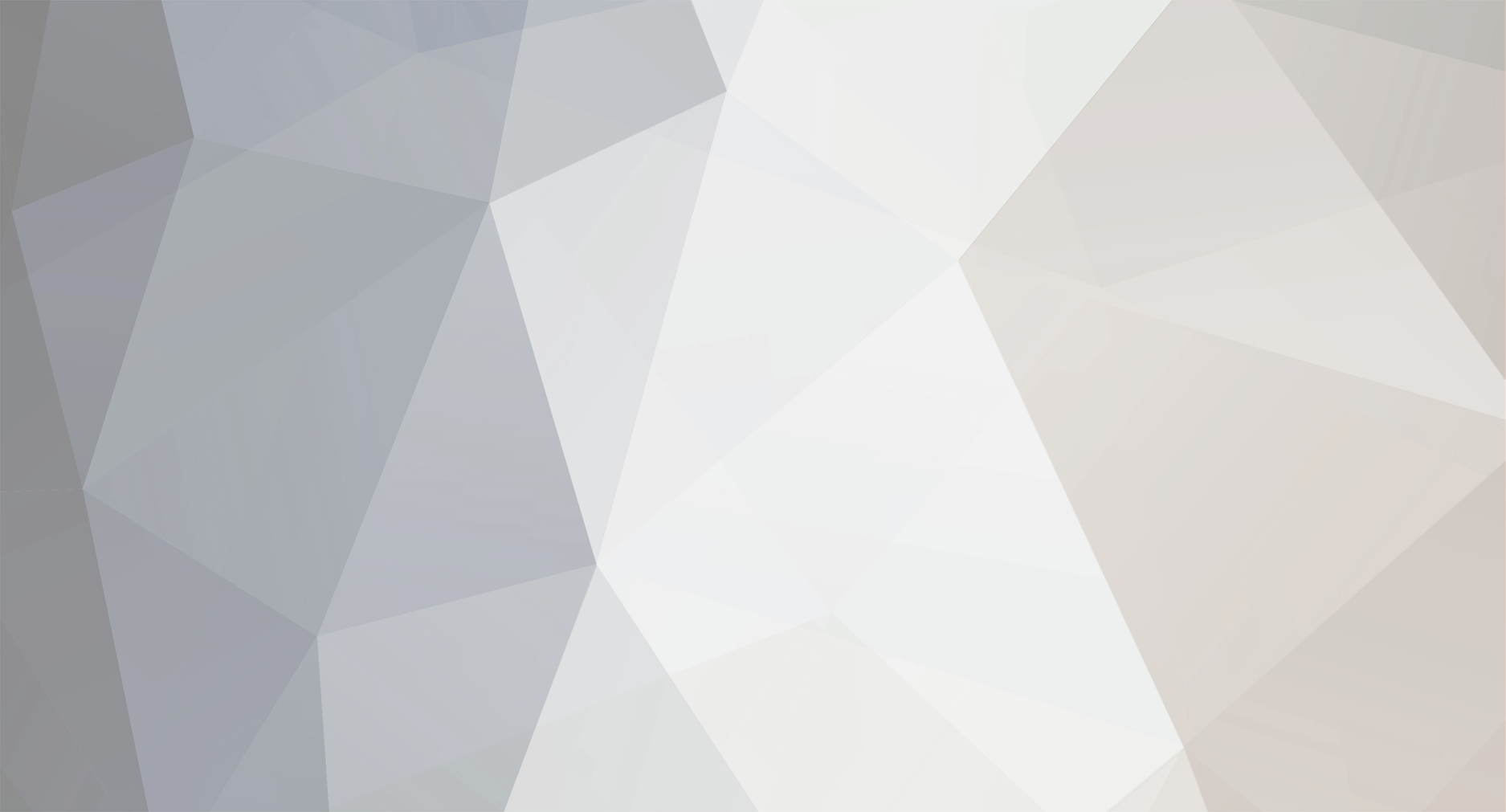
Pasterke
-
Posts
165 -
Joined
-
Last visited
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Posts posted by Pasterke
-
-
-
Thx, will look at it when I have some time. I'm retired, so I almost have no time :)
-
public string LastCast {get; set; }
if (LastCast != "Lava Lash" .....
And put everywhere in your spells : LastCast = spell.name;
-
On what private server are you guys playing ? And is it worth to join ?
-
25fps is a realy good choice. It's not for a reason that it's the default. Higher than 30fps is not recommended. Look on google and search for fps and wow, it will be explained in details.
If you have a long list with spells, it realy don't matter how you order your spells.
The bot looks at your spells, and go down from top to bottom, and then start over again. If a skill is ready, he use it. This process is soo fast, that the order of your spells is not relevant.
And that's the reason why the bot don't do things at the right moment.
In his memory he keep the true actions. So when he starts over from the beginning, he will do the true actions from his memory and add new true conditions to this list.
You never have a skill for the actually time. That's why it's hard to get a good healing class for this bot.
