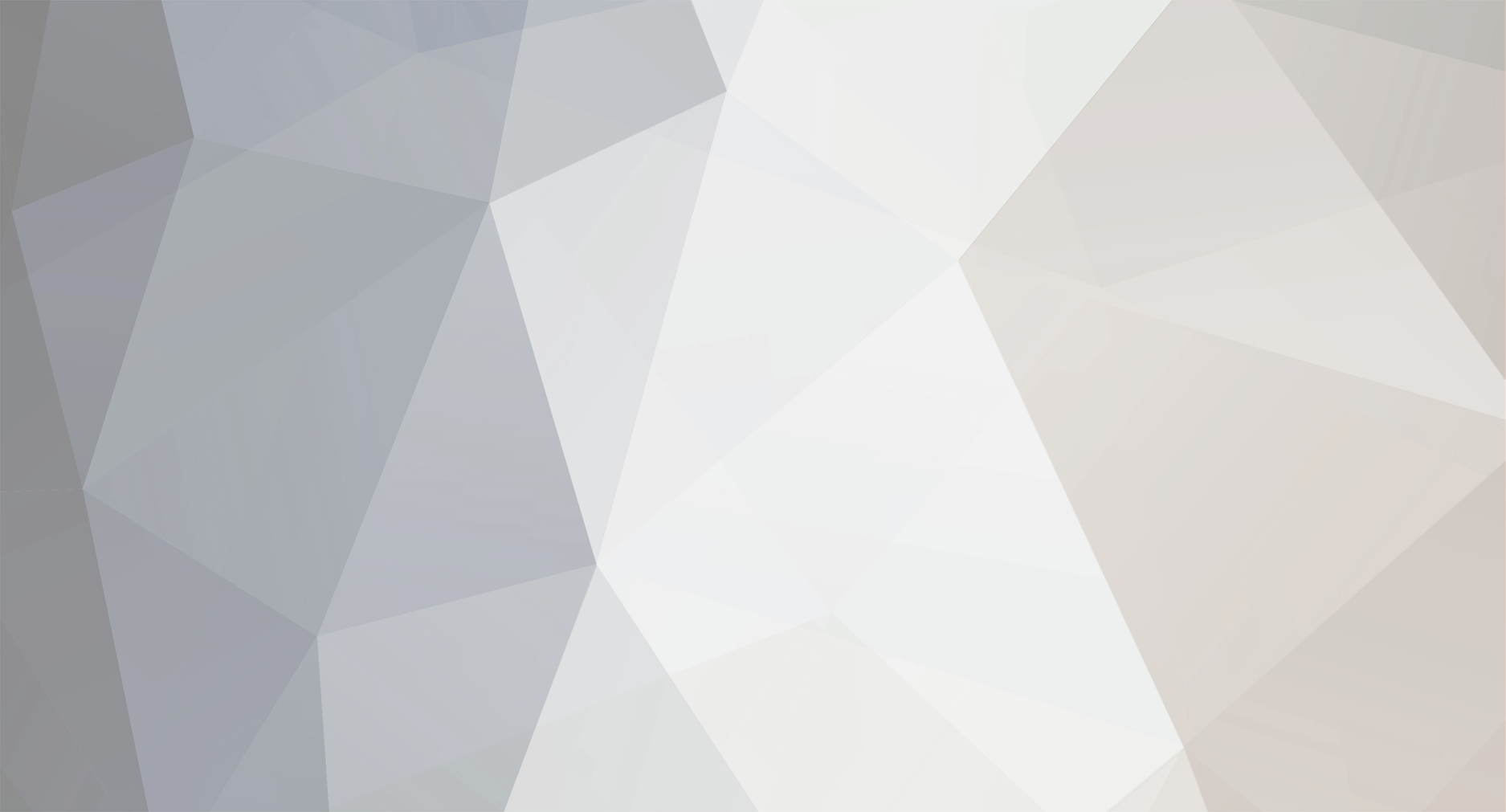
headcrab
-
Posts
93 -
Joined
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Posts posted by headcrab
-
-
Ok. Because all want know, i'll write it here.
-
check AcceptBattlefieldPort is disabled on your server. Open developement tools and run C# code
Battleground.JointBattleGroundQueue(32); // this is random BG Thread.Sleep(1000); Battleground.AcceptBattlefieldPortAll();
This starts BG queue, and should accept BG when it ready. If it works, you need no any modifications. If not accepts, LUA function AcceptBattlefieldPort is disabled on your server or works unstable. So, go to step 2
-
download and install dnSpy
-
in dnSpy open wManager.dll and find method AcceptBattlefieldPortAll
-
right click and run menu item "Edit Method (C#)". You will see obfuscated code, but no problem. Find string with Battleground.AcceptBattlefieldPort and comment it
-
compile and save.
