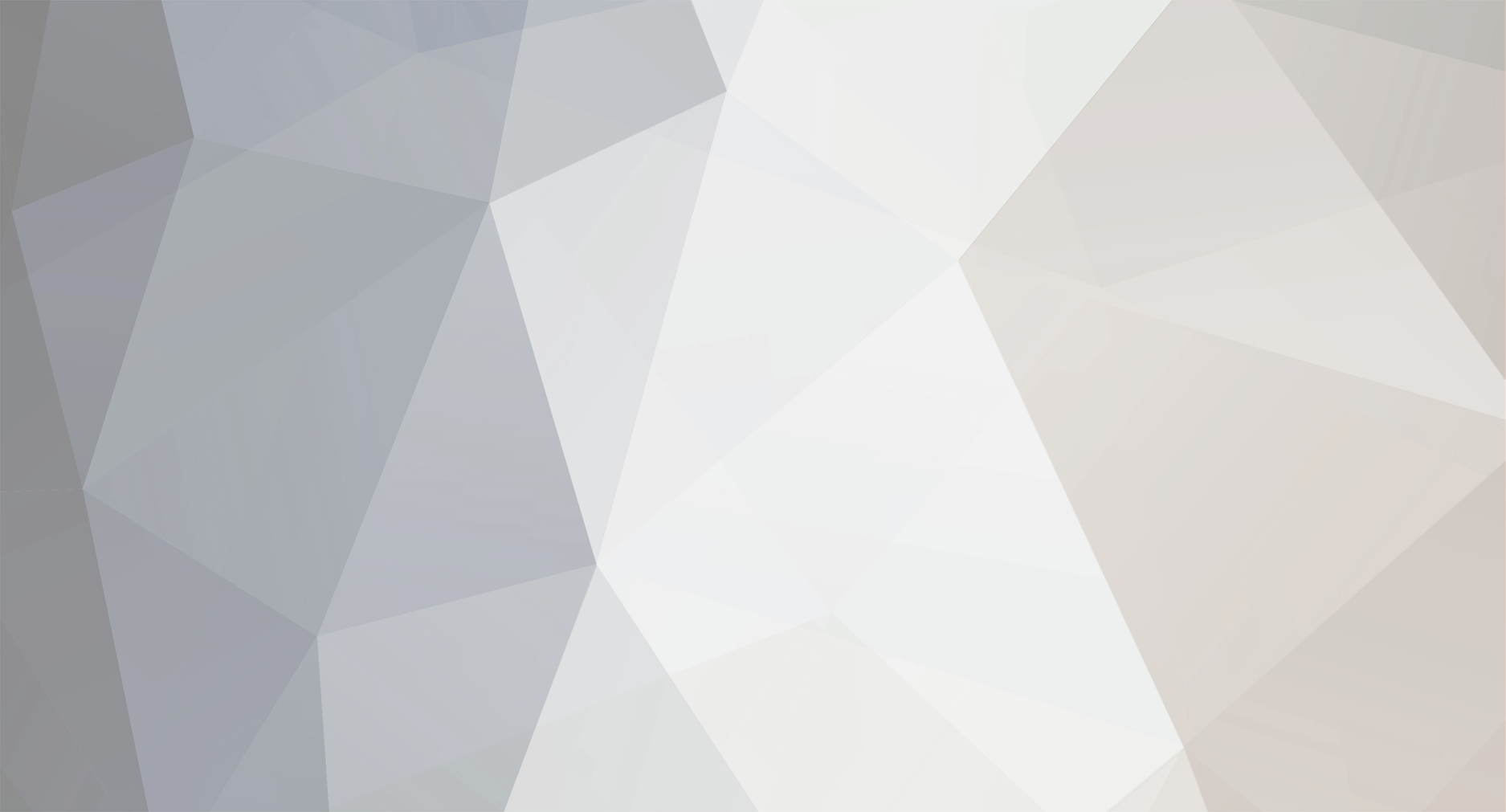
Matenia
Elite user-
Posts
2231 -
Joined
-
Last visited
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Everything posted by Matenia
-
Works for me, with my normal license key (btw, this is the Alliance Quester you are commenting on, but I've only sold the Horde quester). @Droidz added the encryptor recently. Is there any reason why it wouldn't work for someone? When I tried it with the TRIAL key, I get the same error as @yadig1. For now, I will just update the files on Rocketr with the unencrypted version. I hope Droidz can resolve this soon, as I really don't want my product to be easily sharable. EDIT: After having more users test them, they work correctly. Currently, with the TRIAL key it seems to not work. Therefore I will re-upload the encrypted versions and ask @Droidz for clarification. here is proof that these profiles work, in the encrypted version.