The only solution to this problems, is working with coroutines, what they did in Honorbuddy.
Droidz should look at the coroutines section of the Honorbuddy wiki to implent such a system.
That's why wrobot will never compete with honorbuddy in terms of dps.
I wrote alot of Routines for Honorbuddy with the same logic as I write routines for wrobot.
for example : my honorbuddy routine for feral druid and the routine for feral druid in wrobot, the difference in dps is like 12k in favor of Honorbuddy. That's huge.
-
That's the c# programming language, not scripts.
Install visual studio (the free version) and learn how to work with it. It's not that difficult :)
You can load a .cs files in visual studio and see the code. With intellisense it helps you alot to add or change code.
- leetdemon, BetterSister and Dashia
-
3
-
Automaton is much safer to use than a grinder profile in terms to get get caught for botting.
What are good places for Automaton, that's quit simple :
are you full heirloom : you can handle mobs that are 3 levels higher than yours. Quit the place if your level is 3 levels higher than the mobs level.
no heirlooms : areas where mobs are with 1 level higher than yours, leave area if your level is 4x higher than mob level.
With automaton you don't follow a pre-defined route, so you don't come back to the previous location to continue the profile. It's less bottish.
A good tip : for leveling take a profession like skinning, you will make alot of money. (my hunter had 32k gold on level 60)
look at http://www.wow-professions.com/wowguides/wow-skinning-guide.html for the right places to be.
A secondary good profession is herbalism. You can gather herbs on the places where you farm leathers. Herbs also selling good on AH.
By farming on those places you have a chance to get rare drops like azure whelping and others that sells easy on AH between 5k and 15k.
If you reach level 90, then if you want, you can drop those professions and choose others that you realy want. If you quest a little you get drops and quests that brings your profession skills to 600.
That way, it's easier to gather the materials for your profession afterwards.
-
Ok, here you go
public static List<WoWUnit> getPartyPets()
{
Vector3 myPos = ObjectManager.Me.Position;
var ret = new List<WoWUnit>();
var pets = ObjectManager.GetObjectWoWUnit().Where(p => p != null
&& p.IsPet).ToList();
foreach (var m in pets)
{
if (m.IsValid && m.IsAlive && m.InCombat && m.Target.IsNotZero() && m.Position.DistanceTo2D(myPos) <= 40 && !TraceLine.TraceLineGo(m.Position))
{
if (ret.All(u => u.Guid != m.Target))
{
var targetUnit = new WoWUnit(ObjectManager.GetObjectByGuid(m.Target).GetBaseAddress);
if (targetUnit.IsValid && targetUnit.IsAlive)
{
ret.Add(targetUnit);
}
}
}
}
return ret;
}
public static List<WoWUnit> GetPartyTargets()
{
Vector3 myPos = ObjectManager.Me.Position;
var partyMembers = Party.GetPartyHomeAndInstance();
var ret = new List<WoWUnit>();foreach (var m in partyMembers)
{
if (m.IsValid && m.IsAlive && m.InCombat && m.Target.IsNotZero() && m.Position.DistanceTo2D(myPos) <= 40 && !TraceLine.TraceLineGo(m.Position))
{
if (ret.All(u => u.Guid != m.Target))
{
var targetUnit = new WoWUnit(ObjectManager.GetObjectByGuid(m.Target).GetBaseAddress);
if (targetUnit.IsValid && targetUnit.IsAlive)
{
ret.Add(targetUnit);
}
}
}
}
return ret;
}public static int partyCount { get { return GetPartyTargets().Count(); } }
public static bool buffExists(WoWUnit unit, string buff)
{
return unit.HaveBuff(buff);
}public static bool petBuffExists(string buff)
{
return ObjectManager.Pet.HaveBuff(buff);
}public HashSet<string> statsBuffs = new HashSet<string>()
{
"Mark of the Wild",
"Blessing of Kings",
"Gift of the Wild"
};public bool checkBuff(WoWUnit unit)
{
var a = unit.GetAllBuff();
foreach(var aura in a)
{
if (statsBuffs.Contains(aura.ToString())) return false;
}
return true;
}public bool needStatsBuff(string buff, bool reqs)
{
if (!reqs) return false;
foreach(WoWUnit t in GetPartyTargets())
{
if (!buffExists(t, buff) && !checkBuff(t))
{
try
{
SpellManager.CastSpellByNameOn(buff, t.Name);
Logging.WriteFight(buff + "on " + t.Name);
return true;
}
catch(Exception e) { Logging.WriteFight("Single StatsBuffs: " + e.Message); }
}
}
return false;
}
public bool petsNeedStats(string buff, bool reqs)
{
if (!reqs) return false;
foreach (WoWUnit t in getPartyPets())
{
if (!buffExists(t, buff) && !checkBuff(t))
{
try
{
SpellManager.CastSpellByNameOn(buff, t.Name);
Logging.WriteFight(buff + "on " + t.Name);
return true;
}
catch (Exception e) { Logging.WriteFight("Pet StatsBuffs: " + e.Message); }
}
}
return false;
}
private Spell motw = new Spell("Mark of the Wild");
public void Routine()
{
if (partyCount > 0 && needStatsBuff("Mark of the Wild", motw.KnownSpell && motw.IsSpellUsable)) return;
if (partyCount > 0 && petsNeedStats("Mark of the Wild", motw.KnownSpell && motw.IsSpellUsable)) return;
}To check for other buffs make a new hashset and new bools, eg. needFortitude, petsNeedFortitude
-
But Motw and Power Word; Fortitude does. And with a range class, never use interact + launch, because using that wil try the toon to get in meleerange. Use SpellManager.CastSpellByNameLUAOn(name spell, name target);
-
don't matter if it's 3.3.5, the code checks if you are in a party and if some1 doesn't have a stats buff, if not, cast it on yourself, and the others in your party get it also. Even buffs from MoP or Draenor, don't matter, they will not have it and cause no error.