Voila, now your battlegrounder will accept BG with Battlegrounder helper plugin, because it uses alternative method.
Dont update your robot, or do this steps after every update
-
check AcceptBattlefieldPort is disabled on your server. Open developement tools and run C# code
-
17 minutes ago, Artud said:
It still doesn't work for me, even with the plugin.
So, AcceptBattlefieldPort is disabled on your server. You have to patch wManager.dll:
- open wManager.Wow.Helpers.Battleground.AcceptBattlefieldPortAll() method
- find and comment string with Battleground.AcceptBattlefieldPort
- compile
After this bot will 100% accept BG with my plugin
-
Can you target it with left button (i have not herbaist right now, so cant check how it works)?
If cant, try this code
WoWObject _t = ObjectManager.GetWoWGameObjectByName("put here name of herb").OrderBy(o => o.GetDistance).FirstOrDefault(); Interact.InteractGameObject(_t.GetBaseAddress, true, false); Usefuls.WaitIsCasting(); Usefuls.WaitIsLooting();
but dont forget to put right herb name
-
-
Target it with cursor
-
Can you find herb node, select it and in developement tools execute C# code:
Interact.InteractGameObject(ObjectManager.Target.GetBaseAddress, true, false); Usefuls.WaitIsCasting(); Usefuls.WaitIsLooting();
does it gather herb?
But set NORMAL latency, 200-300
-
Not latency, but 3000 in my code
Spell _f = new Spell("Fishing"); for (int i=0; i<10; i++) { _f.Launch(false, false, true); Others.Wait(3000); Logging.Write("Bobber GUID = "+Fishing.SearchBobber()); Lua.LuaDoString("SpellStopCasting"); Others.Wait(100); }
Can you find minimum, maybe 2500 ?
Fishing task not depends on latency, you will have to patch wManager.dll, or wait until @Droidz do it. Thats why i say "bad"
-
Now all code is obfuscated and its impossible to understarnd what it doing. Maybe there is logical error in bot. But in previous versions there was this sequence in farming task:
Interact.InteractGameObject(gameObject.GetBaseAddress, true, false); Usefuls.WaitIsCasting(); Usefuls.WaitIsLooting();
And WaitIsCasting algorithm (with my comments):
robotManager.Helpful.Timer timer = new robotManager.Helpful.Timer((double)(Usefuls.Latency + 200)); while (!timer.IsReady && !ObjectManager.Me.IsCast) { // waits until start casting but no more than Usefuls.Latency + 200 if (!Conditions.InGameAndConnectedAndAliveAndProductStartedNotInPause) { break; } Thread.Sleep(5); } while (ObjectManager.Me.IsCast && Conditions.InGameAndConnectedAndAliveAndProductStartedNotInPause) { // waits while casting Thread.Sleep(30); }
Could you run this C# code in developement tools
wManager.DevelopmentTools.OutPutCSharp= Usefuls.LatencyReal;
-
Its bad. Try to increase wait time 2000->3000, but not much more. Does it helps?
-
Simly copy paste my code, or do not rename Fishing in string
Logging.Write("Bobber GUID = "+Fishing.SearchBobber());
-
Sorry, wrong variable name. Need to rename, for examlpe, Fishing -> _f
Spell _f = new Spell("Fishing"); for (int i=0; i<20; i++) { _f.Launch(false, false, true); Others.Wait(2000); Logging.Write("Bobber GUID = "+Fishing.SearchBobber()); Lua.LuaDoString("SpellStopCasting"); Others.Wait(100); }
-
latency is just server reaction time, you do not need so high settings. 500 ms is enough to get response from server. It recognises you start interact with herb node, and player start casting "Herb Gathering" spell. After this bot waits while player casting (infinite) and then looting (latency+2000).
When gather manually, do you need wait 2500 ms before "Herb Gathering" starts? I think no.
So, suspecting something else. I think it HumanMasterPlugin - it starts moving too early. Disable it and check herb gathering
-
To find the cause go to fishable water, open developer tools and run this C# code:
Spell Fising = new Spell("Fishing"); for (int i=0; i<20; i++) { Fishing.Launch(false, false, true); Others.Wait(2000); Logging.Write("Bobber GUID = "+Fishing.SearchBobber()); Lua.LuaDoString("SpellStopCasting"); Others.Wait(100); }
Write what you see in log. Is there GUID value every time?
