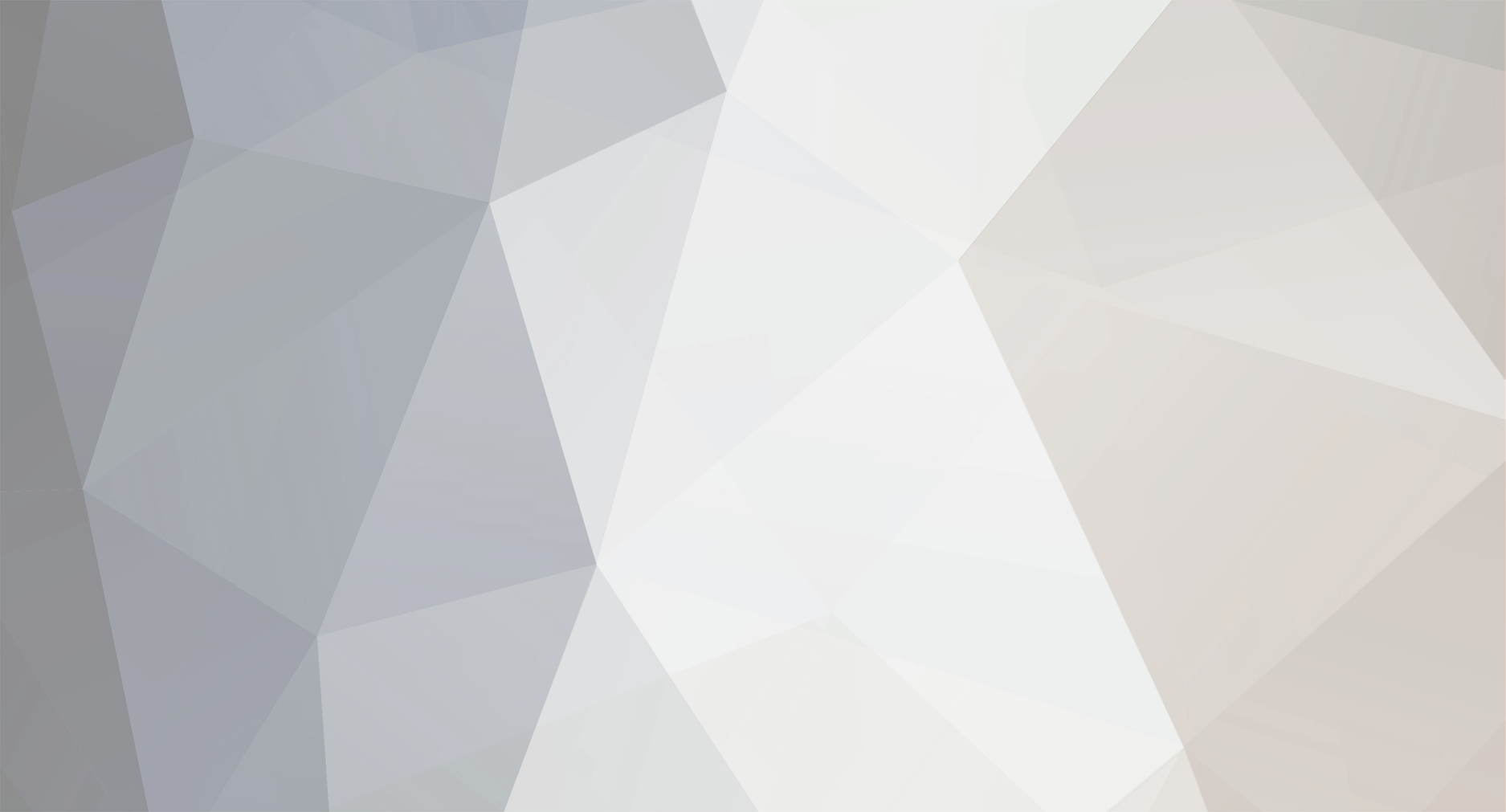
Matenia
-
Posts
2232 -
Joined
-
Last visited
Reputation Activity
-
Matenia reacted to Droidz in Close game when Teleported --> Stop bot when Teleported
Hello, I am not sure to understand what you want but:
robotManager.Events.FiniteStateMachineEvents.OnRunState += delegate(robotManager.FiniteStateMachine.Engine engine, robotManager.FiniteStateMachine.State state, System.ComponentModel.CancelEventArgs cancelable) { if (state is wManager.Wow.Bot.States.StopBotIf && state.DisplayName == "Security/Stop game") { // sample to disable tp detection: wManager.wManagerSetting.CurrentSetting.CloseIfPlayerTeleported = false; wManager.Wow.Bot.States.StopBotIf.ForcePvpMode = true; wManager.Wow.Bot.States.StopBotIf.LastPos = wManager.Wow.ObjectManager.ObjectManager.Me.Position; // how to wrobot check if your char is tp: if (wManager.Wow.Bot.States.StopBotIf.LastPos != null && wManager.Wow.Helpers.Conditions.InGameAndConnectedAndAlive && wManager.Wow.ObjectManager.ObjectManager.Me.Position.DistanceTo(wManager.Wow.Bot.States.StopBotIf.LastPos) >= 450) { // close bot... } } };
-
Matenia reacted to reapler in Left click unit
It's also possible to do this on vanilla, but with mouseover guid:
public void Target(ulong guid) { if (guid == 0) return; ulong tmp = wManager.Wow.Memory.WowMemory.Memory.ReadUInt64( (uint) wManager.Wow.Memory.WowMemory.Memory.MainModuleAddress + 0x74E2C8); wManager.Wow.Memory.WowMemory.Memory.WriteUInt64( (uint) wManager.Wow.Memory.WowMemory.Memory.MainModuleAddress + 0x74E2C8, guid); wManager.Wow.Helpers.Lua.LuaDoString("TargetUnit('mouseover');"); wManager.Wow.Memory.WowMemory.Memory.WriteUInt64( (uint) wManager.Wow.Memory.WowMemory.Memory.MainModuleAddress + 0x74E2C8, tmp); }
-
Matenia got a reaction from Skygirl779 in Left click unit
A SPECIFIC unit? You can take a look at (decompile) how Droidz does it in the "Interact" class in the Helper namespace (lots of memory writing).
If not, use
