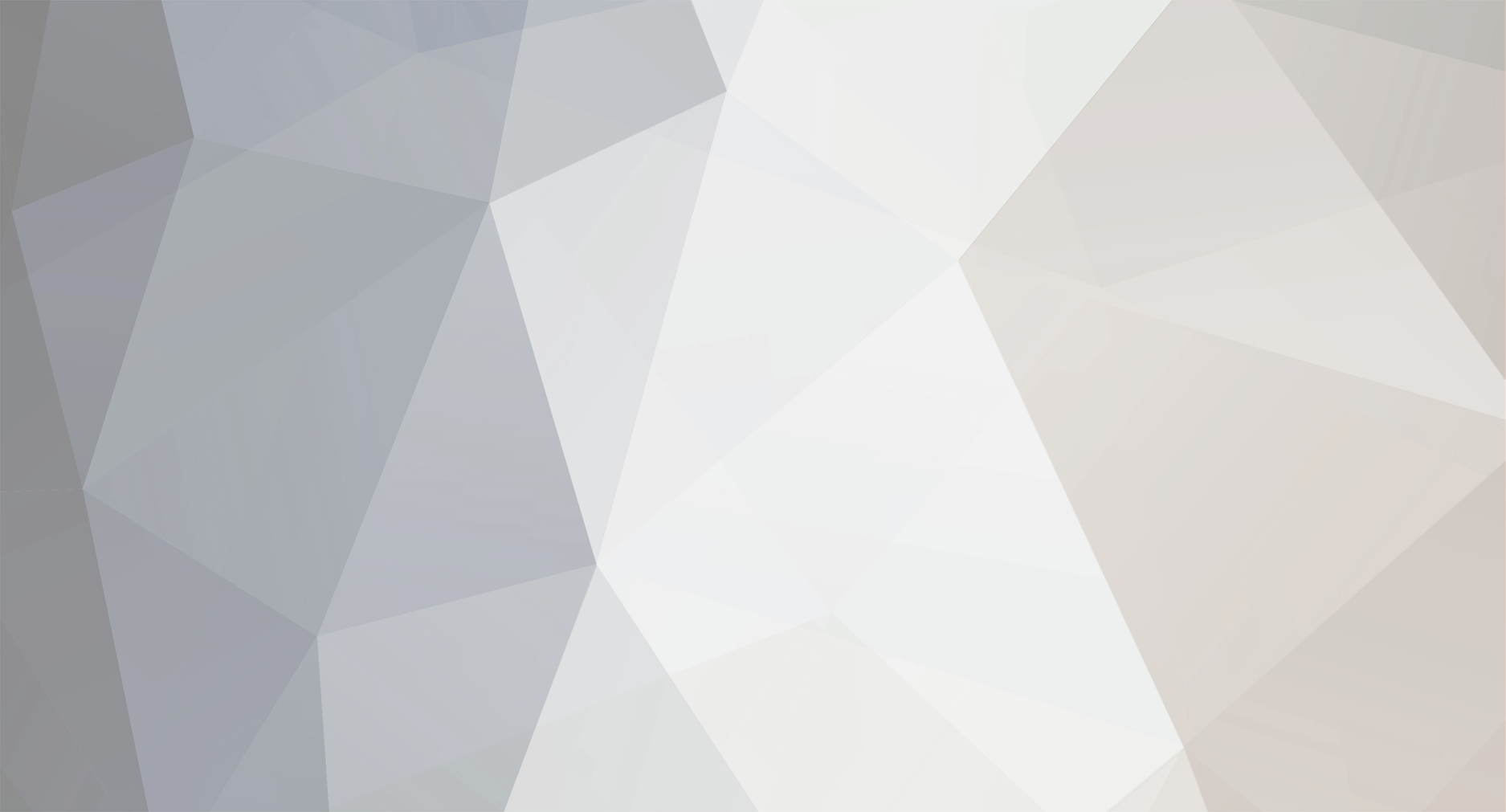
Talamin
-
Posts
329 -
Joined
-
Last visited
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Posts posted by Talamin
-
-
you can try the AIO
-
-
You can make something like this:
public static bool DebuffCheckOwner(Spell spell, bool owner = true) { List<Aura> DebuffList = ObjectManager.Target.GetAllBuff(); Aura aura = null; if(owner) { aura = DebuffList.FirstOrDefault(s => s.GetSpell.Name == spell.Name && s.Owner == ObjectManager.Me.Guid); if(aura != null) { return true; } } return false; }
-
Dann poste doch mal deine Settings bitte ?
-
Also bei der AIO WOTLK Fightclass castet er so lange bis er 20 Wasser hat und danach verbraucht er diese. Hast du da das gleiche Problem?
-
-
Bump again, just to be sure ?
-
Wenn du auf einem Privat Server spielst auf dem eine Version läuft die Wrobot unterstützt, dann ja.
