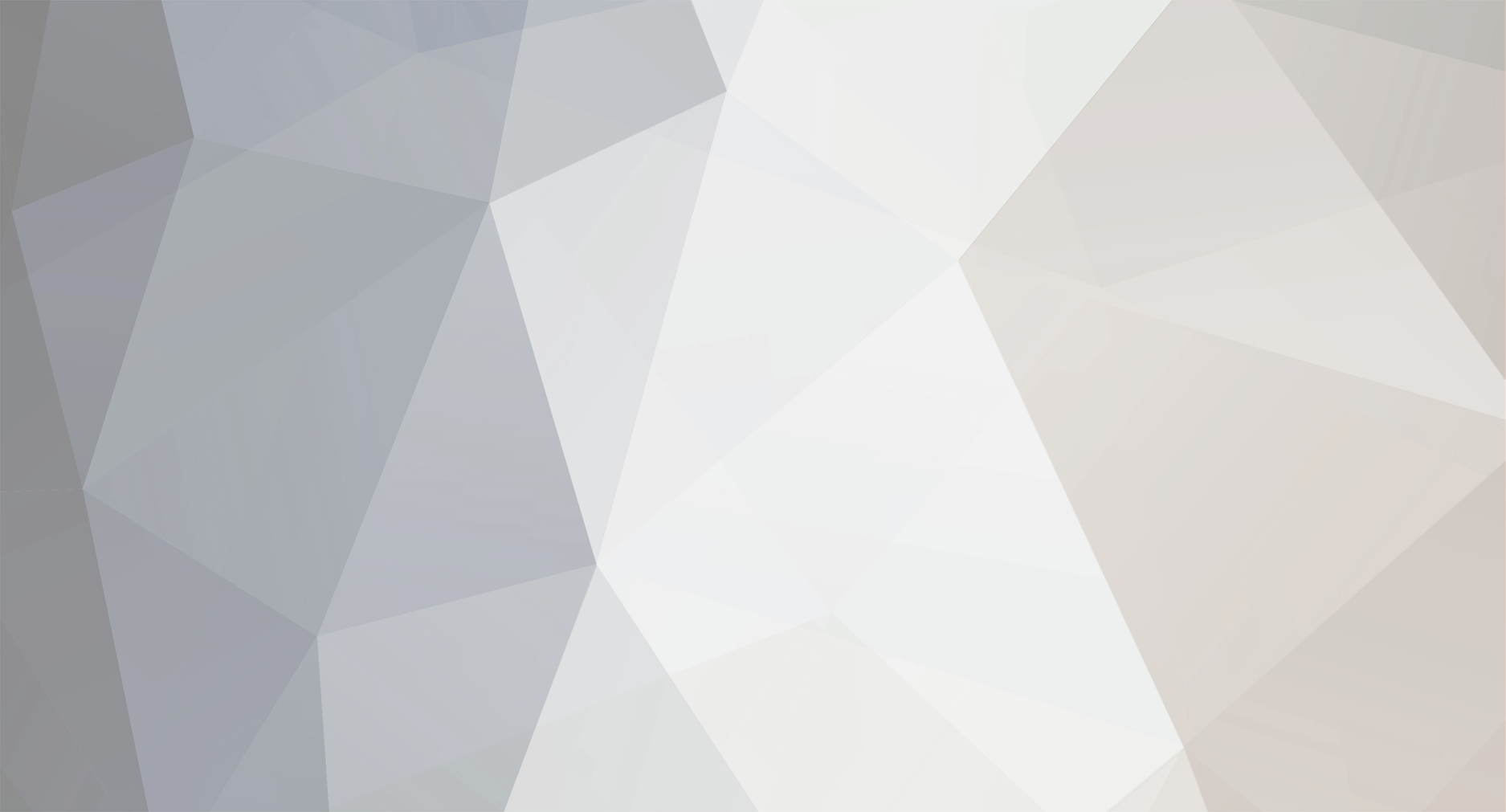
Matenia
-
Posts
2226 -
Joined
-
Last visited
Reputation Activity
-
Matenia got a reaction from Bambo in Thoradins Wall...
New wRobot update should be able to take offmesh even if you can make a path - so you can give it a try.
Otherwise ask FNV for the little piece of code he used to and from Searing Gorge. Basically it intercepts movements and enters your own.
