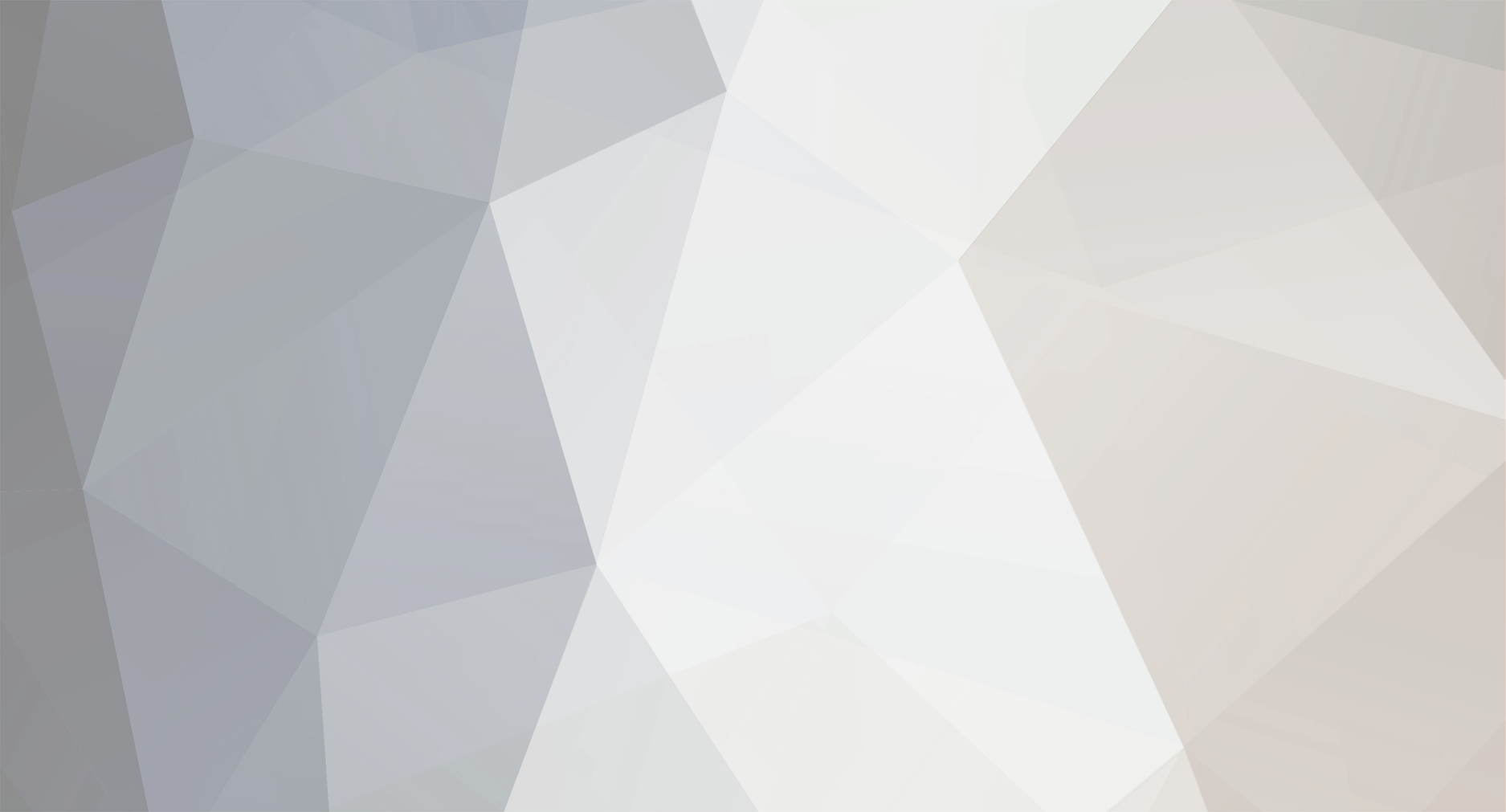
Matenia
-
Posts
2232 -
Joined
-
Last visited
Content Type
Forums
Articles
Bug Tracker
Downloads
Store
Bug Report Comments posted by Matenia
-
-
Problem found: I was using Me.ForceIsCast = true and it never actually reset
-
It's not related to either code snippets - although they both stop working.