-
You can build a List with all Party Members and then try to check each one of them:
public static bool GroupCureSpellPoison(Spell spell) { if (!spell.KnownSpell || !spell.IsSpellUsable || !spell.IsDistanceGood || ObjectManager.Me.HaveBuff("Drink") || ObjectManager.Me.HaveBuff("Food")) { return false; } IEnumerable<WoWPlayer> members = Partystuff.getPartymembers().Where(o => o.IsAlive); if (members.Count() > 0) { foreach (WoWPlayer member in members) { if (!TraceLine.TraceLineGo(member.Position) && HasPoisonDebuff(member)) { Spells.Focus(spell, member); } } } return false; }
-
-
-
wManager.Wow.Bot.Tasks.GoToTask.ToPositionAndIntecractWithNpc(new Vector3(1111.1f, 2222.2f, 3333.3f), 12345);
1111 = x Vector of NPC, 2222 = y Vector of NPC, 3333 = z Vector of NPC, 12345 = ID of NPC.
and then:
wManager.Wow.Bot.States.ToTown.ForceToTown = true;
and after you are done with your Custom Townrun:
wManager.Wow.Bot.States.ToTown.ForceToTown = true;
-
oh ok, never have seen this ?
-
I am not 100% sure if this isn´t implemented, but last time i checked questing wrobot wasn´t able by default to pickup a Quest reward. You can use an addon to achieve this, use a Plugin or buy HMP from Matenia.
-
Don´t use a modified client, download one on another side.
-
Do you get some kind of Buff? If not you could check if you have a Pet with the given name.
-
Talk to @TheSmokie, he released the Profile and can give you an answer, or post in the Thread where you downloaded the Profile.
-
Maybe take a look at the scripts from @ScripterQQ who made some Fightclasses with the Editor.
-
Ah sorry, thought you were making a complete FIghtclass in C#. I never used the Fightclass Editor, so i really don´t know hoa to build this there. I am sure that you can catch if the Target has already the Buff then ignore it.
-
Sorry, but it´s hard to understand what exactly you mean.
If you have a Function which already checks all Party Members then you can use something like to cast only if :
WoWUnit Bufftarget = ObjectManager.GetObjectWoWUnit.Where(o=> o.IsAlive && !o.HaveBuff("Buff") && o.Name == "player-name" && o.IsPartyMember).FirstOrDefault(); Interact.InteractGameObject(Bufftarget.GetBaseAddress); Buff.Launch(); Usefuls.WaitIsCasting();
Or using the same but casting on Focustarget:
WoWUnit Bufftarget = ObjectManager.GetObjectWoWUnit.Where(o=> o.IsAlive && !o.HaveBuff("Buff") && o.Name == "player-name" && o.IsPartyMember).FirstOrDefault(); ObjectManager.Me.FocusGuid = Bufftarget.Guid; Lua.LuaDoString($"CastSpellByID({ Buff.Id}, \"focus\")"); Usefuls.WaitIsCasting();
And complete:
if(Me.IsInGroup) { WoWUnit Bufftarget = ObjectManager.GetObjectWoWUnit.Where(o=> o.IsAlive && !o.HaveBuff("Buff") && o.Name == "player-name" && o.IsPartyMember).FirstOrDefault(); ObjectManager.Me.FocusGuid = Bufftarget.Guid; Lua.LuaDoString($"CastSpellByID({ Buff.Id}, \"focus\")"); Usefuls.WaitIsCasting(); Lua.LuaDoString("ClearFocus();"); }
It would be helpful if you just post the Code you are struggling with.
-
And this Topic is 1 1/2 year old, why you necroing it?
-
public static void DeleteItems(string itemName, int leaveAmount = 0) { var itemQuantity = ItemsManager.GetItemCountByNameLUA(itemName) - leaveAmount; if (string.IsNullOrWhiteSpace(itemName) || itemQuantity <= 0) { return; } string luaToDelete = $@" local itemCount = {itemQuantity}; local deleted = 0; for b=0,4 do if GetBagName(b) then for s=1, GetContainerNumSlots(b) do local itemLink = GetContainerItemLink(b, s) if itemLink then local itemString = string.match(itemLink, ""item[%-?%d:]+""); local _, stackCount = GetContainerItemInfo(b, s); local leftItems = itemCount - deleted; if ((GetItemInfo(itemString) == ""{itemName}"") and leftItems > 0) then if stackCount <= 1 then PickupContainerItem(b, s); DeleteCursorItem(); deleted = deleted + 1; else if (leftItems > stackCount) then SplitContainerItem(b, s, stackCount); DeleteCursorItem(); deleted = deleted + stackCount; else SplitContainerItem(b, s, leftItems); DeleteCursorItem(); deleted = deleted + leftItems; end end end end end end end "; Lua.LuaDoString(luaToDelete); }
Directly out of Matenias Framework, and with DeleteItems("Soulshard", 5) usable.
-
-
Hilfe zu AOE - Soll automatisch rausrennen
in Deutsch
Posted
Ich glaube das es nicht wirklich ein freies Plugin für so etwas gibt. Ich habe mal gesehen das jemand genau ein solches Plugin entwickelt hat, allerdings ist es nie public geworden, von daher stehen die Chancen dafür im Moment eher schlecht.