-
How can I do this on Easy Quest Pofile?
Matenia replied to gto102's topic in WRobot for Wow Vanilla - Help and support
Use 2 quests with the same id. On the first step ("Pulse") you get the item and as CompleteCondition you can check if the item is in your bags (code snippets all over the forums). On the second one, just pick "UseItemOn", add a waypoint and it will use the item there. No need to put any custom complete condition. -
The battlegrounder product settings allow you to create your own profile.
- 3 replies
-
- battlegrounder
- battlegrounds
-
(and 2 more)
Tagged with:
-
You just overwrite the empty files in the battlegrounder folder. Make sure they're names exactly the same and they will be picked up
- 3 replies
-
- battlegrounder
- battlegrounds
-
(and 2 more)
Tagged with:
-
Feed Pet problem
Matenia replied to Photogenic's topic in WRobot for Wow The Burning Crusade - Help and support
I think it's finally time you convert your fightclass to C# or buy Jasabi's. What you'll have to do is cancel all events in the fight loop, while your pet (and begin fight, I believe), while your pet has the feeding buff. You can find how to do that on the forums, but you can't easily make that part of an XML fightclass. wManager.Events.FightEvents.OnFightLoop += (WoWUnit unit, CancelEventArgs args) => { while(ObjectManager.Pet.HaveBuff("Feed Pet") { Thread.Sleep(500); } }; Haven't tested this. You might have to use OnFightStart instead of OnFightLoop. -
How can I train skills every 4 lel ?
Matenia replied to gto102's topic in WRobot for Wow Vanilla - Help and support
Answer is on the forums. You can also modify the levels in one of the TrainLevel plugins here. If you add your trainer in SW and set your bot to use flightmasters, it will choose them to make the route quicker. -
You still haven't understood me. You did exactly what I wanted you to do. 2 separate quests you can pulse (with the same quest id, but different target entries for different mobs). The only difference is you're using C# to directly checking for objective count on the quest objectives, I suggested you use XML to do so. I guess I should've posted a longer example to make it more clear.
-
What you're doing is essentially the same thing I suggested, except I wanted you to fill in the complete condition in XML, whereas you use C#. You'll still need 2 pulses for 2 different quests, both of which have a different objective that needs completing. Each quest then has a different target entry set.
-
Look at the XML file it generates. Looks like this and works fine: <ObjectiveCount1>0</ObjectiveCount1> <ObjectiveCount2>1</ObjectiveCount2> <ObjectiveCount3>0</ObjectiveCount3> <ObjectiveCount4>0</ObjectiveCount4> <ObjectiveCount5>0</ObjectiveCount5> <AutoDetectObjectiveCount1>false</AutoDetectObjectiveCount1> <AutoDetectObjectiveCount2>false</AutoDetectObjectiveCount2> <AutoDetectObjectiveCount3>false</AutoDetectObjectiveCount3> <AutoDetectObjectiveCount4>false</AutoDetectObjectiveCount4> <AutoDetectObjectiveCount5>false</AutoDetectObjectiveCount5>
-
Aldor / Scryer Repeatable Turin
Matenia replied to Grimfell's topic in WRobot for Wow The Burning Crusade - Help and support
For something like this just get the addon TurnIn and spam click the NPC for a few seconds -
Create 2 quests with the same id, but different names (so you can pulse both steps), then set the objective count 1 to whatever number the first kill requires in your questlog (8, for example), and on the second quest, you set objective count 2 to 6. Set all other objective counts to 0. Now you can pulse both steps that each contain different information on where and what to kill.