Or do something similar to how I automate the Darnassus portal in HMP:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Drawing; using System.Linq; using System.Threading; using System.Threading.Tasks; using robotManager.Events; using robotManager.FiniteStateMachine; using robotManager.Helpful; using robotManager.Products; using wManager; using wManager.Events; using wManager.Wow.Bot.States; using wManager.Wow.Bot.Tasks; using wManager.Wow.Forms; using wManager.Wow.Helpers; using wManager.Wow.ObjectManager; public class Darnassus { public static bool TakingPortal; private static WoWLocalPlayer Me = ObjectManager.Me; private static Vector3 _currentDestination = Vector3.Empty; private static List<Vector3> _darnassusPolygon = new List<Vector3> { new Vector3(10749.604f, 2412.323f, 0f), new Vector3(11296.502f, 2097.9175f, 0f), new Vector3(11259.335f, 936.64453f, 0f), new Vector3(10914.204f, 317.97504f, 0f), new Vector3(9990.316f, 104.99061f, 0f), new Vector3(9236.339f, 480.24902f, 0f), new Vector3(8944.306f, 1230.7659f, 0f), new Vector3(9045.189f, 2295.6887f, 0f), new Vector3(9512.443f, 2792.6523f, 0f), new Vector3(10292.969f, 2904.216f, 0f), new Vector3(10569.073f, 2655.7341f, 0f), new Vector3(10946.0625f, 2371.7546f, 0f), }; public static void Start() { MovementEvents.OnMovementPulse += MovementEventsOnOnMovementPulse; } public static void Stop() { TakingPortal = false; _currentDestination = Vector3.Empty; MovementEvents.OnMovementPulse -= MovementEventsOnOnMovementPulse; } private static void MovementEventsOnOnMovementPulse(List<Vector3> points, CancelEventArgs cancelable) { //cancel all movement events that aren't our own /*if (_takingPortal && _currentDestination != Vector3.Empty && points.Last() != _currentDestination && !Logging.Status.ToLower().Contains("taxi")) { cancelable.Cancel = true; }*/ if (!TakingPortal && points.Count > 0) { bool taken = TakePortalIfNecessary(points.Last()); if (taken) { //GoToTask.ToPosition(points.Last()); cancelable.Cancel = true; } } } private static bool TakePortalIfNecessary(Vector3 destination) { bool taken = false; if (Usefuls.ContinentId == 1) { if (VectorHelper.PointInPolygon2D(_darnassusPolygon, Me.Position) && !VectorHelper.PointInPolygon2D(_darnassusPolygon, destination)) { PluginLog.Log("Player in Darnassus, leaving through portal"); GoThroughPortal(new Vector3(9947.029, 2618.881, 1316.962), new Vector3(9946.723, 2618.692, 1316.909)); taken = true; } else if (!VectorHelper.PointInPolygon2D(_darnassusPolygon, Me.Position) && VectorHelper.PointInPolygon2D(_darnassusPolygon, destination)) { PluginLog.Log("Player outside Darnassus, entering through portal"); GoThroughPortal(new Vector3(8786.36, 967.445, 30.197), new Vector3(8788.761, 967.9706, 30.19558)); taken = true; } } TakingPortal = false; _currentDestination = Vector3.Empty; return taken; } private static void GoThroughPortal(Vector3 destination, Vector3 direction) { TakingPortal = true; _currentDestination = destination; var tmp = wManagerSetting.CurrentSetting.CloseIfPlayerTeleported; wManagerSetting.CurrentSetting.CloseIfPlayerTeleported = false; GoToTask.ToPosition(destination); //For some reason, GoToTask ends right away if (Me.IsDead && Me.HaveBuff("Ghost")) { MovementManager.Go(PathFinder.FindPath(destination)); while (Conditions.InGameAndConnectedAndAliveAndProductStartedNotInPause && Me.Position.DistanceTo(destination) >= 2) { Thread.Sleep(500); } } MovementManager.MoveTo(direction); Thread.Sleep(500); Move.Forward(Move.MoveAction.PressKey, 5000); //Reenable(tmp); } private static async void Reenable(bool close) { await Task.Run(() => { Products.InPause = true; Thread.Sleep(5000); MovementManager.StopMove(); MovementManager.CurrentPath.Clear(); MovementManager.CurrentPathOrigine.Clear(); wManagerSetting.CurrentSetting.CloseIfPlayerTeleported = close; Products.InPause = false; TakingPortal = false; //Products.ProductRestart(); PluginLog.Log("Resetting path after port"); }); } }
-
Matenia reacted to Droidz in Warmane Lordaeron Bans
Hello,
If you can try with new update and tell me if problem is resolved (be careful)
-
Matenia got a reaction from Grevlen in Part Combat, not switching from combat target to heal party
You can't create party fightclasses with the XML editor afaik. But also, if this is about vanilla - you need to cancel cat form before casting anything else
-
Matenia reacted to Droidz in [TAURI] Wrobot detected
I found how they detect WRobot I'll fix it in next update
-
Matenia reacted to fantasia in Issues with combat after ressing when using C# fight class
It was a different but similar situation.