Lua.LuaDoString(TargetEnemy("name")) (I think) or
Lua.RunMaroText("/target name")
-
Matenia reacted to Seminko in Prioritize Low HP targets
You need an OnFightLoop fight event.
I'm not sure whether this is the correct way to handle events but it's the way I could come up with, since my knowledge of Csharp is very limited still... BUT, what I do is put the OnFightLoop event in a method and call it during initialization. Once it is called the events "are recognized" and will fire everytime you are in a fight loop.
Disclaimer: this is not tested, put this together just now...
public void TargetSwitcher() // needs to be called just once, ie during Initialize { FightEvents.OnFightLoop += (unit, cancelable) => { // this code will loop everytime you are fighting List<WoWUnit> attackers = ObjectManager.GetUnitAttackPlayer(); // gets all units attacking you if (attackers.Count > 1) // if you are attacked by more than one mob { Logging.WriteDebug("More than 1 attackers detected."); //WoWUnit highestHP = attackers.OrderBy(uo => uo.HealthPercent).LastOrDefault(); // sort the list based on HP from lowest to highest, pick highest WoWUnit lowestHP = attackers.OrderBy(ou => ou.HealthPercent).FirstOrDefault(); // sort the list based on HP from lowest to highest, pick lowest if (lowestHP != null && lowestHP.IsValid && lowestHP.IsAlive && !lowestHP.IsMyTarget) // if the lowest hp mob is valid, alive and NOT your current target { cancelable.Cancel = true; // not TOO sure about this one haha Interact.InteractGameObject(lowestHP.GetBaseAddress); // switch to it Fight.StartFight(lowestHP.GetBaseAddress); // start fighting it Logging.WriteDebug("Switched to lowestHP target."); } } }; }
As a bonus, check this post by Droidz. Might be usefull too.
Let me know how it worked out for ya...
-
Matenia got a reaction from BetterSister in All my bots on Warmane Outland got banned
They probably finally started tracking popular Outland locations. Feral farming is pretty much just SCREAMING to get banned.