I've determined it only happens with one fightclass. However, the fightclass itself does not touch movement.
I think it might be related to Lua because in combat I've gotten the Lua error "stackoverflow, table too big to unpack". -
Caused by this:
in Aura.cs
-
????
in Bug Tracker
-
It's possible fairly easily, as the quest type already uses a grinder under the hood. But creating the path recorder is more complicated.
I suggest you record the path in the grinder product and just copy over the waypoints. WRobot's code basically creates a path between all hotspots using the pathfinder. -
//This is how I handle it in my very own fight mechanism: _fightStartTs = DateTime.Now; // chase target if necessary, otherwise wait while (Conditions.InGameAndConnectedAndAliveAndProductStartedNotInPause && _target.IsAlive && _fightTrigger && !_target.IsTapDenied) { KeepTarget(_targetGuid); //MoveAwayFromEnemies(); // needs to know if enemy is caster KiteIfNecessary(_target); SwimUpForAir(); PotionUser.UsePotions(); ChaseTarget(_target, () => ClassRotation.Range); HandleEvade(_target); EscapeIfNecessary(); Thread.Sleep(150); } private static void SwimUpForAir() { if (AntiDrown.NeedsAirInCombat) { AntiDrown.GaspForAir(); } } using System; using System.Collections.Generic; using System.Threading; using robotManager.FiniteStateMachine; using SmartRobot.Bot.Helpers; using wManager.Wow.Enums; using wManager.Wow.Helpers; using wManager.Wow.ObjectManager; namespace SmartRobot.Bot.States { public class AntiDrown : State { private static DateTime _breathExpires = DateTime.MaxValue; static AntiDrown() { EventsLuaWithArgs.OnEventsLuaStringWithArgs += AntiDrownEventHandler; } public override string DisplayName => "AntiDrown"; private bool NeedsAir => DateTime.Now.AddSeconds(30) > _breathExpires; public static bool NeedsAirInCombat => DateTime.Now.AddSeconds(15) > _breathExpires; public static bool IsBreathing => _breathExpires != DateTime.MaxValue; public override bool NeedToRun => Conditions.InGameAndConnectedAndAliveAndProductStartedNotInPause && ObjectManager.Me.IsSwimming && NeedsAir; ~AntiDrown() { EventsLuaWithArgs.OnEventsLuaStringWithArgs -= AntiDrownEventHandler; } private static void AntiDrownEventHandler(string id, List<string> args) { if ((id == "MIRROR_TIMER_START") & (args[0] == "BREATH")) { //sets BreathExpires to be in the future by x milliseconds var expiryDate = DateTime.Now.AddMilliseconds(double.Parse(args[1])); _breathExpires = expiryDate; if (!ObjectManager.Me.HaveBuff("Water Breathing")) { ItemsManager.UseItem(5996); } } if (id == "MIRROR_TIMER_STOP") { _breathExpires = DateTime.MaxValue; } } public override void Run() { GaspForAir(); } public static void GaspForAir() { Logger.Info("Swimming up for air"); MovementManager.StopMove(); while (Conditions.InGameAndConnectedAndAliveAndProductStartedNotInPause && IsBreathing && ObjectManager.Me.IsSwimming) { Move.JumpOrAscend(Move.MoveAction.DownKey, 1000); Thread.Sleep(500); } // wait at surface while (Conditions.InGameAndConnectedAndAliveAndProductStartedNotInPause && IsBreathing && !Common.IsAttackedByNpc) { Thread.Sleep(500); } } } }
Hope it helps for inspiration.
-
-
Warmane, correct? I swear this happened with people where changing focus target/casting on focus would stop working after a while. I had complaints about that with some of my fightclasses.
-
-
Yeah, I figured if I'm suspending the CustomClassThread while it's writing to client memory (not allowing it finish whatever work it was doing) it would either deadlock wRobot or the wow client itself (through directx endscene), while I was testing some things.
I decided it's time I write my own customclass implementation that allows me to properly pause it from an outside thread and provides more customizability than what wRobot currently offers.
