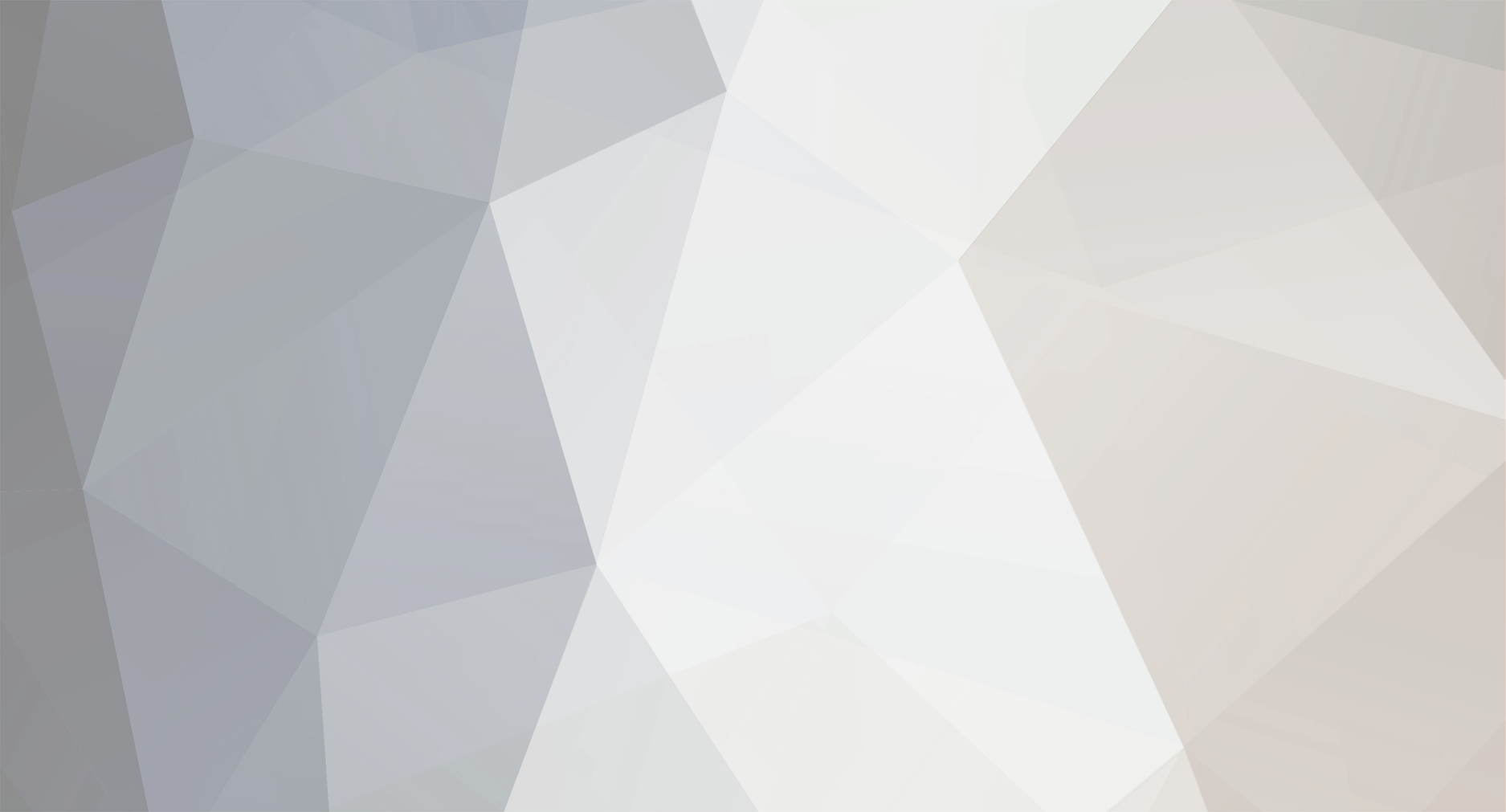
Matenia
-
Posts
2232 -
Joined
-
Last visited
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Posts posted by Matenia
-
-
Check out the thread regarding repair. They're stickied. There's an error at the top, that might need fixing (like you installing .NET Framework).
However, it also seems you're trying to run a grinder without a profile? Or maybe you bought a grinder here that needs to be started as quester?
-
The simple answer is no. The longer answer is that through Lua events and more complicated C# code, you might be able to achieve that. You could also just record a quest with "FollowPath" type and tick the little box with "Is complete when last path reached".
But something like "have I actually interacted successfully with the flightmaster" might not really work.
What you could do is set said flight master as a quest giver. You would then set your 1 step (in the quester) as to pick up some random quest (that's unlikely/impossible to be finished) and your bot would run there, interact with the NPC trying to pick it up, but not find it. Thus you'd get stuck at the NPC.
-
You can use the quester and use a CompleteCondition of having reached a certain zone name or whether "return new Vector3D(x, y, z).DistanceTo(ObjectManager.Me.Position) <= 5".
-
Other people who come here and ask questions are generally willing to put in some time and effort into understanding. I am not asking you to become a programmer. I am not asking you to study software engineering. I am asking you to invest (once!) 2-3 hours to understand the very, very basics, so that you can read/understand (very roughly) the code snippets you are given, as opposed to expecting others to do work for you.
I know this isn't too much to ask for, because it's no more complicated than learning the fightclass editor (in fact, it's less complicated, because it requires less logic). I also know this isn't too much, because plenty of other people on here and Discord have managed - with zero knowledge - to make something of the code snippets.
