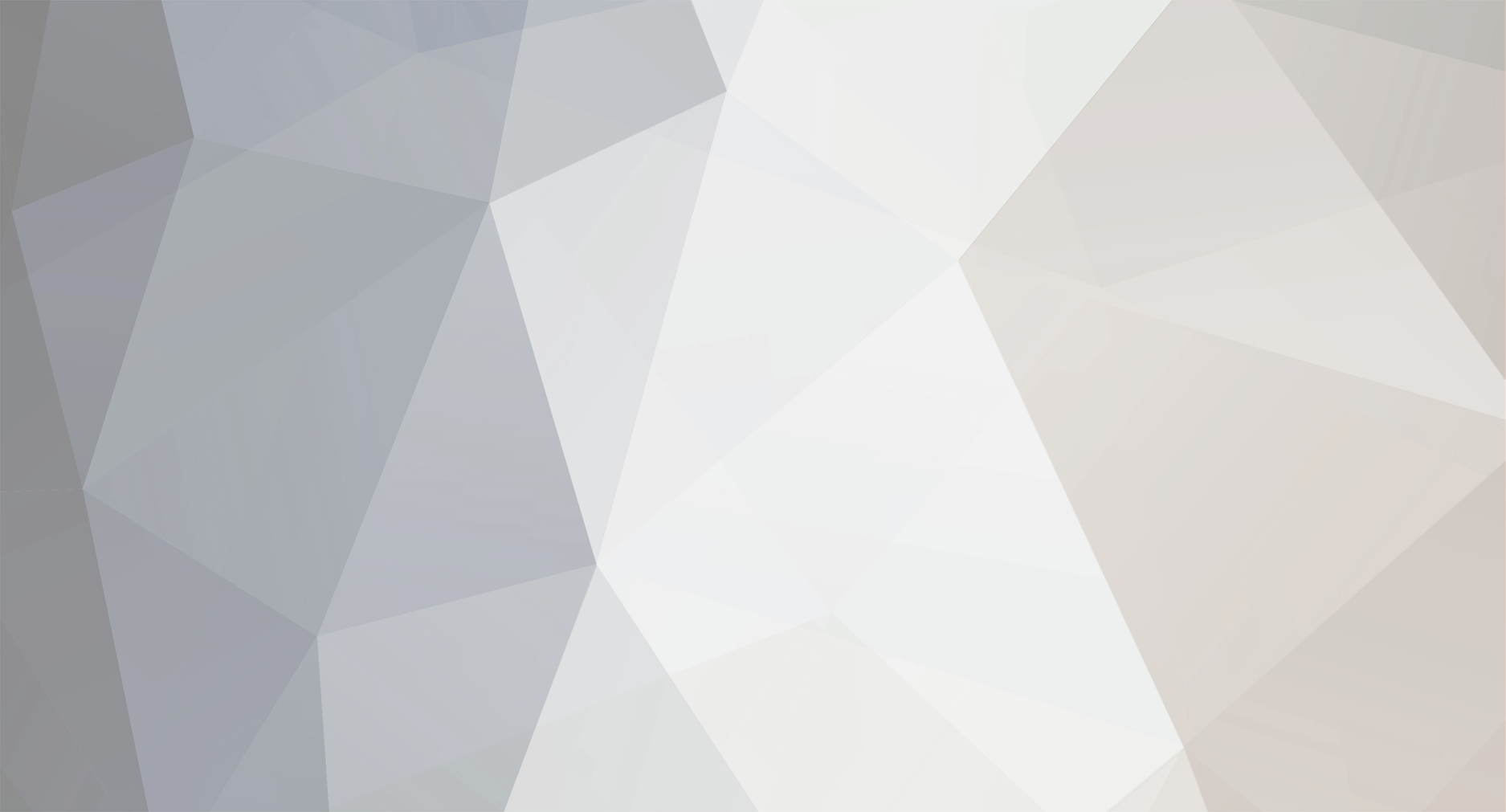
headcrab
-
Posts
93 -
Joined
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Posts posted by headcrab
-
-
If your target is WoWPlayer or WoWUnit you can get it class:
WoWClass targetClass = ((WoWUnit)target).WoWClass; swith (targetClass) { case WoWClass.Warrior: //melee case WoWClass.Paladin: // melee case WoWClass.Hunter: // range //blablabla }
but in some cases you need also target specialization (for example, for Druid). Its possible, but only on battlefield. Look Battleground helper plugin, how it detects enemy healers, no problem to detect melee/range too
-
5 hours ago, Drwolftech said:
Yes, but i use xml fight class for every class i play.
I had the same problem with xml fight classes and solved it by this way:
In fight class editor there is spell option "Lock Frame", true by default. In raids/battlegrounds there are too much events handled by robot, and this option can freeze your screen. Simply set false value for spells with heavy/long-calculated conditions, and it will unfreeze your screen. For spells with instant-calculated conditions you can leave true value.
Usually, C# classes dont use this option
-
Do you use XML fight class for your death knight?
