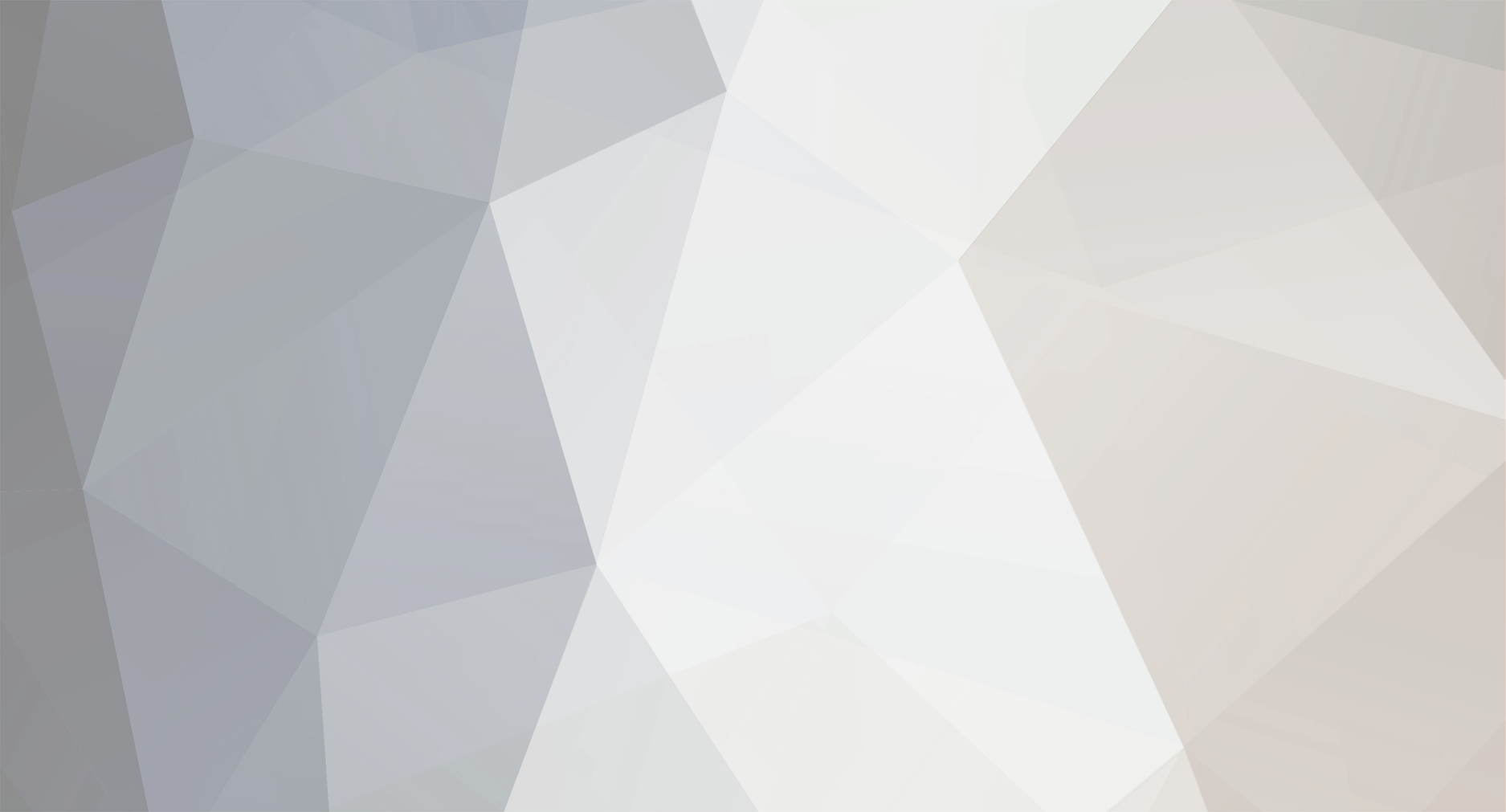
Matenia
-
Posts
2226 -
Joined
-
Last visited
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Posts posted by Matenia
-
-
Just wanted to chip in and say you absolutely shouldn't store NPCs in the database. Temporarily adding them (unless already available) is fine. But if you save them, you'll eventually give WRobot too much info that it doesn't need. It will go to the wrong NPCs/trainers just because they're closer in 2D space.
Back in the day before I wrote my own states and completely got rid of WRobot's states, I would intercept the ToTown state, clear the NPC DB and just inject my own based on 3D distance from a pre-select NPC list.