I used some code that someone else made that would pause the bot for a while if it was a pvp death. But it was written wrong so it only paused the fight class not the entire bot and it triggered if the enemy wasn't same faction as the bot (which caused it to trigger on mobs since they are not same faction...).
I should have checked their code better, somehow it sounded like it was good in my head when I read it but ofcourse it isn't.
-
Matenia reacted to FNV316 in No navigation In Silithus ( cenarion hold )
Took an unskilled programmer like me around four hours to fully automate entering Thorium Point, reach any NPC at Thorium Point and leaving Thorium Point, without my bot breaking his nose every other minute. Might be a dirty solution, but it works. Why exactly is this still a thing?
Thorium Point - Default Path Finder VS Custom Path Plugin.mp4 -
Matenia got a reaction from ScripterQQ in Help with plugin working 50% (easy)
wManager.Wow.Bot.Tasks.GoToTask.ToPosition(new Vector3(-5814.899, 650.6406, 94.55161));
This code is blocking - but your loop should have a Thread.Sleep anyway to keep your CPU from exploding.
I'm guessing SOMETHING interrupts your code - so add some logging to see if the above quoted code actually returns anything. It probably breaks early, so maybe try an exitCondition for the function call that's basically just if you're getting attacked.
Or do MovementManager.Go(PathFinder.FindPath(vector3)), that will never terminate unless your movement is otherwise interruped (say by fightclass, plugin, etc). On the downside, you need to add a sleep until it reaches the final destination, because it's not blocking.
Edit:
You could also calculate the path ONCE and then just walk it in your loop. Pseudo code below, I don't have a PC right now, just doing this from the top of my head.
using System; using robotManager.Products; using wManager.Plugin; using wManager.Wow.Enums; using robotManager.Helpful; using System.Threading; using wManager.Wow; using wManager.Wow.Helpers; using wManager.Wow.ObjectManager; public class Main : wManager.Plugin.IPlugin { const int HONORREQUIRED = 10000; bool _running; List<Vector3> pathToFollow = new List<Vector3>(); public void Initialize() { _running = true; while (_running) { if (Conditions.InGameAndConnectedAndAliveAndProductStartedNotInPause && !Conditions.IsAttackedAndCannotIgnore && !Battleground.IsInBattleground()) { if(!pathToFollow.IsEmpty() && ObjectManager.Me.Position.DistanceTo(pathToFollow[pathToFollow.Count - 1]) > 5) { MovementManager.Go(pathToFollow); //wait until we reach last point or enter combat while(ObjectManager.Me.Position.DistanceTo(pathToFollow[pathToFollow.Count - 1]) > 5) { Thread.Sleep(500); if(Conditions.IsAttackedAndCannotIgnore || !Conditions.InGameAndConnectedAndAliveAndProductStartedNotInPause) { break; } } } var zone = Lua.LuaDoString<string>("return GetZoneText()"); var h = Lua.LuaDoString<int>("local points = GetHonorCurrency(); return points;"); if (h >= HONORREQUIRED && zone == "Stormwind City") { pathToFollow = PathFinder.FindPath(new Vector3(-5768.91, 419.931, 103.921)); wManager.Wow.Bot.Tasks.GoToTask.ToPositionAndIntecractWithNpc(new Vector3(-5768.91, 419.931, 103.921), 34081); Lua.LuaDoString<int>("BuyMerchantItem(9,1)"); } // Wait Position if (h < HONORREQUIRED && zone == "Stormwind City") { pathToFollow = PathFinder.FindPath(new Vector3(-5814.899, 650.6406, 94.55161)); wManager.Wow.Bot.Tasks.GoToTask.ToPosition(new Vector3(-5814.899, 650.6406, 94.55161)); } } Thread.Sleep(500); } } public void Dispose() { _running = false; } public void Settings() { } }
-
Matenia got a reaction from zzzar in Custom Script in quester profile
http://wowwiki.wikia.com/wiki/API_IsResting?oldid=134114
Lua.LuaDoString("return IsResting()");
-
Matenia got a reaction from Marsbar in Party does not attack enemy (PLAYERS)
Party product is not being developed anymore. It's not even part of the advertised features. He threw it in there for good measure because he developde waaaaaay back in the day.