-
You can do some research with dnSpy. Download dnSpy.zip, unpack, run and open wManager.dll - and you will see how robot works. New versions are obfuscated, and is impossible to understand what's going on inside, but old are pretty clear (if you know c# or resembling language). Using old and new versions you can find similar places in code and even do patch, for example, change this 45f to 60f. Or understand, is it possible to customize robot with plugin
- Matenia and ScripterQQ
-
2
-
indeed, i forgot protected lua available from robot, but you want call it from wow process. So, you still need unlocker for addon.
But because they both (robot and unlocker) change the same memory address in wow process, you will get this error sooner or later
-
Ofcourse, you can check it. Simply run protected function CastSpellByName with any available spell name :)
-
You do not need any unlocker when robot run, because robot unlocks lua. Simply close EWT.
-
1 hour ago, scripterx said:
Ok maybe I don't get your reply at all, but I just want to know where that 45 comes from.
45f is hardcoded in FightBG private function.
Do you have decompiler? Try to find old, non-obfuscated robot and open function used in FightBG.StartFight. You will see something like this:
if (woWUnit.Position.DistanceTo(position) > 45f) { Logging.WriteDebug(BlackListSerializable.Icaleodoarea()); return Int128.Zero(); }
In old versions you can change this value and recompile, new versions are obfuscated, only byte-patch can help
-
plugin can switch target only if distance to new target is less than CustomClass.GetRange
To be more precise, it switch and bot starts move to target, but if someone attack you while move, bot will switch to nearest attacker. So works robot's algorithm
-
Yes, i looked into robot code, its not enough to find new target, but need to cancel current fight too. Added it into new plugin version (2.1.0), now switching is more precious (look code for FightEvents.OnFightStart and FightEvents.OnFightLoop).
You can see switch events in log (">>>>Attack enemy healer<<<< ")
-
Did you saw Battleground helper? It contains example how to switch to enemy healer in radius (FocusHealer method). Simply filter enemies and order by distance
-
You can patch wManager.dll this way:
1) Make shure bot cant find bobber. Go to fishing water and run this c# code in developement tools:
Spell _f = new Spell("Fishing"); for (int i=0; i<10; i++) { _f.Launch(false, false, true); Others.Wait(2000); Logging.Write("Bobber GUID = "+Fishing.SearchBobber()); Lua.LuaDoString("SpellStopCasting()"); Others.Wait(100); }
look in the log. If there always this strings, it means bot cant find bobber:
[F] 19:10:33 - [Spell] Cast Fishing (Fishing) 19:10:35 - Bobber GUID = 0
2) try to find minimum wait value to find bobber. Gradually increase 2000 -> 3000 until you see in log non-zero bobber GUID. This code will help you:
Spell _f = new Spell("Fishing"); for (int i=2000; i<3000; i=i+100) { _f.Launch(false, false, true); Others.Wait(i); Logging.Write("Wait:" + i+ " Bobber GUID = "+Fishing.SearchBobber()); Lua.LuaDoString("SpellStopCasting()"); Others.Wait(100); }
If found, you can patch program
3) download and install dnSpy (dnSpy.zip)
4) run dnSpy, open wManager.dll and find method wManager.Wow.Bot.Tasks.FishingTask.LoopFish (with many arguments)
You will see obfuscated code, but there is string easy to find. It should looks like this (contains symbols ThreadStart):
5) click on the method in the end of string (name can be different). You will open this method. Find in this method string "Others.Wait". This is place to patch
6) Right click ans select "Edit Method (C#)". As argument type value you found, for example, 2500:
7) Compile, save, and enjoy
Never update your robot, or do this steps again, if updated, and fising not works as before
-
Even better, no need to interrupt, simply remove ground mount for N milliseconds :)
private void mountDelay(int delay) { if (delay == 0) return; string mountName = wManager.wManagerSetting.CurrentSetting.GroundMountName; if (mountName == null) return; new Thread(() => { wManager.wManagerSetting.CurrentSetting.GroundMountName = null; try { Thread.Sleep(delay); } catch (Exception arg) { Logging.WriteError("Exception during nomount: "+arg, true); } finally { wManager.wManagerSetting.CurrentSetting.GroundMountName = mountName; } }).Start(); }
Will publish in next plugin version
-
Found proper event (LUA event PLAYER_UNGHOST), new plugin version uploaded. Now you can tune delay in settings
-
for me this works:
robotManager.Events.FiniteStateMachineEvents.OnRunState += StateWatcher; ... private void StateWatcher(Engine engine, State state, CancelEventArgs cancelable) { if (state == null) return; if (state is ResurrectBG && AutoBGSettings.CurrentSetting.ressBGpause) { string mountName = wManager.wManagerSetting.CurrentSetting.GroundMountName; if (mountName == null) return; wManager.Wow.Class.Spell mount = new wManager.Wow.Class.Spell(mountName); new System.Threading.Thread(() => { System.Threading.Thread.Sleep(1000); Timer mountTimer = new Timer(1000); while (!mountTimer.IsReady && ObjectManager.Me.IsAlive) { if (ObjectManager.Me.CastingSpellId == mount.Id) { Lua.LuaDoString("SpellStopCasting()"); Logging.Write("stop mount"); } System.Threading.Thread.Sleep(50); } }).Start(); } }
Solution is more complex, because ResurrectBG fires many times when player dead. Need to play with timers, but in general it works. I'll test it and will publish new version of plugin
-
Yes, for me too. They work in the same thread and sometimes cast mount starts before ResurrectBG ends. Need to search another event or maybe start thread with mounting control on event start. I will search
-
My previous solution dont work 100% and i found better (simply dont let to use ground mount for 1st second):
if (state is ResurrectBG) { String mountName = wManager.wManagerSetting.CurrentSetting.GroundMountName; if (mountName == null) return; wManager.Wow.Class.Spell mount = new wManager.Wow.Class.Spell(mountName); for (int i=0; i<10; i++) { System.Threading.Thread.Sleep(100); if (ObjectManager.Me.CastingSpellId == mount.Id) Lua.LuaDoString("SpellStopCasting()"); } }
I added it to my Battleground helper plugin, so you can simply install it and select option ResurrectBG pause
-
for me this code helps
static Main(){ robotManager.Events.FiniteStateMachineEvents.OnAfterRunState += (engine,state) => { if (state == null) return; if (state is ResurrectBG) { Usefuls.WaitIsCasting(); Usefuls.WaitIsCasting(); Usefuls.WaitIsCasting(); } }; }
just need some pause to cast 2-3 buffs
- arkhan and ScripterQQ
-
2
-
ObjectManager.GetWoWUnitHostile is useful function in many cases. To select lowest HP enemy, to multi dot, etc. But useless in pvp, because returns List<WoWUnit> objects with WoWPlayer exclusion (there is woWObject.Type==WoWObjectType.Unit condition inside this function). So, proper GetWoWUnitHostile is:
public static List<WoWUnit> GetWoWPlayerHostile() { List<WoWUnit> result = new List<WoWUnit>(); try { foreach (WoWObject woWObject in ObjectManager.ObjectList) { if (!woWObject.IsValid || woWObject.IsInvisible) continue; WoWUnit o; switch (woWObject.Type) { //case WoWObjectType.Unit: // o = new WoWUnit(woWObject.GetBaseAddress); // break; case WoWObjectType.Player: o = new WoWPlayer(woWObject.GetBaseAddress); break; default: continue; } if (woWObject.IsInvisible) continue; if (o.Reaction <= Reaction.Unfriendly && !o.IsDead) { result.Add(o); } } } catch (Exception arg) { Logging.WriteError("GetWoWPlayerHostile error " + arg, true); } return result; } public static List<WoWUnit> GetWoWUnitHostile() { List<WoWUnit> enemies = GetWoWPlayerHostile(); if (enemies.Count == 0) enemies = ObjectManager.GetWoWUnitHostile(); return enemies; }
This function selects enemy players first, and if nothing found, selects hostile units.
I left some commented code in GetWoWPlayerHostile so you can see my 1st variant - 1 list with players and units together. But i think final variant is better for PVP and even for all cases
-
Always false means robot can't recognize player's cast. Maybe bot bug, maybe they use modified client - anyway it will be hard botting on this server. You can use Droidz workaround if it relays only gathering, but it not good if bot can't recognize every cast.
-
try to increase in 4rd string 200 -> 300...400...
Does it helps or every time it writes Me.IsCast=False?
If helps, but requires big value (>500), just forget this server.
-
Looks like WaitIsCasting broken. Does this code gathers herb (with normal latency, less than 500)? What you see in log window?
WoWObject _t = ObjectManager.GetWoWGameObjectByName("Peacebloom").OrderBy(o => o.GetDistance).FirstOrDefault(); Interact.InteractGameObject(_t.GetBaseAddress, true, false); // this code works like Usefuls.WaitIsCasting(); robotManager.Helpful.Timer timer = new robotManager.Helpful.Timer((double)(Usefuls.Latency + 200)); while (!timer.IsReady && !ObjectManager.Me.IsCast) { Thread.Sleep(5); } Logging.Write("Me.IsCast="+ObjectManager.Me.IsCast); while (ObjectManager.Me.IsCast) { Thread.Sleep(30); } Usefuls.WaitIsLooting(); Move.JumpOrAscend();
If not gathers, try to increase in 4rd string 200 -> 300...400 but not much more.
-
Yes, it should. There is condition Conditions.InGameAndConnectedAndAliveAndProductStartedNotInPause, so need to run any product. Can you execute the same code, but with wrotation running? Does it gather herb in this case?
-
Try to add 1 more string to the end of my code, just to check WaitIs.. functions working properly (with normal latency):
Move.JumpOrAscend();
Does bot gather herb in this case?
BG join
in Battlegrounder assistance
Posted
Try to use version 2.2.0, maybe will work without patching