-
Matenia reacted to Droidz in Specific vendor in profile
Hello,
You cannot with default, but if you use custom code (plugin in this sample):
using System; using System.Collections.Generic; public class Main : wManager.Plugin.IPlugin { public void Initialize() { // NPC list var npcs = new List<Tuple<int, int, wManager.Wow.Class.Npc>> // Item1=minlevel, Item2=maxlevel, Item3=npc { new Tuple<int, int, wManager.Wow.Class.Npc>(1, 5, new wManager.Wow.Class.Npc { Type = wManager.Wow.Class.Npc.NpcType.Repair, Name = "Npc name for level 1 to 5", Entry = 12345, ContinentId = wManager.Wow.Enums.ContinentId.Azeroth, Faction = wManager.Wow.Class.Npc.FactionType.Neutral, Position = new robotManager.Helpful.Vector3(1, 2, 3), CanFlyTo = true }), new Tuple<int, int, wManager.Wow.Class.Npc>(6, 9, new wManager.Wow.Class.Npc { Type = wManager.Wow.Class.Npc.NpcType.Repair, Name = "Npc name for level 6 to 9", Entry = 6789, ContinentId = wManager.Wow.Enums.ContinentId.Azeroth, Faction = wManager.Wow.Class.Npc.FactionType.Neutral, Position = new robotManager.Helpful.Vector3(6, 7, 8), CanFlyTo = true }), // ... }; // do: uint lastLevelCheck = 0; robotManager.Events.FiniteStateMachineEvents.OnBeforeCheckIfNeedToRunState += (engine, state, cancelable) => { var level = wManager.Wow.ObjectManager.ObjectManager.Me.Level; if (state != null && state.DisplayName == "To Town" && lastLevelCheck != level) { robotManager.Helpful.Logging.WriteDebug("Check NPC for current level"); wManager.Wow.Helpers.NpcDB.AcceptOnlyProfileNpc = true; wManager.Wow.Helpers.NpcDB.ListNpc.RemoveAll(n => n.CurrentProfileNpc); foreach (var npc in npcs) { if (npc.Item1 >= level && npc.Item2 <= level) { wManager.Wow.Helpers.NpcDB.AddNpc(npc.Item3, false, true); robotManager.Helpful.Logging.WriteDebug("Add npc: " + npc.Item3); } } lastLevelCheck = level; } }; } public void Dispose() { } public void Settings() { } } (not tested, tell me if this don't works)
In this sample, wrobot will refresh npc list at all level (and add npcs by level), but you can add condition to check current charater zone (by position disdance), ...
You can also run this code in quester step (put only content of method "Initialize()")
-
Matenia got a reaction from y2krazy in Sell items before buy food/drink
@Droidz this could be done by just changing priorities in the ToTown state.
Make it SELL first, then purchase food/drink.
@maukor in general bot settings, just disable "automatically add new NPCs to database".
If your bot only uses NPCs from profile AND doesn't add any new NPCs, you're good to go. But you will also have to add your own mailboxes and such.
@Droidz
If NpcDB.AcceptOnlyProfileNpc = true; doesn't work, it's a bug imo. This could be only for vanilla, I haven't really had problems in TBC.
-
Matenia got a reaction from Marsbar in Switch target, polymorph, switch to original target
Pretty much this. Plus, since the bot runs on another thread, sometimes it switches your target back BEFORE your poly cast even starts. Then you end up polying 2 targets back and forth and looking like a retard. Basically why Jasabi could never get polymorph to work correctly, I believe.
cancelable.Cancel = true is correct. However, I recommend NOT breaking the OnFightLoop event handler UNTIL your target has been successfully polymorphed. You can solve this with a while loop (Thread.Sleep inside) until poly is on another target.
-
Matenia got a reaction from Seminko in [SOLVED] Move after Frost Nova - is it really that hard?
You actually need to set isTooClose to false again after the while loop and maybe give it a short Thread.Sleep(500) or so at the end of each iteration.
Does the bot interrupt movement and then starts strafing again? Or is the strafing itself just choppy?