WRobot will do a lot of dumb things if you give it free reign. Having a ton of NPCs available is just asking it to make the wrong choices.Intercepting states and forcing selection is absolutely the way to go. I know people who wrote specific questers/grinders for their operations that would force inject a food vendor and repair for every level section and overwrite them with every "zone" change. Same for trainers, pretty much.
-
Für alle Versionen
-
Für Legion und aufwärts gibt's das glaub ich nicht.
@Droidz is TRIAL completely turned off or only for the WoW 6.0+ versions of WRobot?
-
Ist weiterhin möglich. Du müsstest TRIAL als Key eingeben und dann + drücken um den Key hinzuzufügen bevor du ihn verwendest. Aber lass dir gesagt sein, wenn du gar kein Englisch sprichst bist du hier falsch.
Es läuft fast alles auf dem englischen Client, was die Community so erstellt, und um überhaupt irgendwas zu finden, musst du in den Foren eigentlich Englisch beherrschen. -
Fightclass folder, just like everything else. Then you select it, just like any other
-
Just wanted to pop in and say for Vanilla it's really worth finding some of the older wiki entries regarding Tooltip handling in general. Tooltip handling in vanilla is very different from later expansions and honestly such a mess.
I don't think you can just destroy tooltips. For example, as far as I know, you can not get ToolTips to be garbage collected by just setting them back to 'nil'.
They will continue to exist and you're just dumping your memory full of new tooltips eventually. You absolutely need to reuse the tooltip and clear all lines as well as assigning a new owner.As far as I remember, in Vanilla you even need to manually delete some sub texts on the tooltip because it's such a rudimentary (buggy) implementation in 1.12. The old wiki entries should have more info.
A code snippet from my own TBC+ code:
List<int> containerSlot = Bag.GetItemContainerBagIdAndSlot(bagItem.Entry); if (!containerSlot.IsNullOrEmpty()) { Lua.LuaDoString($@" {TooltipName}:Hide(); {TooltipName}:SetOwner(UIParent, 'ANCHOR_NONE'); {TooltipName}:ClearLines(); {TooltipName}:SetBagItem({containerSlot[0]}, {containerSlot[1]}); "); ParsedItem parsedBagItem = ItemParser.ParseCurrentTooltip(TooltipName); ...
-
Either your parameters aren't correct for 3.3.5a or the server isn't giving your client the correct info. Or even worse, your client is modified.
local name, nameSubtext, text, texture, startTime, endTime, isTradeSkill, castID, notInterruptible = UnitCastingInfo(unit);
This is taken from 3.3.5a interface files. notInterruptable probably returns nil, so you may have to double check what actually is returned.
Here's the source:
https://github.com/wowgaming/3.3.5-interface-files/blob/main/CastingBarFrame.lua -
Your Lua is wrong. The second local shouldn't be there. You're just creating a second local variable called name in that conditional block instead of overwriting the one outside of it.
Instead of searching for a variable that will constantly be overwritten, just return what you need like so:
private bool SpellIsInterruptible() { return Lua.LuaDoString<bool>( @" local name, _, _, _, _, _, _, notInterruptible = UnitCastingInfo(""target""); if not name then name, _, _, _, _, _, notInterruptible = UnitChannelInfo(""target""); end if name then return not notInterruptible; end return false; "); }
-
I believe you can just let the state run that originally triggered your event handler (ToTown, most likely). It should know vendors in Moonglade, if your database is correctly filled.
If you really needed to, you could just create an instance of ToTown and Training in your plugin and run those manually. The run method should be blocking and as long as you're in Moonglade, there shouldn't be anything interrupting the state so you don't need (much) extra logic to figure out if it was successfully run.
-
It absolutely won't happen with a bot. Maybe if you tried to code the routine from scratch, but you'd have to basically forsake all of WRobot's normal combat management etc.
-
You'd have to intercept ToTown using State Events.
An example can be found here. You can adjust it to use the Moonglade spell instead. -
Look at what I linked. The error happens ONCE after you add your state. This code works fine - wRobot's FiniteStateMachine that iterates the states throws the error.
Since you only modify the collection ONCE to add your state(s), the very next iteration across all states works just fine. I'm telling you, you can ignore the error.
-
That error happens once, in WRobot's code. Next iteration it does, it now has your states in it and everything works without error.
Edit: These are 5 years old, but the code is still applicable as far as I know. No guarantee PartyBot Helper still works with HMP. -
It's probably your fightclass pausing to achieve something (like regen to summon pet).