Thanks for the insight though, that was very helpful. -
I think it has do to with me suspending CustomClass.Thread, like this:
private void FightClassWaitForRegen() { List<Thread> threadsToSleep = new List<Thread>(); threadsToSleep.Add(CustomClass.CustomClassThread); var fightClassSleepThread = SleepFightClassThread(threadsToSleep); WaitUntilRegenerated(threadsToSleep); fightClassSleepThread.Abort(); } private Thread SleepFightClassThread(List<Thread> threadsToSleep) { Thread fightClassSleepThread = new Thread(() => { try { foreach (var thread in threadsToSleep) { thread.Suspend(); } while (Conditions.InGameAndConnectedAndAliveAndProductStarted && !Common.IsAttackedByNpc) { Thread.Sleep(500); } } finally { foreach (var thread in threadsToSleep) { thread.Resume(); } } }); fightClassSleepThread.Start(); return fightClassSleepThread; }
-
Similar issue:
Wow stays unfrozen, but wRobot (interface too) is frozen.
When I force-kill wRobot.exe process, WoW throws this error:ERROR #132 (0x85100084) Fatal Exception Program: F:\Games\WoW 3.3.5\Wow.exe Exception: 0x80000004 (SINGLE_STEP) at 0023:66D54590
I think it happens when wRobot targets itself (or others) using Interact.InteractGameObject
-
No, I do not. But I know you do (CastSpellByName).
I think that is causing it eventually to be corrupted.
It's just a guess because someone CastSpellByName stops working after a while on WotLK. -
Also if you know a better way to pause fightclass thread than this, let me know:
private Thread SleepFightClassThread(List<Thread> threadsToSleep) { Thread fightClassSleepThread = new Thread(() => { try { foreach (var thread in threadsToSleep) { thread.Suspend(); } while (Conditions.InGameAndConnectedAndAliveAndProductStarted && !Common.IsAttackedByNpc) { Thread.Sleep(500); } } finally { foreach (var thread in threadsToSleep) { thread.Resume(); } } }); fightClassSleepThread.Start(); return fightClassSleepThread; }
-
Hello @Droidz,
I found the issue, I think. It's a multi threading issue, I think.
If you call Lua.LuaDoString twice within the same framelock there are some Lua variables that get restored to their incorrect state.
Pseudo code:
-- first thread local _protectedFunctionSave = protectedFunction; protectedFunction = function(abc) return expectedValueForAntiCheat; end -- do actual lua code here -- !first thread -- before this function is restored another thread comes and does this -- second thread: local _protectedFunctionSave = protectedFunction; -- this is now the wrong function we are saving protectedFunction = function(abc) return expectedValueForAntiCheat; end -- !second thread -- first thread protectedFunction = _protectedFunctionSave; -- original is restored correctly -- !first thread -- second thread -- do actual lua code here protectedFunction = _protectedFunctionSave; -- wrong function is restored and breaks client a bit -- !second thread
Is this possible? If yes, maybe thread-lock Lua class?
-
Yes, thank you. I noticed this is a different issue that seems to taint some Lua functions on Warmane somehow. I cannot reproduce it reliably, so for now it's okay.
-
Update: It seems this actually works... It's weird, sometimes it seemed to break.
I'd write the mouseover guid, call Lua.LuaDoString($"CastSpellByName('{name}', 'mouseover')") and nothing happens. It seems almost like the WoW client breaks for a bit. Happens on Warmane only I guess and I can't really reproduce it. -
Problem here was that if you don't activate Use Lua to Move, it causes endless game freezes
-
No, he means someone killed the vendor and wRobot tries to run back to it.
-
You're starting an encrypted profile with what I can only assume is maybe a trial version of wRobot. Anyway, it's more up to the profile than Droidz to fix this (which was created by a user who has since left).
-
Yes, no other Subway gameobject exist.
-
On WotLK: http://wotlk.cavernoftime.com/?search=Subway (all called Subway)
On TBC: http://tbc.cavernoftime.com/?search=Subway (same, all called Subway)
On Vanilla: https://classicdb.ch/?search=Subway (same)
It's 176080 to 176086 - each CART in the tram (2 trams) is one id.
-
-
Droidz - run 2-3 wRobot sessions at the same time. People have said if you do that it happens much faster
[Fight] Chases targets with wrong LoS flags
in Bug Tracker
Posted
I will try to create a video. It happened mostly in Arathi Basin (battleground) where other players would kite my character around a cart and other small objects.