-
Crazy circles at flags in AV
Matenia replied to joeblowy's topic in WRobot for Wow The Burning Crusade - Help and support
Make your own profiles -
Wrobot CPU usage
Matenia replied to Photogenic's topic in WRobot for Wow The Burning Crusade - Help and support
You're using MoP. The client is a buggy mess for private servers. So just use this plugin to auto-fix it: using System; using System.Threading; using System.Threading.Tasks; using System.Diagnostics; using System.ComponentModel; using System.Collections.Generic; using System.Collections; using robotManager.Helpful; using robotManager.Products; using wManager.Plugin; using wManager.Wow.Enums; using wManager.Wow.Helpers; using wManager.Wow.ObjectManager; public class Main : IPlugin { private Stopwatch ActionTimer; private bool isRunning; private BackgroundWorker pulseThread; public void Initialize() { isRunning = true; EventsLuaWithArgs.OnEventsLuaWithArgs += Events; EventsLua.AttachEventLua(LuaEventsId.PLAYER_ENTER_COMBAT, m => Event_PLAYER_ENTER_COMBAT()); EventsLua.AttachEventLua(LuaEventsId.PLAYER_LEAVE_COMBAT, m => Event_PLAYER_LEAVE_COMBAT()); EventsLua.AttachEventLua(LuaEventsId.PLAYER_REGEN_ENABLED, m => Event_PLAYER_LEAVE_COMBAT()); Logging.Write("[LuaCombatFix] Init"); ActionTimer = new Stopwatch(); pulseThread = new BackgroundWorker(); pulseThread.DoWork += Pulse; pulseThread.RunWorkerAsync(); } public void Events(LuaEventsId id, List<string> args) { /*for (int i = 0; i < 11; i++) { Logging.Write(id.ToString()); Logging.Write("\nArg" + i + " contains: " + args[i]); }*/ if (id == LuaEventsId.UNIT_SPELLCAST_SENT && args[0] == "player") { Logging.WriteDebug("[LuaCombatFix] Player cast sent: " + args[1]); if (ObjectManager.Me.InCombat) { //Logging.WriteDebug("[LuaCombatFix] Resetting timer with time left: " + ActionTimer.ElapsedMilliseconds); ActionTimer.Reset(); if(!ActionTimer.IsRunning) { ActionTimer.Start(); } } else { ActionTimer.Reset(); ActionTimer.Stop(); } } } public void Pulse(object sender, DoWorkEventArgs args) { try { while (isRunning) { if (!Products.InPause && Products.IsStarted) { Logging.WriteDebug("[LuaCombatFix] Checking Timer with time left: " + ActionTimer.ElapsedMilliseconds); if (ActionTimer.ElapsedMilliseconds >= 8000) { Logging.Write("[LuaCombatFix] Fixing Combat"); ActionTimer.Reset(); Lua.LuaDoString("ReloadUI()"); } } Thread.Sleep(1000); } } catch (Exception ex) { Logging.Write(ex.Message); } } public void Event_PLAYER_ENTER_COMBAT() { Logging.WriteDebug("[LuaCombatFix] Player entering combat with time left: " +ActionTimer.ElapsedMilliseconds); ActionTimer.Reset(); ActionTimer.Start(); } public void Event_PLAYER_LEAVE_COMBAT() { Logging.WriteDebug("[LuaCombatFix] Player leaving combat with time left: " + ActionTimer.ElapsedMilliseconds); ActionTimer.Reset(); ActionTimer.Stop(); } public void Dispose() { try { isRunning = false; Logging.Write("[LuaCombatFix] Stopped"); } catch (Exception ex) { Logging.Write(ex.Message); } } public void Settings() { Logging.Write("[LuaCombatFix] No settings available"); } } -
Wrobot CPU usage
Matenia replied to Photogenic's topic in WRobot for Wow The Burning Crusade - Help and support
I already have all that, since I write my own fightclasses (including targeting). So I guess I could easily load my fightclasses as a plugin instead, to reduce load and then just delete wRotation.dll? Usually my bots are never sitting above 5% CPU load anyway, but sometimes after running it all day, it really starts building up, especially if I do a lot of shit in BGs. -
Wrobot CPU usage
Matenia replied to Photogenic's topic in WRobot for Wow The Burning Crusade - Help and support
To clarify, you just use your own targeting logic and then basically just call your own C# fightclass yourself? -
Version 1.0.2
412 downloads
This plugin is great for leveling mining. The bot usually doesn't know which nodes you can mine with your current skill. Therefore, when you use this plugin, it will blacklist all nodes you cannot mine with your current skill, so the bot will not even approach them. It doesn't waste any time and makes you look a whole lot less like a bot. It will clean the blacklist whenever your skill updates. Example debug log: -
You can use C# Lua.RunMacroText("/cast Chain Heal(Rank1)"); Or Lua directly CastSpellByName("Chain Heal(Rank1)")
-
Game version incorrect
Matenia replied to suss1's topic in WRobot for Wow The Burning Crusade - Help and support
The trial is only for private servers and should work. However, I figured that something may have corrupted the your install (maybe faulty RAM), which could be your problem. That's why I suggested a complete pre-installed client download (try the one Excalibur supplies). -
Game version incorrect
Matenia replied to suss1's topic in WRobot for Wow The Burning Crusade - Help and support
Try to download a different pre-installed client. The Feenix one is modified, for example. -
Game version incorrect
Matenia replied to suss1's topic in WRobot for Wow The Burning Crusade - Help and support
Start both WoW and the bot with admin privileges -
Request: Arathi Basin
Matenia replied to nofancy's topic in WRobot for Wow The Burning Crusade - Help and support
Make your own profile, don't use the default