Check the source code. If it doesn't pause anything - you're probably using the PC and hitting the shortcut to pause WRobot.It seems you're not using plugins and only Grinder, not even a Quester. So there's no code injections outside of your fightclass as far as I can tell.
-
Try
Lua.LuaDoString("TradeSkillCreateAllButton:Click()")
If that doesn't work, the problem seems to be that Wrobot executes secure calls and the button won't won't work when doing so. There might be an extra argument that allows disabling secure call.
Additionally, maybe the anti-cheat protection WRobot uses breaks some code. -
It's a common bug on some shitty private servers. Nothing that can be done and not related to WRobot.
You can try and hope setting higher (500-1000ms) in advanced WRobot settings will alleviate it slightly, but this shit happened all the time, especially on vanilla servers.
Best bet is to use the relogger and just hourly relog if your server has this issue constantly. -
If you can't access the authentication server through the great firewall, you need to use a VPN that can connect to it
-
Get behind some proper VPNs. Worst case scenario, join a torrent tracker and find some C# ebooks
-
IsFacing needs a radian cone as an argument. I never got it to work well. You just need to implement the math yourself and hope that works
-
If you use WRotation only and still get banned it's just proof that the bot is detected. If you don't get banned then, it's not detected automatically. Rather they detect certain profiles, grinding statistics etc
-
They likely have you fingerprinted and some decent-ish detection when it comes to bot behavior.
If you use WRotation and don't get banned, you're safe.But then you also know it's likely related to movement. Meaning you can try a few things like enabling/disabling smooth movement in WRobot, using Lua movement, keyboard movement (disabling CTM entirely), trying CTM with your game locked to 60 Hz via Rivatuner, etc.
Considering my second character I used for testing (so it got stuck a lot, as I was refining my products) got to level 40 before getting banned, I'm fairly certain GMs just sit in starting areas while they answer tickets and ban some bots. Once you get past that, you're pretty safe and it's only player reports.
-
Is this for Vanilla? For Vanilla clock to move to work, you need to be at 60Hz. You can use Rivatuner Statistics Server to limit your Wow.exe to 60 fps for the same effect.
Otherwise the timestamps internal to the client are off and it won't work.
Just iterate the list and within each step, use blocking code that only succeeds and jumps to the next step in the loop if ObjectManager.Pet.Position.DistanceTo(Vector3) < 3 -
That's not what he said. He said meta info is cached. Buffs are still read from memory every time you make a call.
If you're making a fightclass and want it to be a efficient, create a state of truth for all units around you and make sure it doesn't change until after the iteration is over.
That holds true for ids, distance, buffs, debuffs, whatever you need to make decisions in your rotation -
Vanilla is probably the worst expansion to work with, but it's also had a multitude of profiles released. There's fightclasses for every class (even free), tons of profiles, etc.
A couple years ago, it had the most paid content and some of it is still up. Some of it has been released for free if the creators disappeared.
However, if you're not willing to do anything yourself/barely set anything up besides loading an old profile that may not fully work anymore or isn't 100% compatible with your server, you're out of luck.
https://wrobot.eu/files/category/164-plugins-vanilla/
https://wrobot.eu/files/category/163-fight-classes-vanilla/
https://wrobot.eu/files/category/166-grinder-vanilla/
Force to Moonglade on "ToTown" state.
in WRobot for Wow Vanilla - Help and support
Posted
I actually think these threads are quite constructive, so I'm going to share some pathing code I use in HMP to hopefully help you out.
You can specifically add OffMesh connections. In fact, you can add them to be used EVEN if WRobot can still find a path. You can also store functions in WRobot's internal Vars, which can be referenced using the C# string that offmesh connections are capable of using. So you could even call them from a quester profile, as long as you save the function in that Var map first.
Here is an example of how to use Darnassus connections. It teaches WRobot that the portals and ships are just regular pathing connections.
For the sake of at least protecting some of my "secrets" of several thousands of hours that went into HMP since 2016, I'm not going to share my ship implementations. They are simple enough that I think you could figure them out though.
Back when I started, none of this was documented and I basically had to trial and error through a lot of it or build things from scratch. Thankfully @Droidz was very, very helpful and even added more Offmesh functionality for people to use more easily.
I hope this helps at least a few people in the future if they ever stumble upon this topic covering custom pathing, offmesh connections or Darnassus portals or ships. Of course, this can be adapted to add new elevators or similar portals too.