I already released a free plugin for in-party leveling here:
If you don't like some of the code in it, you can just modify it since the source is attached.
I personally think Droidz should just remove the Party product and a few others so people stop acting entitled to it.
-
Matenia got a reaction from artur.k in Thoughts so far
You're not supposed to use free profiles. You're supposed to make your own (like absolutely everyone who knows anything about botting does), because sharing with people creates bot trains and will get you banned.
In 2016/2017 there were basically no paid fight classes at all. People still botted just fine using the fightclass editor - which I agree could use some work (because it was created for MoP and backported to other expansions later).
HumanMasterPlugin came out by the end of 2017. It's not even 1 year old and only works for the first 3 expansions. I personally started using wRobot for MoP and was fine using grinders (in Quester product) where food was set for a level range of ~5, using wRobot's normal food buying by just adding only 1 vendor and repair to the profile and limiting wRobot to them. That's how I came up with the free food and drink plugin (I essentially used that code in quester).
Obviously I think my plugin is useful and so are my fightclasses. But these are things most private grinders had beforehand anyway - it's just stuff that helps with laziness.
If you've ever botted outside of wRobot you know that the framework it provides is already pretty damn good. You will never see another HonorBuddy (it was an actual company with paid developers and they paid their community devs because they appealed to the masses) and if you compare wRobot to Glider or Pirox, it's doing damn well.
For most people here botting is a business. Which makes it a competitive scene. It's actually (considering the community's small size) a miracle people are still this nice and are sharing that much stuff and code for free.
-
Matenia got a reaction from Findeh in Thoughts so far
You're not supposed to use free profiles. You're supposed to make your own (like absolutely everyone who knows anything about botting does), because sharing with people creates bot trains and will get you banned.
In 2016/2017 there were basically no paid fight classes at all. People still botted just fine using the fightclass editor - which I agree could use some work (because it was created for MoP and backported to other expansions later).
HumanMasterPlugin came out by the end of 2017. It's not even 1 year old and only works for the first 3 expansions. I personally started using wRobot for MoP and was fine using grinders (in Quester product) where food was set for a level range of ~5, using wRobot's normal food buying by just adding only 1 vendor and repair to the profile and limiting wRobot to them. That's how I came up with the free food and drink plugin (I essentially used that code in quester).
Obviously I think my plugin is useful and so are my fightclasses. But these are things most private grinders had beforehand anyway - it's just stuff that helps with laziness.
If you've ever botted outside of wRobot you know that the framework it provides is already pretty damn good. You will never see another HonorBuddy (it was an actual company with paid developers and they paid their community devs because they appealed to the masses) and if you compare wRobot to Glider or Pirox, it's doing damn well.
For most people here botting is a business. Which makes it a competitive scene. It's actually (considering the community's small size) a miracle people are still this nice and are sharing that much stuff and code for free.
-
Matenia reacted to Droidz in Character nickname in title of wow window
Hello,
Use this plugin: Change Wow Window Title.cs
using System; using System.Runtime.InteropServices; public class Main : wManager.Plugin.IPlugin { [DllImport("user32.dll", SetLastError = true, CharSet = CharSet.Auto)] public static extern bool SetWindowText(IntPtr hwnd, String lpString); public void Initialize() { try { SetWindowText(wManager.Wow.Memory.WowMemory.Memory.WindowHandle, wManager.Wow.ObjectManager.ObjectManager.Me.Name); } catch {} } public void Dispose() { } public void Settings() { } }
-
Matenia reacted to Droidz in Help with quester profile
try
string stepName = "MyStepName"; var p = wManager.Wow.Helpers.Quest.QuesterCurrentContext.Profile as Quester.Profile.QuesterProfile; if (p != null) { for (int i = 0; i < p.QuestsSorted.Count; i++) { if (p.QuestsSorted[i].Action == wManager.Wow.Class.QuestAction.StepName && p.QuestsSorted[i].NameClass == stepName) { wManager.Wow.Helpers.Quest.QuesterCurrentContext.CurrentStep = i; break; } } }
-
Matenia got a reaction from BetterSister in Hunter issue
If you have problems facing the target, look at the 1000s of topics regarding this issue where peopel don't run on 60 Hz with vsync enabled
-
Matenia got a reaction from Findeh in Northdale Bans?