I am not coming at you, but if I didn't feel a general unwillingness to learn, I wouldn't have said anything. Consider this constructive feedback, as I am still trying to help you.
This one, simple article covers everything you need to know. You'll be able to understand what it is that you're actually doing and the next time someone gives you code that isn't copy-pastable but still correct, you'll know how to apply it. -
You are supposed to have minimal understanding of what you're doing. The code can't just be copy pasted as Csharp.
Take the Lua part of it, so everything in green until the first comma. Then do a Lua condition, as search you put hasMainHandEnchant and as result, you put true (or 1, I'm not 100% sure, but you can look at the API documentation of GetWeaponEnchantInfo to see what it returns).
I really think it's time for you to understand the things you're doing here. You constantly expect people to do work for you, so you may profit of it. You expect to be given code snippets that are copy paste ready.
The only reason I'm still helping is because you've shared fightclass before and I hope you will contribute to do so.
I highly recommend looking at a basic programming course. Even if it's just the first chapter, it would be very useful if you understood variables, functions and types. It would help you read Lua documentation on your own and better understand the code snippets people are giving you.
There are some cheap and free JavaScript courses out there. It's extremely similar to Lua and will teach you the basics.
-
private bool HasMainHandEnchant() { return Lua.LuaDoString<bool>("hasMainHandEnchant, mainHandExpiration, mainHandCharges, hasOffHandEnchant, offHandExpiration, offHandCharges = GetWeaponEnchantInfo();", "hasMainHandEnchant"); }
This is the C# code I use to determine whether the mainhand is enchanted (has a poison).
The Lua is right in there. You can choose yourself which language to use.
-
-
You can buy a legion quester, they will work on Tauri for the most part.
-
Oh yeah, you'd need to disable bot movement during your own, I suppose. Can't do that without C#.
-
The bot just runs through a loop of your rotation at certain intervals. That takes a while, usually not more than 100 milliseconds. But if, for example, your global cooldown ends between it checking whether to cast Mangle (higher priority than Fairie Fire) and Fairie Fire, you end up casting what's lower in the list.
-
This plugin reads profession level through Lua. You can check out the source code.
Here is the relevant C# code:
private void SetMiningSkill() { CurrentMining = Lua.LuaDoString<int>(@" miningSkill = 1; for i = 1, GetNumSkillLines() do local skillName, isHeader, isExpanded, skillRank, numTempPoints, skillModifier = GetSkillLineInfo(i) if isHeader and not isExpanded then ExpandSkillHeader(i); end end for i = 1, GetNumSkillLines() do local skillName, isHeader, isExpanded, skillRank, numTempPoints, skillModifier = GetSkillLineInfo(i) if not isHeader and skillName == ""Mining"" then miningSkill = skillRank end end ", "miningSkill"); }
-
You can try a higher latency, but at this point, only a C# fightclass can really achieve what you need.
500-850 latency might fix it -
Spells with () in the name can't be executed by the TBC client unless you add Rank(x). Can confirm what @lonellywolf said, I debugged this last week.
-
I have 1-60 and 60-70 alliance questing on MoP, but it's still unfinished and a bit buggy. Tested it on Tauri bakc when it came out.
You can join my Discord, if interested, but I won't be giving any support if you end up buying it (for a low price).
-
Set it higher and make sure to activate auto-loot
-
Set your latency in general settings from 600 to 900.
-
That's most likely a wRobot problem (if your Bot State does show it's stuck at PickUp or TurnIn). You can get an addon like TurnIn to automatically accept and turn in quests.
-
25 minutes ago, reapler said:
@apexx Namespace System.Linq is not referenced. So you need to copy this on the top of your code:
using System.Linq;
Both .Where() & .Count() are included in Linq, that's the reason why .Count() couldn't work because it doesn't had an overload available.
So in the end you can use this:
if (ObjectManager.GetUnitAttackPlayer().Count(u => u.GetDistance <= 6) > 2) { }
Or use other Linq methods.
Didn't know Linq hatd Count() - for some reason I had gotten compile errors before. iMod recently told me I could use .NET later than 4.0 too, maybe that's why I hadn't noticed it so far.
-
-
Try the EvadeHate plugin.
-
This cleans your blacklist and blacklists nodes you are too high level for by default. It should solve your problem. -
My comment as to it being broken on Warmane is incorrect as of today. I have used it several times successfully.
If you still have issues, repair the bot as Droidz advised (or better yet do a full reinstall).If you have problems with the bot reloading your UI, you most likely have faulty addons fucking with your addon memory. Disable all addons. If this is not the case, the fightclass you chose might be overloading your addon memory.
I highly recommend using a fightclass that's been proven to work. In @eniac86's log I can clearly see he is trying to use a retail hunter fightclass for 1.12. Things like that can easily mess with the bot.
-
You can also do something like
return Quest.IsObjectiveComplete(1, 1);
to check for objectives (and their count)
If you haven't turned the Quest in yet -
Use 2 quests with the same id. On the first step ("Pulse") you get the item and as CompleteCondition you can check if the item is in your bags (code snippets all over the forums).
On the second one, just pick "UseItemOn", add a waypoint and it will use the item there. No need to put any custom complete condition.
Get started
in WRobot for Wow Wrath of the Lich King - Help and support
Posted
https://wrobot.eu/forums/topic/1381-repairinstall-wrobot/#comment-966