I highly recommend getting Visual Studio and doing a basic C# tutorial (or even Java, they're essentially the same
-
Matenia reacted to Droidz in Use hearthstone when bags are full
Hello, use plugin like HearthstoneToGoToTown.cs (not tested):
using System; using System.ComponentModel; using System.Threading; using System.Windows.Forms; using robotManager.Events; using robotManager.FiniteStateMachine; using robotManager.Helpful; using wManager.Wow.Helpers; using wManager.Wow.ObjectManager; public class Main : wManager.Plugin.IPlugin { public void Initialize() { FiniteStateMachineEvents.OnRunState += FiniteStateMachineEventsOnOnRunState; Logging.Write("[HearthstoneToGoToTown] Loadded."); } public void Dispose() { Logging.Write("[HearthstoneToGoToTown] Disposed."); } public void Settings() { MessageBox.Show("[HearthstoneToGoToTown] No settings for this plugin."); } private void FiniteStateMachineEventsOnOnRunState(Engine engine, State state, CancelEventArgs cancelable) { try { if (string.IsNullOrWhiteSpace(state.DisplayName) || state.DisplayName != "To Town") return; Logging.WriteDebug("[HearthstoneToGoToTown] Use Hearthstone."); var o = wManager.wManagerSetting.CurrentSetting.CloseIfPlayerTeleported; wManager.wManagerSetting.CurrentSetting.CloseIfPlayerTeleported = false; MovementManager.StopMove(); Thread.Sleep(Usefuls.Latency + 150); Lua.LuaDoString("local itemName, _, _, _, _, _, _, _ = GetItemInfo(6948); RunMacroText('/use ' .. itemName);"); Thread.Sleep(Usefuls.Latency + 500); if (ObjectManager.Me.IsCast) { Usefuls.WaitIsCasting(); Thread.Sleep(Usefuls.Latency + 10000); // wait load screen } wManager.wManagerSetting.CurrentSetting.CloseIfPlayerTeleported = o; } catch (Exception e) { Logging.WriteError("[HearthstoneToGoToTown] " + e); } } }
-
Matenia got a reaction from ScripterQQ in Huge banwave of bots 3.3.5
This happened on Outland every day since day 1. They ban at least 50 accounts a day. It means nothing, because the bot isn't detected.
It's just manual reports adding up. If their GMs actually put in any real work, they'd be banning 200 accounts a day. I've been botting on Warmane since day 1, got banned twice after never really logging out and staying in one spot for days.
-
Matenia got a reaction from nudl in Huge banwave of bots 3.3.5
This happened on Outland every day since day 1. They ban at least 50 accounts a day. It means nothing, because the bot isn't detected.
It's just manual reports adding up. If their GMs actually put in any real work, they'd be banning 200 accounts a day. I've been botting on Warmane since day 1, got banned twice after never really logging out and staying in one spot for days.
-
Matenia got a reaction from BetterSister in Huge banwave of bots 3.3.5
This happened on Outland every day since day 1. They ban at least 50 accounts a day. It means nothing, because the bot isn't detected.
It's just manual reports adding up. If their GMs actually put in any real work, they'd be banning 200 accounts a day. I've been botting on Warmane since day 1, got banned twice after never really logging out and staying in one spot for days.
-
Matenia got a reaction from Seminko in Making Range a variable
You have it correct.
Now if you have a bool for your fightclass like this
private bool _IsLaunched = false; private bool ChangeRange = false; private static WoWLocalPlayer Me = ObjectManager.Me; public float Range { get { if(ChangeRange) { return 29f; } return 5f; } } private void Pulse() { while(_IsLaunched) { //check if player not in combat yet, but bot wants to fight its current target //then set ChangeRange to true if target is further away than 12 yards, otherwise false ChangeRange = !Me.InCombat && Fight.InFight && ObjectManager.Target.GetDistance >= 12; Thread.Sleep(100); } } Then you need to call Pulse once in your Initialize method (in your fightclass).
Pulse should basically be executing your rotation. You can take a look at existing fightclasses like Eeny's to get a better idea at how they work.
Anyway, that should change your range accordingly. It's possible, that the bot won't react to this right away. If that's the case, you basically have to intercept events and change the range before they fire.