I think @Findeh's idea of them flooding ports on your LAN somehow before banning you seems the most reasonable. This would definitely an exploit in Warden though - it's absolutely not possible the way Blizzard (intentionally) engineered it.
That would explain why some people haven't gotten hit at all (luck + different network architecture) whereas people runnign several VMs are also safe.
It would just be so much better if you could spawn network adapters and route all traffic through them + still force WoW through a proxy. Maybe if someone took up mass-botting on a linux machine again this could work.
-
Matenia reacted to Findeh in Northdale Bans?
There will be a lot of text. Sorry in advance for my english, hope it will be readable without bleeding from both eyes.
Different clients don't work. I do that. Got 20 copies of wow. And i do delete them all after each ban and create new one. They are targeting lan, no doubt there.
The guy that runs "many" bots is clearly running like 3-4 per PC, or even less. Maybe even with VM, that's my guess to calm you )
Current solution - many network adapters or many vpn. It also seems that they are flooding the port from time to time. Maybe that's their check. My bots do disconnect atleast couple times befor ban, sometimes they can not even reach Wrobot server (according to the logs), so they clearly experiencing some short-time internet trouble. At the same time, bots at another PC with the same internet don't even disconect. So it's not internet globally, it's an only one adapter / pc problem.
P.S. Also, if there are really guys, who is running 10+ bots per pc with 1 network adapter, 20+ hours per day, without VM, and they are not affected by mass bans, i'd assume that they have some kind of inbuild proxy or NAT within their provider network, that is, somehow, protects them by not leaking their real local ip. For example, if you will have adsl connection, it's mostly using vpn during each connect. Lets asume they gather statistic "how many connects from local ip x.y.z.n were done". If you are running 10 bots with the same local ip, you will hit that filter very fast. If you are running like 3, maybe you will not hit it. And if you change your local IP often (like every 12 hours atleas, because you are running adsl vpn), maybe you will not hit that filter as well. So you may think you are superior, but in fact, it's just a coincidence or your provider network architecture.
It's just a theory, i'm not an network engineer or something, have no idea what i'm talking about )
-
Matenia got a reaction from vanbotter in PVP plugins - track enemy players for example
foreach (WoWUnit Mob in ObjectManager.GetWoWUnitAttackables().Where(x => x.Type == WoWObjectType.Player && x.GetDistance2D < AggroMonitorSettings.CurrentSetting.SearchRange && x.TargetObject.Name != AggroMonitorSettings.CurrentSetting.Tank1 && x.TargetObject.Name != AggroMonitorSettings.CurrentSetting.Tank2 && x.TargetObject.Name != AggroMonitorSettings.CurrentSetting.Tank3 && x.TargetObject.Name != ObjectManager.Me.Name && ObjectManager.Target.TargetObject != null)) This filters it to just players, if you modify AggroMonitor
-
Matenia got a reaction from vanbotter in PVP plugins - track enemy players for example
ObjectManager.Me.Position
-
Matenia got a reaction from exuals in Walking into multiple mobs to target far away, pulls 3+
Gonna dump some code here I've used to experiment, you can adjust it for your own needs:
public class SmartPulls { private static WoWLocalPlayer Me = ObjectManager.Me; private static int _checkDistance = 22; private static int _frontDistance = 15; public static void Start() { if (PluginSettings.CurrentSetting.SmartPulls) { FightEvents.OnFightStart += FightValidityCheck; Radar3D.OnDrawEvent += Radar3DOnOnDrawEvent; } } private static void Radar3DOnOnDrawEvent() { if (Radar3D.IsLaunched) { Vector3 rightPlayerVector = Helper.CalculatePosition(Me.Position, -Helper.Atan2Rotation(Me.Position, Me.Position, Me.Rotation), -_checkDistance); Vector3 leftPlayerVector = Helper.CalculatePosition(Me.Position, -Helper.Atan2Rotation(Me.Position, Me.Position, Me.Rotation), _checkDistance); Vector3 rightTargetVector = Helper.CalculatePosition(rightPlayerVector, -Helper.Atan2Rotation(Me.Position, rightPlayerVector, 0), _checkDistance + _frontDistance); Vector3 leftTargetVector = Helper.CalculatePosition(leftPlayerVector, -Helper.Atan2Rotation(Me.Position, leftPlayerVector, 0), -(_checkDistance + _frontDistance)); Radar3D.DrawCircle(rightPlayerVector, 2.5f, Color.Blue); Radar3D.DrawCircle(leftPlayerVector, 2.5f, Color.Green); Radar3D.DrawCircle(rightTargetVector, 2.5f, Color.Yellow); Radar3D.DrawCircle(leftTargetVector, 2.5f, Color.DarkRed); Radar3D.DrawLine(rightPlayerVector, leftPlayerVector, Color.Gold); Radar3D.DrawLine(leftPlayerVector, leftTargetVector, Color.Gold); Radar3D.DrawLine(leftTargetVector, rightTargetVector, Color.Gold); Radar3D.DrawLine(rightTargetVector, rightPlayerVector, Color.Gold); } } public static void Stop() { FightEvents.OnFightStart -= FightValidityCheck; Radar3D.OnDrawEvent -= Radar3DOnOnDrawEvent; } public static void Pulse() { if (PluginSettings.CurrentSetting.SmartPulls && !Escape.IsEscaping && Me.Target > 0 && !Fight.InFight && !Me.InCombatFlagOnly && Logging.Status != "Regeneration" && !Me.HaveBuff("Resurrection Sickness")) { Vector3 rightPlayerVector = Helper.CalculatePosition(Me.Position, -Helper.Atan2Rotation(Me.Position, Me.Position, Me.Rotation), -_checkDistance); Vector3 leftPlayerVector = Helper.CalculatePosition(Me.Position, -Helper.Atan2Rotation(Me.Position, Me.Position, Me.Rotation), _checkDistance); Vector3 rightTargetVector = Helper.CalculatePosition(rightPlayerVector, -Helper.Atan2Rotation(Me.Position, rightPlayerVector, 0), _checkDistance + _frontDistance); Vector3 leftTargetVector = Helper.CalculatePosition(leftPlayerVector, -Helper.Atan2Rotation(Me.Position, leftPlayerVector, 0), -(_checkDistance + _frontDistance)); List<Vector3> boundBox = new List<Vector3> { rightPlayerVector, leftPlayerVector, rightTargetVector, leftTargetVector }; WoWUnit unit = ObjectManager.GetObjectWoWUnit() .Where(o => o.IsAlive && o.Reaction == Reaction.Hostile && !o.IsPet && !o.IsPlayer() && o.Guid != Me.Target && (o.Level >= Me.Level ? o.Level - Me.Level <= 3 : Me.Level - o.Level <= 6) && VectorHelper.PointInPolygon2D(boundBox, o.Position) && !TraceLine.TraceLineGo(Me.Position, o.Position, CGWorldFrameHitFlags.HitTestSpellLoS) && !wManagerSetting.IsBlackListed(o.Guid)) .OrderBy(o => o.GetDistance) .FirstOrDefault(); if (unit != null) { PluginLog.Log($"{unit.Name} is in our way, attacking!"); MovementManager.StopMoveTo(false, 500); ObjectManager.Me.Target = unit.Guid; Fight.StartFight(unit.Guid); ObjectManager.Me.Target = unit.Guid; } } } //don't pull another target if we are already in combat with anyone (e.g. body pulled mobs) private static void FightValidityCheck(WoWUnit unit, CancelEventArgs cancelable) { if (Escape.IsEscaping || ObjectManager.GetObjectWoWUnit().Count(u => u.IsTargetingMeOrMyPet && u.Reaction == Reaction.Hostile) > 0) { return; } if (CheckForGroup(unit, cancelable)) { MovementManager.StopMoveTo(false, 500); cancelable.Cancel = true; return; } if (CheckForClearPath(unit, cancelable)) { MovementManager.StopMoveTo(false, 500); cancelable.Cancel = true; return; } } private static bool CheckForGroup(WoWUnit unit, CancelEventArgs cancelable) { // group too large, nothing we can do, stop fight and go away int addAmount = ObjectManager.GetObjectWoWUnit().Count(o => o.IsAlive && o.Reaction == Reaction.Hostile && o.Guid != unit.Guid && o.Position.DistanceTo(unit.Position) <= 12 && !o.IsTargetingMeOrMyPetOrPartyMember); if (addAmount > 1) { wManagerSetting.AddBlackList(unit.Guid, 60000); Fight.StopFight(); Lua.LuaDoString("ClearTarget()"); wManagerSetting.AddBlackListZone(unit.Position, 30 + addAmount * 5); PathFinder.ReportBigDangerArea(unit.Position, 30 + addAmount * 5); PluginLog.Log("Stopping pull on large group!"); return true; } return false; } private static bool CheckForClearPath(WoWUnit unit, CancelEventArgs cancelable) { if (Me.InCombatFlagOnly) { return false; } Vector3 rightPlayerVector = Helper.CalculatePosition(Me.Position, -Helper.Atan2Rotation(unit.Position, Me.Position, 0), _checkDistance); Vector3 leftPlayerVector = Helper.CalculatePosition(Me.Position, -Helper.Atan2Rotation(unit.Position, Me.Position, 0), -_checkDistance); Vector3 rightTargetVector = Helper.CalculatePosition(unit.Position, -Helper.Atan2Rotation(Me.Position, unit.Position, 0), -_checkDistance); Vector3 leftTargetVector = Helper.CalculatePosition(unit.Position, -Helper.Atan2Rotation(Me.Position, unit.Position, 0), _checkDistance); List<Vector3> boundingBox = new List<Vector3>{rightPlayerVector, leftPlayerVector, rightTargetVector, leftTargetVector}; if (ObjectManager.GetObjectWoWUnit().FirstOrDefault(o => o.IsAlive && o.Reaction == Reaction.Hostile && o.Guid != unit.Guid && VectorHelper.PointInPolygon2D(boundingBox, o.Position)) != null) { WoWUnit newEnemy = ObjectManager.GetObjectWoWUnit() .Where(o => o.IsAlive && o.Reaction == Reaction.Hostile && o.SummonedBy <= 0 && !o.IsPlayer() && o.Guid != unit.Guid && !wManagerSetting.IsBlackListed(o.Guid) && !wManagerSetting.IsBlackListedNpcEntry(o.Entry)) .OrderBy(o => o.GetDistance) .FirstOrDefault(); if (newEnemy != null) { wManagerSetting.AddBlackList(unit.Guid, 60000); Fight.StopFight(); Fight.StartFight(newEnemy.Guid); PluginLog.Log($"Enemy in the way, can't pull here - pulling {newEnemy.Name} instead"); } return true; } return false; } }
-
Matenia got a reaction from Avvi in Avvi's C# Tips & Tricks with Helpful Code Snippets
Everything after run is regular Lua, so if you want to run your macro like that, just do
(Vanilla has no RunMacroText, so it would never work anyway - but more importantly, you should use an IDE to edit your code so you can see things like trying to put 4 quotation marks won't work, because your string ends at ( and then inbetween the next ")" it tries to execute Macro2 as code. See Strings.)
Lua.LuaDoString("RunMacro('Macro2')");
-
Matenia reacted to Droidz in Resurrecting bugged? (elysium)
Hello, I added setting to select distance to res:
wManager.wManagerSetting.CurrentSetting.RetrieveCorpseMaxDistance (by default 26)
-
Matenia got a reaction from vanbotter in Avvi's C# Tips & Tricks with Helpful Code Snippets
Everything after run is regular Lua, so if you want to run your macro like that, just do
(Vanilla has no RunMacroText, so it would never work anyway - but more importantly, you should use an IDE to edit your code so you can see things like trying to put 4 quotation marks won't work, because your string ends at ( and then inbetween the next ")" it tries to execute Macro2 as code. See Strings.)