-
You don't need to cast it on the target, if you cast it on yourself, then everyone get the buff. Don't make things too complicate.
private Spell MoTW = new Spell("Mark of the Wild");
public bool needMotw(bool reqs)
{
if (!reqs) return false;
if (!MoTW.KnownSpell) return false;
if (!MoTW.IsSpellUsable) return false;
var t = GetPartyMembers().Where(p => p != null&& p.GetDistance <= 40
&& !p.HaveBuff(MoTW.Name)
&& !p.HaveBuff("Legacy of the Emperor")
&& !p.HaveBuff("Blessing of Kings")
&& !p.HaveBuff("Lone Wolf: Power of the Primates")
&& !p.HaveBuff("Bark of the Wild")
&& !p.HaveBuff("Blessing of Kongs")
&& !p.HaveBuff("Embrace of the Shale Spider")
&& !p.HaveBuff("Strength of the Earth"));
if (t != null)
{
try
{
SpellManager.CastSpellByNameOn(MoTW.Name, ObjectManager.Me.Name);
Logging.WriteFight(MoTW.Name);
return true;
}
catch (Exception e)
{
Logging.WriteFight("MotW: " + e.Message);
}
}
return false;
}Then just look if you need it :
if (needMotw(Party.GetPartyNumberPlayers() > 0)) return true;
-
You don't use a flying mount. If you use an flying mount profile you can run into troubles, because the bot is unable to reach some nodes.
As druid you should use Travel Form.
-
Maybe your wow settings ?
-
When using Automaton, wrobot crashes. When choosing close the program, wow also crash.
-
Check if de debuff exists Void Tendrils. If it exists, while your distance to the target smaller than x, don't cast any spell.
-
-
The only possibility to make a heal class is in C#. You can't make a healer routine with the fightclass creator. That's why it's called fightclass :)
-
The worst thing you can do is add Barrier to the rotation. Normaly, the raid leader will instruct the healers when to use such a cooldown.
-
Depends if it's your 1st char. If you already have mounts, you can use one of them. Ofc you need to learn mount skill, for Alliance in SW, for Horde in Ogrimmar, Trainers are near the Flight Master.
-
Select Gatherer.
Product Settings
Profile Creator
Set Record Path on on. Minimize Window.
Usefull addon : Gathermate2 and Gathermate2_data.
In addon config Gathermate2 choose import (check what you want to import)
Open your map and put it in mini mode.
You will see the ores on your map.
Now fly araound making a circle (you end where you start)
If you reach your startpoint again, maximize Profile Creator.
Hit the save button, and give it a name.
Close Profile Creator.
Load your just created profile.
Hit start.
Realy easy to create your own profile.
- Droidz and BetterSister
-
2
-
If you have an level 100 and you can fly in draenor, then you need no profiles to level 90-100. Just get the treasures and the bonus quests for each zone.
-
It's only the starting area quests that are different for each race.
Once they are level 5, move them to a capital city and start a quest line for Alliance or Horde.
example Alliance : goto SW, pick up Eversong Wood Quests, Westfall Quests, Redridge Mountain Quests, Duskwood Quests, Strangleton Quests, Western(35-40) + Eastern Plaguelands Quests (40+), Burning Steppes, Swamp of Sorrow, Blasted Lands.
You setup the same quest line for each faction, it's easier than setup different quest lines for each race.
-
Since WoD, Mangle is Bear Form only. Look in your spell book, need Bear Form !
-
If you play WoW on an Linux or FreeBSD machine, you have to run WoW in 32-bit mode, otherwise you run into problems with wine.
Only older computers can only run in 32 bit mode, but I think that playing WoW on such an older computer will be an pain in the ...
Running an windows 32 bit version limits you in memory use, only 4GB of memory is available.
I also think it's time to move to an 64-bits bot, but who am I ? :)
A few questions for succesful wotlk botters
in WRobot for Wow Wrath of the Lich King - Help and support
Posted
Ok, I got an account on warmane and when making an new char, it was already level 80.
When I try to make a new one, I get an character create error.
How can you level up a charcater ?