-
Matenia got a reaction from Seminko in Warlock keeping multiple targets dotted
public static bool CastSpell(RotationSpell spell, WoWUnit unit, bool force) { // targetfinder function already checks that they are in LoS if (unit != null && spell.IsKnown() && spell.CanCast() && unit.IsValid && !unit.IsDead) { if (wManager.wManagerSetting.CurrentSetting.IgnoreFightGoundMount && ObjectManager.Me.IsMounted) return false; Lua.LuaDoString("if IsMounted() then Dismount() end"); if (ObjectManager.Me.GetMove && spell.Spell.CastTime > 0) MovementManager.StopMoveTo(false, 500); if (ObjectManager.Me.IsCast && !force) return false; if (force) Lua.LuaDoString("SpellStopCasting();"); if (AreaSpells.Contains(spell.Spell.Name)) { /*spell.Launch(true, true, false); Thread.Sleep(Usefuls.Latency + 50); ClickOnTerrain.Pulse(unit.Position);*/ SpellManager.CastSpellByNameOn(spell.FullName(), GetLuaId(unit)); //SpellManager.CastSpellByIDAndPosition(spell.Spell.Id, unit.Position); ClickOnTerrain.Pulse(unit.Position); } else { if (unit.Guid != ObjectManager.Me.Guid) { MovementManager.Face(unit); } SpellManager.CastSpellByNameOn(spell.FullName(), GetLuaId(unit)); //Interact.InteractObject also works and can be used to target another unit } return true; } return false; } This is the function I use to cast spells and make sure my bot stops walking, if required.
Here, spell.IsKnown() and spell.CanCast() are shorthand functions on my spell class to make sure that the spell is not on cooldown and IsSpellUsable is true.
AreaSpells is just a list of strings containing spell names like Blizzard, Rain of Fire and other targeting AoE spells.
Then when deciding whether to use buffs (or like combat spells on an enemy), I just check as follows:
if(Fight.InFight || ObjectManager.Me.InCombat) { //Rotation here, for example CastSpell("Corruption", ObjectManager.Target) }else { //use buffs }
-
Matenia reacted to reapler in Radar3D How to use it?
Hello, you can subscribe your method to the event on initialize and unsubscribe on dispose:
public void Initialize() { Radar3D.Pulse(); Radar3D.OnDrawEvent += Radar3DOnDrawEvent; } public void Dispose() { Radar3D.OnDrawEvent -= Radar3DOnDrawEvent; Radar3D.Stop(); } private static void Radar3DOnDrawEvent() { if (ObjectManager.Target.IsValid) Radar3D.DrawLine(ObjectManager.Me.Position, ObjectManager.Target.Position, Color.Chocolate); Radar3D.DrawCircle(ObjectManager.Me.Position, 5, Color.Chocolate); }
-
Matenia reacted to reapler in How to get angle or rotation info?
This should finally do the desired work while the other method was just for calculating :)
/// <summary> /// Used to get the facing from two positions based on the origin rotation. /// </summary> /// <param name="from">The 1. position.</param> /// <param name="to">The 2. position.</param> /// <param name="originRotation">The origin rotation.</param> /// <returns>Negative radian on the right; positive on the left.</returns> public float FacingCenter(Vector3 from, Vector3 to, float originRotation) { var face = NormalizeRadian(Atan2Rotation(from, to) - originRotation); if (face < System.Math.PI) return -face; return NormalizeRadian(originRotation-Atan2Rotation(from, to)); } /// <summary> /// Used to normalize the input radian. /// </summary> /// <param name="radian">The radians to normalize.</param> public float NormalizeRadian(float radian) { if (radian < 0.0) return (float) (-(- radian % (2.0 * System.Math.PI)) + 2.0 * System.Math.PI); return radian % 6.283185f; } /// <summary> /// Used to calculate atan2 of to positions. /// </summary> /// <param name="from">The 1. position.</param> /// <param name="to">The 2. position.</param> /// <param name="addRadian">Radians to add.</param> /// <returns></returns> public float Atan2Rotation(Vector3 from, Vector3 to, float addRadian = 0) { return (float) System.Math.Atan2(to.Y - from.Y, to.X - from.X) + addRadian; }
Usage:
var rot = FacingCenter(ObjectManager.Me.Position, ObjectManager.Target.Position, ObjectManager.Me.Rotation); if (rot < 0) Logging.Write("Facing to right: "+rot); Logging.Write("Facing to left: "+rot);
-
Matenia reacted to reapler in How to get angle or rotation info?