-
1 hour ago, xerian said:
Someone resolved problem?
Resolved with battleground helper, but in some cases wManager.dll requires patch (if AcceptBattlefieldPort is disabled on server)
-
I think you can call GetInstanceInfo.
For example, if you use lua events listener (i never tried, but maybe it possible in grinding profile too), you can call it after PLAYER_ENTERING_WORLD event:
private void MyLuaHandler(LuaEventsId id, List<string> args) { switch (id) { case LuaEventsId.LFG_PROPOSAL_SHOW: Lua.LuaDoString("AcceptProposal()"); break; case LuaEventsId.PLAYER_ENTERING_WORLD: StartInstance(); break; default: break; } } private void StartInstance() { string[] dungeonInfo = Lua.LuaDoString<string[]>("return GetInstanceInfo()"); // name=dungeonInfo[0] type=dungeonInfo[1] difficulty=dungeonInfo[2] ... if (dungeonInfo[1] == "party") { // here you can check dungeon name switch (dungeonInfo[0]) { case "Gruul's Lair": start_quest_1 break; case "another name": start_quest_2 break; ... default: break; } } }
-
There are alot of LFG functions and LFG events (check started with "LFG"). Read documentation, try functions in developer tools, and write code like in pattern above. Much of functions and events undocummented, maybe you can find better source with google
-
you can try to make auto accept dungeon plugin, like i did in Battleground helper.
For this you have to listen Lua event LFG_PROPOSAL_SHOW, and when it fires, call AcceptProposal() function, or, if it disabled, click accept button programmatically. To discover name of button, type /fstack command, join dungeon queue, and when confirm dialog popup, put cursor above button and read it name.
Plugin code should be like this:
using wManager.Wow.Enums; using wManager.Wow.Helpers; using System.Collections.Generic; public class Main : wManager.Plugin.IPlugin { public void Initialize() { EventsLuaWithArgs.OnEventsLuaWithArgs += MyLuaHandler; } public void Dispose() { EventsLuaWithArgs.OnEventsLuaWithArgs -= MyLuaHandler; } private void MyLuaHandler(LuaEventsId id, List<string> args) { if (id == LuaEventsId.LFG_PROPOSAL_SHOW) { Lua.LuaDoString("AcceptProposal()"); // or Lua.LuaDoString("Button_name_you_found:Click()"); } } public void Settings() { // no settings } }
Of course, you can handle other events too (like invite accept and role select)
-
Работает это так: бот проверяет качество вещей в сумках, и продает те, на которых галочка. При этом, независимо от качества, не продаются те, которые в списке Do not Sell, и продаются те, которые в списке Force Sell.
Названия в списках должны в точности соответствовать тому, как они пишутся в WoW
Медная руда - для русского клиента
Copper Ore - для английского
-
Условия на самом деле разные, посмотри в самый конец. Ну а код - пипец. Вместо этих 4 строчек в скобочках используй метод с сигнатурой:
public void Launch(bool stopMove, bool waitIsCast, bool ignoreIfCast, bool castOnSelf)
Думаю, с аргументами тут всё должно быть понятно.
Regen - это время поесть/попить, если задано. Поищи в настройках
-
-
Есть заготовка плагина для БГ. Делает примерно то же, что и аддон BattlegroundTargets, но в роботе: определяет состав и роли в группе противника. Все это складывает в таблицу, которой можно дальше пользоваться. Например, атаковать в первую очередь хилеров. Пока что просто спамит в инстанс чат количество противников и кто у них хилер (настраивается).
Интересует кого такая штука или пофиг?
Насчет атаки - нужна только идея, на какое событие посадить выбор противника
-
Does it write "confirm" and other states to log and WoW console?
If you think it clicks too fast simply add string
System.Threading.Thread.Sleep(100); // or another value if 100 is not enough
in the BattlefieldStatusHandler method, before string
Lua.LuaDoString("PVPReadyDialogEnterBattleButton:Click()");
-
13 hours ago, Alex007 said:
Same situation
I uploaded new version, try to use LUA callback.
In settings set spam=True to make sure plugin works.
Also, try use wrotation and join to battleground queue manually - does it auto accepts BG?
-
try this:
Gathering herbs while using quest profile
in WRobot for Wow Vanilla - Help and support
Posted
Does it works with normal latency?
This C# code is approximate algorythm from old version of wrobot (i used 7.1.5 archieve available on this server). Now it obfuscated and almost impossible to say what it doing. Maybe there is some errors there, maybe some plugin or profile code interrupts herb gathering. Try to find older version of wrobot