Lua.LuaDoString("RunMacro('Macro2')");
-
Matenia reacted to Droidz in Repair/Install WRobot
Before request help, thank you to:
Make sure you start wow in 32-bit and run Wow in Windowed mode. Make sure do you use WRobot on administrator Windows session. Keep Windows updated. Try to disable your antivirus/firewall, redownload and reinstall WRobot in empty folder. Delete completely WRobot folder, download and install it again (you can try to download preinstalled version). Try to put WRobot folder on your desktop and in root of the disc. (Re)Install DirectX, Framework (minimum 4.5) (or Framework Repair Tool), SlimDX (4.0 X86), Redistributable Visual C + + 2010 (X86). If the bot does not work at all, that WRobot freeze, change the version of DirectX that wow uses (do not forget to restart Wow) (if this don't resolve problem, you can try to launch WRobot with shortcut "WRobot No DX"). Reset key bindings Wow (the bot uses Forward, Backward, Jump, Sit / Stand, strafe right and left, but I suggest you reset everything). Some wow addon can cause problems, so disable all. Close Windows program like Skype, Teamviewer and all overlays e.g. Overwolf, Geforce Experience, raptr, AMD Gaming Evolved, teamspeak (these programs can cause problems at WRobot, also programs for record screen or programs which draw/write in game window). Close/disable your VPN, Proxy and program like ProxyCap. If you have already run WRobot, you can try to remove folde "WRobot\Data\Meshes\". If WRobot window is not display correctly, you can you try to Right-click on WRobot.exe and then click "Properties". On the "Compatibility" tab, select "Disable Display Scaling On High DPI Settings", and then click "OK" ( https://www.youtube.com/watch?v=0xS-UCuyq7s ). Some virus/malwares can block WRobot. Scan you computer AdwCleaner and/or Malwarebytes for remove malwares, and anti-virus like Eset or Kaspesky. Disable "Bliz Streaming" feature, for it, on the Battle.net App, go to upper left corner, click the down arrow, go to Settings. From there, go to Streaming and uncheck Enable Streaming. When WRobot is launched, you cannot minimize Wow, of course you can keep Wow in background and use your computer, but you cannot minize (put in taskbar) Wow (if minimized, WRobot don't works correctly). Sometime, you need to put "full control" at "WRobot.exe" (and WRobot folder): https://www.windowscentral.com/how-take-ownership-files-and-folders-windows-10 Do a search to see if a solution exists: https://www.google.com/search?q=site:wrobot.eu+adding+mailbox or http://wrobot.eu/search/. If you start to use bot, you can watch this video: http://wrobot.eu/forums/topic/3633-getting-started-with-wrobot-video/ If your problem is not resolved, request help on good forum, to get quick reply, don't forget to share your log file.
-
Matenia got a reaction from Marsbar in Northdale Warden scanning for hardware?
As far as I know you can execute Lua code through warden in Vanilla. If this is possible they could do something like this:
- they collected a few proxy IPs to put on a blacklist
- everyone currently logged into those IPs is made to execute some Lua snippet
- it makes you join a channel but hides all messages that would indicate this from the UI
- sends a message to that channel (either from server or client itself)
- message could contain a hash of some sort that the server expects (maybe wRobot blocks this entirely and they don't receive the hash at all => must be bot)
- message could contain GetTime() (Lua function) which tells you EXACTLY how long your PC has been running in milliseconds (they assume no PCs connected to their server will ever return the same time)
I'm not sure how @Droidz blocks Lua unlock detection. If he prevents the server from executing FrameScipt_Execute entirely through Warden, it would mean wRobot is detected and they would probably ban a lot of people. So this is unlikely.
If the server is allowed to execute this, they can access your computer's uptime to have a very loose indicator that some clients might run on the same computer (but really out of 10000 players, what are the chances that 6 suspected botting accounts all have started their computer within 3-5 milliseconds of each other?).
If they use GetTime() to tell computer identity (I'm laughing internally at the combined genius and stupidity of this), this following line put into the chat after you log into the game (you cannot relog after this or reload the UI) would fix detection:
/run getTimeConstant = math.random(0, 10000000); _gt = GetTime; function GetTime() return _gt() + getTimeConstant end Keep in mind, that this can break many things, such as cooldown calculation for spells. Use with care.
-
Matenia got a reaction from ScripterQQ in Wrotation warmane
Yes it isn't detected and neither is EWT. Pretty sure people have even modified the old PQR by now to work around Warmane's detection (which was mostly string detection, looking for PQR in chatframe)