Hello, this should work:
private static double ActualRotation(double value, double min, double max) { if (value < min) return min; return value > max ? max : value; } public static double CalculateFacingCenter(Vector3 source, float playerRotation, Vector3 destination) { Vector3 v2 = destination - source; v2.Z = 0.0f; Vector3 v1 = new Vector3((float) Math.Cos(playerRotation), (float) Math.Sin(playerRotation), 0.0f); return Math.Acos(ActualRotation(Vector3.Dot(ref v1, ref v2) / (v2.Magnitude() * (double) v1.Magnitude()), -1.0, 1.0)); } And additional snippets which could help you:
/// <summary> /// Used to calculate new position by parameter. /// </summary> /// <param name="from">The position to calculate from.</param> /// <param name="rotation">The rotation of the object in radians.</param> /// <param name="radius">The radius to add.</param> /// <returns></returns> public Vector3 CalculatePosition(Vector3 from, float rotation, float radius) { return new Vector3(System.Math.Sin(rotation) * radius + from.X, System.Math.Cos(rotation) * radius + from.Y, from.Z); } /// <summary> /// Used to calculate atan2 of to positions. /// </summary> /// <param name="from">Position 1.</param> /// <param name="to">Position 2.</param> /// <param name="addRadian">Radians to add.</param> /// <returns></returns> public float Atan2Rotation(Vector3 from, Vector3 to, float addRadian = 0) { return (float) System.Math.Atan2(to.Y - from.Y, to.X - from.X) + addRadian; } /// <summary> /// Used to face to the desired position. /// </summary> /// <param name="to">The position to face.</param> /// <param name="addRadian">Radians to add from the position.</param> public static void Face(Vector3 to, float addRadian = 0) { wManager.Wow.Helpers.ClickToMove.CGPlayer_C__ClickToMove(0, 0, 0, wManager.Wow.ObjectManager.ObjectManager.Me.Guid, 2, (float)System.Math.Atan2(to.Y - wManager.Wow.ObjectManager.ObjectManager.Me.Position.Y, to.X - wManager.Wow.ObjectManager.ObjectManager.Me.Position.X)+addRadian); } Usage for second block(i guess first one should be understandable)
//turn 180° Face(ObjectManager.Target.Position, (float)System.Math.PI); //turn left Face(ObjectManager.Target.Position, 1.53589f);//88° = 1.53589 rad //turn right Face(ObjectManager.Target.Position, -1.53589f);//88° = 1.53589 rad
-
Matenia got a reaction from Apexx in Alot of questions about FightClass and API
Yeah, the error message is very clear. Count is a property, you cannot call it like a method (such as "Count(args...)").
if (ObjectManager.GetUnitAttackPlayer().Where(u => u.GetDistance <= 6).ToList().Count > 2) Should do the trick
-
Matenia reacted to Droidz in Bot is spinning at one place, going forward then back again
Hello,
to use elevator you can use this sample: https://wrobot.eu/forums/topic/2681-snippets-codes-for-quest-profiles/?do=findComment&comment=24442
In WRobot code, I use offmeshconnection for that like:
new PathFinder.OffMeshConnection(new List<Vector3> { new Vector3(1900.185, -4362.321, 43.23154), new Vector3(1901.796, -4370.77, 44.39288) {Action = "c#: Logging.WriteNavigator(\"[OffMeshConnection] Ogrimmar > Wait Elevator\"); while (Conditions.InGameAndConnectedAndProductStartedNotInPause) { var elevator = ObjectManager.GetWoWGameObjectByEntry(206609).OrderBy(o => o.GetDistance).FirstOrDefault(); if (elevator != null && elevator.IsValid && elevator.Position.Z <= 44) break; Thread.Sleep(10); }"}, new Vector3(1903.901, -4382.015, 105.6566) {Action = "c#: Logging.WriteNavigator(\"[OffMeshConnection] Ogrimmar > Wait to leave Elevator\"); while (Conditions.InGameAndConnectedAndProductStartedNotInPause) { var elevator = ObjectManager.GetWoWGameObjectByEntry(206609).OrderBy(o => o.GetDistance).FirstOrDefault(); if (elevator != null && elevator.IsValid && elevator.Position.Z >= 105) break; Thread.Sleep(10); }"}, }, (int)ContinentId.Kalimdor),
-
Matenia reacted to Mykoplazma in The WRobot rant (long)
Ahh well becuase you dont understand how the gathering bot works :) Everytime where node is in scan range ( you can set it in global settings ) the bot is breaking the standard route and searching routine is generating shortest way to the node. Sometimes between player and the node are trees rocks or other crap and well the pathfinding routine is 'blind' to that things sometimes? ) (well soooome times) and bot will try to get the node and stuck on that crap. The solution? Reduce searching range to 20 yards and fly over the ground 20 yards when recording. ( i have a tool to set the point exactly 20 yards above the ground and only when I wish the point is added ) I created a perfect path and the bot can fly around 8 hours without any crazy ideas and the water - yest the water just fly higher to prevent some crazy water digging :P And all above all - the bot is for ground mount not for flying mount and the fly mount's landing procedures and avoiding are well - in beta.
-
Matenia got a reaction from Asoter in 1 Plugin - 2 or more cs files
Compile it yourself in Visual Studio into 1 DLL and it'll work just fine.
-
Matenia got a reaction from ScripterQQ in Preventing an istant spell to be casted (UNIT_SPELLCAST_SENT)
Actionbuttons use the function "UseAction" as far as I'm aware.
Could try hooking that. I'm not entirely sure how buttons cast the spell internally, but I know it goes through that function at some point.
-
Matenia got a reaction from gramdeck in Npc vendoring
You can blacklist vendors (tools => blacklist), remove them from your profile (if that's the source).
You cannot set a main vendor, but you can set your profile to ONLY use vendors from your profile, not your Bot's database.
In a quester profile, it looks like this:
<QuestsSorted Action="RunCode" NameClass="wManager.Wow.Helpers.NpcDB.AcceptOnlyProfileNpc = true;" />
-
Matenia reacted to reapler in Heals Casting Twice With Timer
Hello, i've created a small example for this, everything else is explained in the comments:
using System; using System.Collections.Generic; using System.Threading; using wManager.Plugin; using wManager.Wow.Class; using wManager.Wow.Enums; using wManager.Wow.Helpers; using wManager.Wow.ObjectManager; using static robotManager.Helpful.Logging; public class Main : IPlugin { #region Variables private bool _isLaunched; private int _msDelay = 200; private DateTime _unlockTime = DateTime.Now; private readonly HashSet<string> _noGcdSpells = new HashSet<string> { "Counterspell", //... //http://wowwiki.wikia.com/wiki/Cooldown => Abilities noted for not affecting nor being affected by the global cooldown: }; #endregion #region Properties public bool GcdActive => DateTime.Now < _unlockTime; #endregion #region WRobot Interface public void Initialize() { var pGuid = ToWoWGuid(ObjectManager.Me.Guid); EventsLuaWithArgs.OnEventsLuaWithArgs += delegate(LuaEventsId id, List<string> args) { if ( id == LuaEventsId.COMBAT_LOG_EVENT_UNFILTERED && args[2] == pGuid && !_noGcdSpells.Contains(args[9]) && ( args[1] == "SPELL_CAST_SUCCESS" || args[1] == "SPELL_HEAL" || args[1] == "SPELL_DAMAGE" ) ) { Write("lock"); _unlockTime = DateTime.Now.AddMilliseconds(_msDelay); } }; _isLaunched = true; Write("Loaded"); while (_isLaunched) { try { if (Conditions.ProductIsStartedNotInPause) { Pulse(); } } catch (Exception e) { WriteError(e.ToString()); } Thread.Sleep(30); } } public void Dispose() { _isLaunched = false; } public void Settings() { } #endregion #region Pulse public void Pulse() { var spell = new Spell("Lesser Heal"); if (!GcdActive && spell.IsSpellUsable && !ObjectManager.Me.IsCast && ObjectManager.Me.TargetObject.HealthPercent <= 90 ) { spell.Launch(false, false); //will cast the heal if "_msDelay" was passed } /* if (spell.IsSpellUsable && !ObjectManager.Me.IsCast && ObjectManager.Me.TargetObject.HealthPercent <= 90) { spell.Launch(false, false); //.IsSpellUsable is true after gcd was passed but the heal itself can delay //and cause double heal cast if no additional delay is added like above //so ObjectManager.Me.TargetObject.HealthPercent <= 90 would be true for a short time }*/ } #endregion #region Methods public string ToWoWGuid(ulong guid) { var wowGuid = ObjectManager.Me.Guid.ToString("x").ToUpper(); var c = 16 - wowGuid.Length; for (var i = 0; i < c; i++) { wowGuid = "0" + wowGuid; } return "0x" + wowGuid; } #endregion } if you are going to lower the tickspeed this will rarer happens, but it can still happen. Instant spells aren't included.